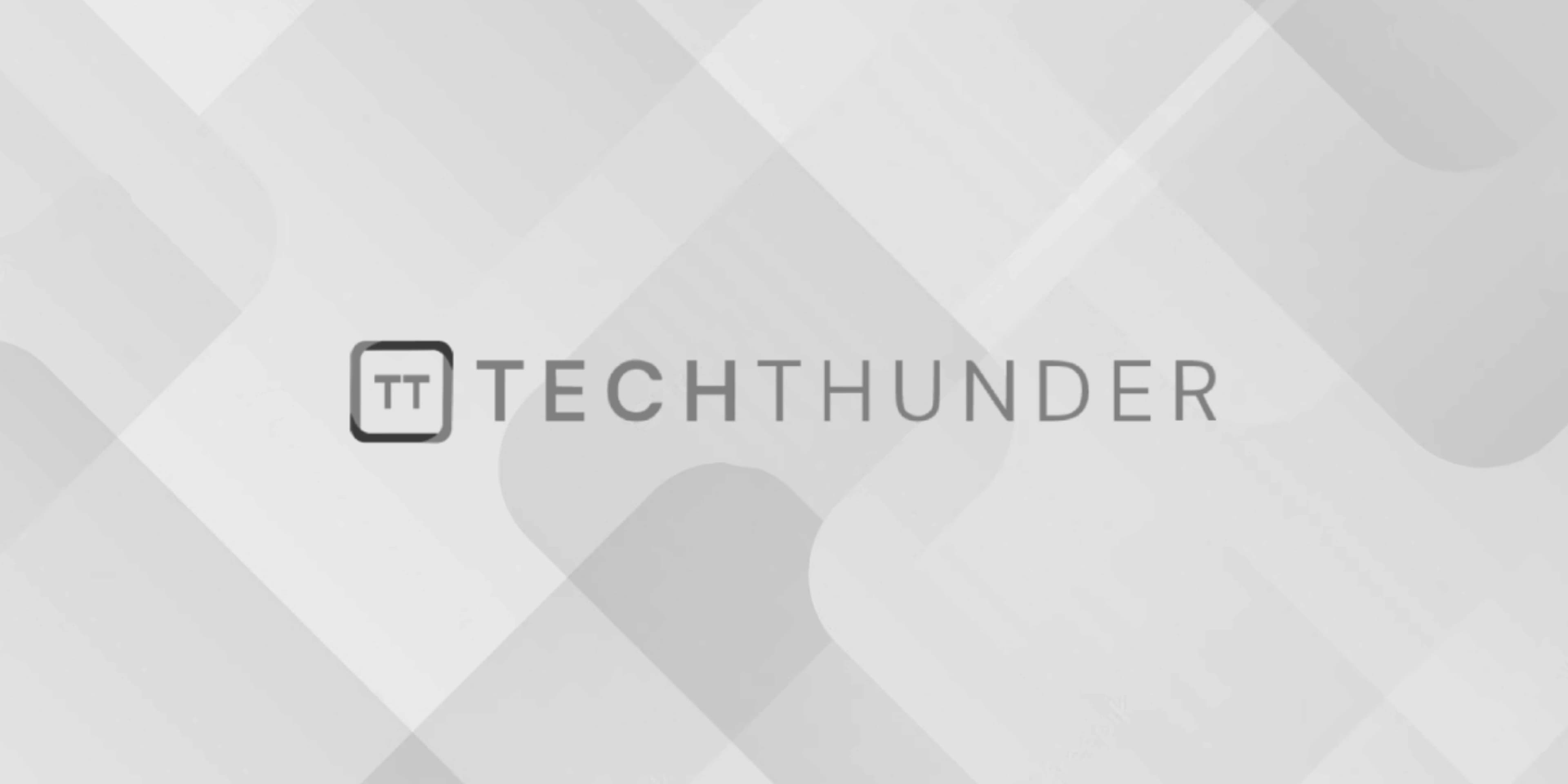
Implementing a GET service to retrieve all Posts of a User
To implement a GET service that retrieves all posts of a user in a Spring Boot application, you can follow these steps:
- Create a
Post
Repository:
If you haven’t already, create a repository for thePost
entity by extendingJpaRepository<Post, Long>
. - Create a Service:
Create a service class that will handle retrieving posts for a user. Inject thePostRepository
andUserRepository
as needed.
@Service
public class PostService {
@Autowired
private PostRepository postRepository;
@Autowired
private UserRepository userRepository;
public List<Post> getAllPostsByUserId(Long userId) {
Optional<User> user = userRepository.findById(userId);
if (user.isPresent()) {
return postRepository.findByUser(user.get());
} else {
throw new UserNotFoundException("User not found with ID: " + userId);
}
}
}
- Create a Controller:
Create a controller class to handle the REST endpoint for getting all posts of a user. Inject thePostService
.
@RestController
@RequestMapping("/users/{userId}/posts")
public class PostController {
@Autowired
private PostService postService;
@GetMapping
public List<Post> getAllPostsByUserId(@PathVariable Long userId) {
return postService.getAllPostsByUserId(userId);
}
}
- Use the Endpoint:
You can now access the endpoint using a URL like/users/{userId}/posts
to retrieve all posts of a specific user.
In this setup, the getAllPostsByUserId
method in the PostService
queries the database to find the user by their ID. If the user exists, it then retrieves all posts associated with that user using the findByUser
method provided by Spring Data JPA’s query derivation mechanism. If the user is not found, it throws a UserNotFoundException
.
The PostController
uses the PostService
to fetch posts for the requested user and returns them as a list in the response.
Remember to handle imports and error handling properly, and make sure that your database tables are properly set up with the relationships defined in the User
and Post
entities.