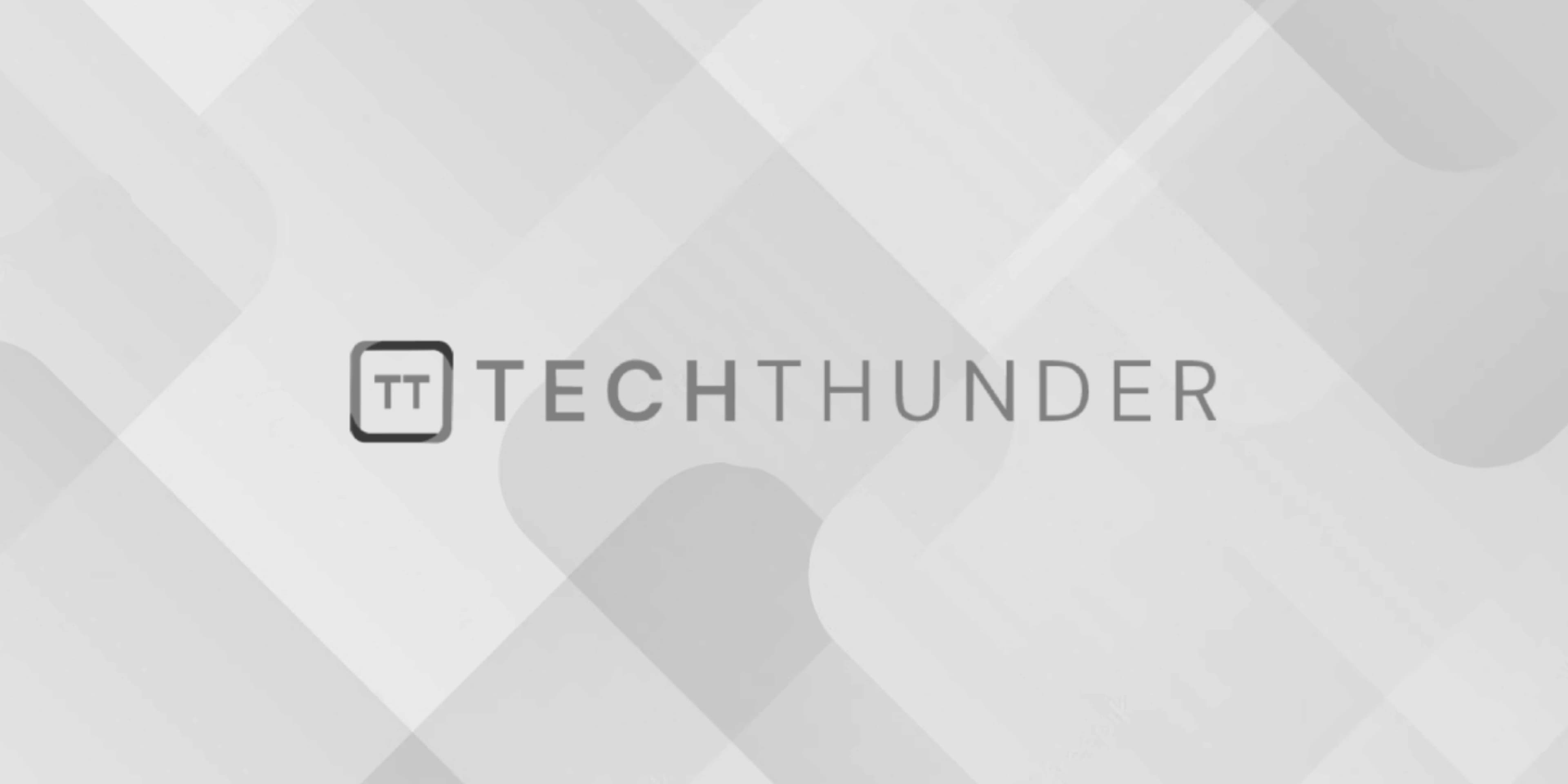
Enhancing the Hello World Service with a Path Variable
Enhancing a “Hello World” service in Spring Boot with a path variable allows you to create dynamic and parameterized endpoints. Path variables are placeholders within the URL that can capture values provided by clients, making your service more versatile. Here’s how to enhance a “Hello World” service with a path variable:
Step 1: Create or Update the Controller:
- Open or create a Spring Boot controller class that handles the “Hello World” service. If you don’t have one yet, you can create a new controller class. For this example, we’ll assume you have a controller named
HelloWorldController
.
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/hello")
public class HelloWorldController {
@GetMapping("/{name}")
public String sayHello(@PathVariable String name) {
return "Hello, " + name + "!";
}
}
In this updated controller:
- We’ve added a
@PathVariable
annotation to thename
parameter in thesayHello
method. This annotation tells Spring to capture the value provided in the URL path. - The
{name}
in the@GetMapping
annotation’s value attribute corresponds to the path variable we want to capture. For example, if a client sends a GET request to/hello/John
, Spring Boot will extract “John” from the URL and pass it to thename
parameter.
Step 2: Test the Enhanced Service:
You can now test your enhanced “Hello World” service by accessing it with different names. The service will respond with personalized greetings based on the provided path variable. Here are some sample requests:
- GET
/hello/John
returns “Hello, John!” - GET
/hello/Alice
returns “Hello, Alice!” - GET
/hello/World
returns “Hello, World!”
Step 3: Run the Application:
Start your Spring Boot application. You can do this by running the main
method in your application’s entry point class or by using the mvn spring-boot:run
or ./gradlew bootRun
command if you’re using Maven or Gradle, respectively.
Step 4: Access the Service:
Once your application is running, open a web browser or use a tool like curl
or Postman to send GET requests to your enhanced “Hello World” service by specifying different names in the URL path.
Enhancing your “Hello World” service with path variables allows you to create more dynamic and interactive endpoints in your Spring Boot application. You can capture user-specific data from the URL and use it to provide customized responses. This approach is particularly useful when building RESTful APIs that need to handle different data based on client requests.