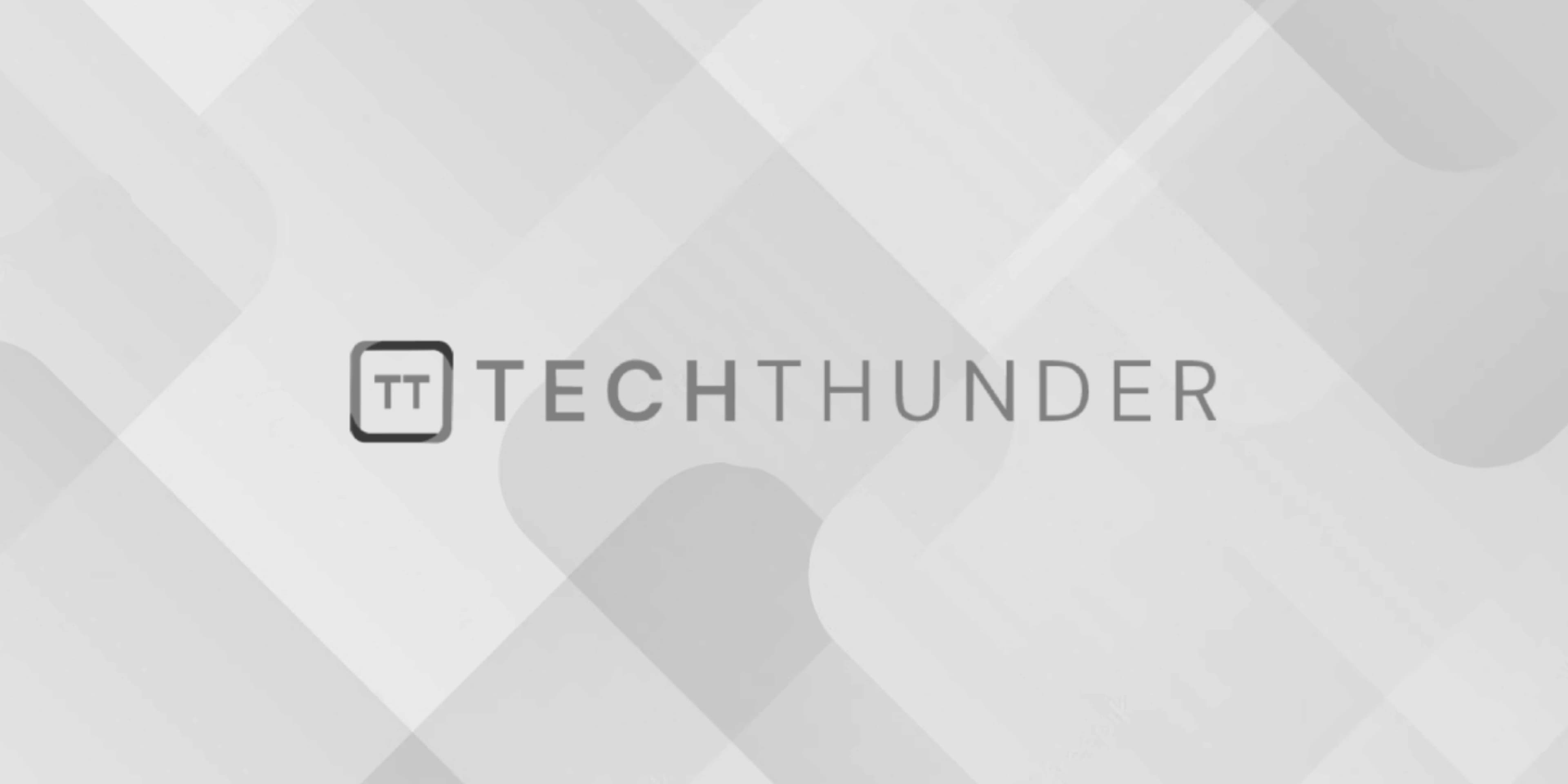
144 views
Connecting RESTful Services to JPA
Connecting RESTful services to JPA (Java Persistence API) in a Spring Boot application involves creating a backend system that exposes RESTful endpoints to interact with a database using JPA for data storage and retrieval. Here’s a step-by-step guide on how to achieve this:
- Setup your Spring Boot Project:
Create a new Spring Boot project using Spring Initializr or your preferred IDE. Include dependencies for “Spring Web,” “Spring Data JPA,” and the database driver of your choice (e.g., H2, MySQL, PostgreSQL). - Define JPA Entity Classes:
Create Java classes that represent your data entities. Annotate them with JPA annotations like@Entity
,@Id
, and others to define the database schema.
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String email;
// Getters and setters
}
- Create JPA Repositories:
Create interfaces that extendJpaRepository
or related interfaces from Spring Data JPA. These repositories provide CRUD operations and more.
public interface UserRepository extends JpaRepository<User, Long> {
}
- Implement REST Controllers:
Create controllers to handle RESTful endpoints. Inject the JPA repository and use it to interact with the database.
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping
public List<User> getAllUsers() {
return userRepository.findAll();
}
@GetMapping("/{id}")
public ResponseEntity<User> getUserById(@PathVariable Long id) {
Optional<User> user = userRepository.findById(id);
return user.map(ResponseEntity::ok).orElse(ResponseEntity.notFound().build());
}
@PostMapping
public User createUser(@RequestBody User user) {
return userRepository.save(user);
}
@PutMapping("/{id}")
public ResponseEntity<User> updateUser(@PathVariable Long id, @RequestBody User newUser) {
Optional<User> existingUser = userRepository.findById(id);
if (existingUser.isPresent()) {
User updatedUser = existingUser.get();
updatedUser.setUsername(newUser.getUsername());
updatedUser.setEmail(newUser.getEmail());
return ResponseEntity.ok(userRepository.save(updatedUser));
} else {
return ResponseEntity.notFound().build();
}
}
@DeleteMapping("/{id}")
public ResponseEntity<Void> deleteUser(@PathVariable Long id) {
userRepository.deleteById(id);
return ResponseEntity.noContent().build();
}
}
- Configure Database Connection:
Set up your database connection details inapplication.properties
orapplication.yml
based on your chosen database. Spring Boot’s auto-configuration will handle most of the database setup. - Run and Test:
Run your Spring Boot application and test the REST endpoints using tools like cURL, Postman, or any web browser.
This setup connects your RESTful services to a database using JPA in a Spring Boot application. It provides a complete backend system that can create, retrieve, update, and delete data through RESTful endpoints while using JPA for data persistence. Make sure to handle error cases, security considerations, and other aspects specific to your application’s requirements.