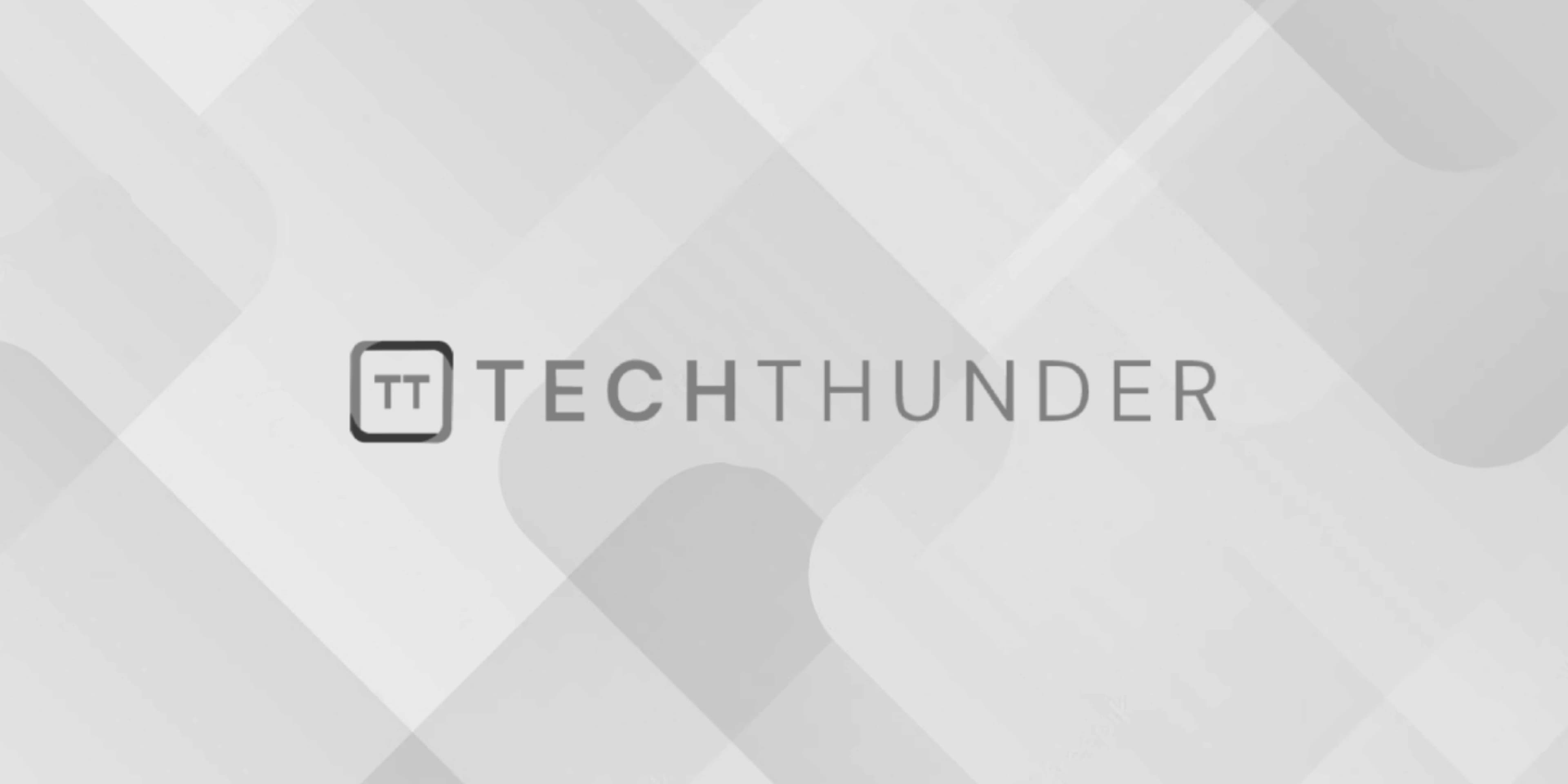
Remoting with Spring
Remoting with Spring refers to the process of invoking methods or functions on remote objects as if they were local objects within a distributed application. Spring provides support for various remoting technologies, allowing you to build distributed systems without having to deal with low-level networking and communication details. The primary goal is to make remote method invocations look like local method calls, abstracting away the complexities of distributed computing.
There are several remoting options available in Spring, each with its own characteristics and use cases. Here are some of the most commonly used Spring remoting technologies:
1. RMI (Remote Method Invocation):
- Use Case: Suitable for Java-to-Java communication within the same network.
- Configuration: Spring supports RMI as a remoting option, allowing you to expose and invoke remote Java objects using RMI.
- Implementation: Spring’s
RmiProxyFactoryBean
andRmiServiceExporter
classes simplify RMI client and server setup.
2. Hessian and Burlap:
- Use Case: Suitable for cross-platform communication, as they are binary protocols.
- Configuration: Spring supports Hessian and Burlap as remoting options, enabling you to expose and consume remote services using these protocols.
- Implementation: Spring provides
HessianProxyFactoryBean
andHessianServiceExporter
for Hessian, andBurlapProxyFactoryBean
andBurlapServiceExporter
for Burlap.
3. HTTP Invoker:
- Use Case: Suitable for invoking remote services over HTTP, which can work with any platform that supports HTTP.
- Configuration: Spring’s
HttpInvokerProxyFactoryBean
andHttpInvokerServiceExporter
classes facilitate HTTP-based remoting. - Implementation: Remote services are accessible via HTTP, typically using Java serialization for communication.
4. JMS (Java Message Service):
- Use Case: Suitable for asynchronous messaging and communication between loosely coupled components.
- Configuration: Spring supports JMS for messaging-based remoting, allowing you to send and receive messages between distributed components.
- Implementation: Spring provides abstractions for sending and receiving JMS messages and integrates with JMS providers like ActiveMQ and RabbitMQ.
5. Spring Cloud for Microservices:
- Use Case: Suitable for building microservices architectures, where services communicate over the network.
- Configuration: Spring Cloud provides tools like Spring Cloud Feign, Spring Cloud Hystrix, and Spring Cloud Ribbon for building and consuming RESTful microservices.
6. RSocket:
- Use Case: Suitable for building reactive and resilient microservices.
- Configuration: Spring supports RSocket as a protocol for reactive remoting, enabling reactive communication between services.
- Implementation: RSocket provides reactive, multiplexed communication over a single connection, which is well-suited for microservices.
In Spring, remoting is typically configured using Spring beans and XML or Java configuration. You define remote interfaces, create remote service proxies, and configure the communication protocol and endpoints accordingly. The choice of remoting technology depends on your specific use case, performance requirements, and the technologies used in your distributed system.
Overall, Spring’s remoting support simplifies the development of distributed applications by abstracting away many of the complexities associated with remote communication, allowing developers to focus on building business logic and services.