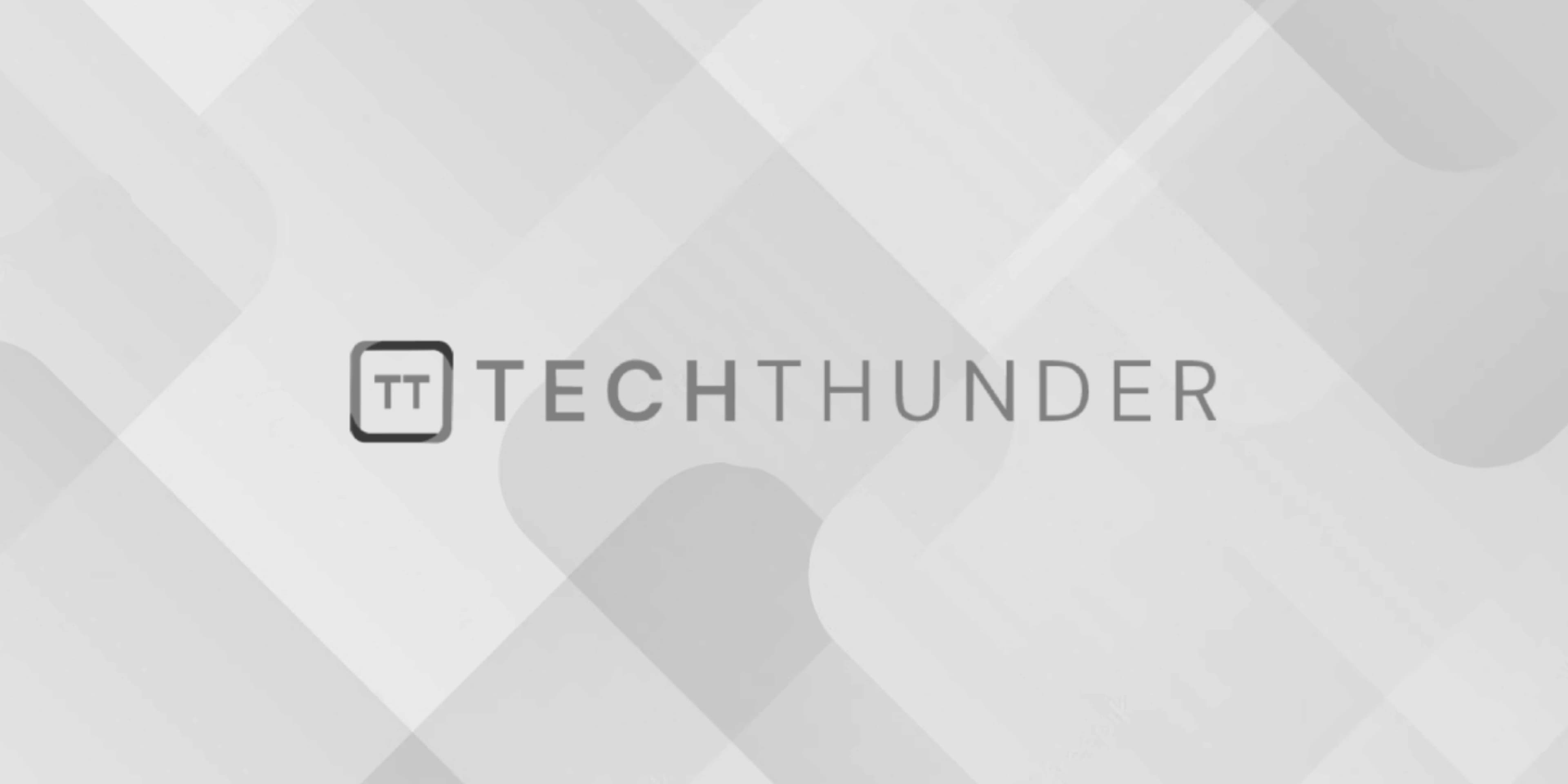
Spring Constructor Injection with Map2
The Spring, you can perform constructor injection with a Map
in various ways. The approach you choose depends on your specific requirements. Here, I’ll provide two different examples of constructor injection with a Map
:
1. Using Map Elements in XML Configuration:
In this approach, you can directly define a Map
of key-value pairs in your XML configuration file and inject it into a bean’s constructor. Let’s say you have a Team
class that takes a Map
of team members’ names and roles:
public class Team {
private Map<String, String> teamMembers;
public Team(Map<String, String> teamMembers) {
this.teamMembers = teamMembers;
}
// Getter and setter for 'teamMembers'
}
Here’s how you can configure the beans in your XML configuration file:
<bean id="team" class="com.example.Team">
<constructor-arg>
<map>
<entry key="leader" value="John" />
<entry key="member" value="Alice" />
</map>
</constructor-arg>
</bean>
In this configuration:
- We define a “team” bean of class
com.example.Team
and inject aMap
of team members’ names and roles using the<map>
element.
2. Using a Properties File for Map Values:
Another approach is to store the map values in a properties file and use Spring’s util:properties
element to load the properties file and inject the properties as a java.util.Properties
object. Then, you can convert the Properties
object to a Map
in your bean’s constructor. Here’s how to do it:
Create a properties file (e.g., team.properties):
teamMembers.leader=John
teamMembers.member=Alice
Configure the beans in your XML configuration file:
<util:properties id="teamMembers" location="classpath:team.properties" />
<bean id="team" class="com.example.Team">
<constructor-arg>
<util:map>
<entry key="teamMembers" value-ref="teamMembers" />
</util:map>
</constructor-arg>
</bean>
In this configuration:
- We use the
<util:properties>
element to load the properties from the “team.properties” file and store them in the “teamMembers” bean. - We define a “team” bean of class
com.example.Team
and inject aMap
of team members using the<util:map>
element, referring to the “teamMembers” bean as the value.
With this approach, you can manage map values in an external properties file, which can be beneficial for configuration and localization purposes.
Choose the approach that best fits your use case and organization’s preferences. Both methods allow you to perform constructor injection with a Map
in Spring.