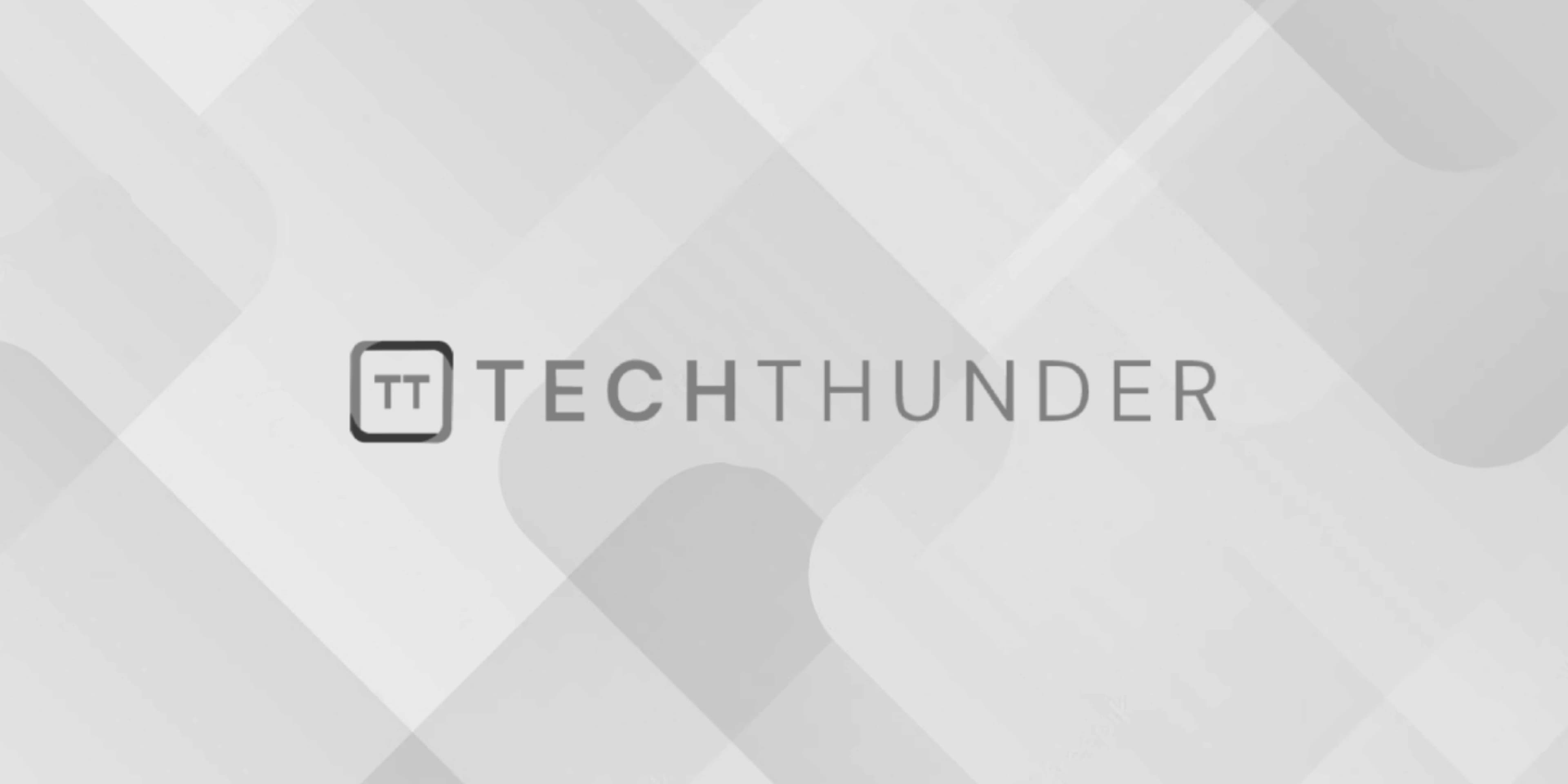
Spring Burlap
Burlap is another binary web service protocol similar to Hessian that allows you to create and consume remote services over HTTP. Just like Hessian, Spring provides support for Burlap-based remoting, enabling you to build distributed systems where the client and server communicate using the Burlap protocol. Here’s how to use Spring’s Burlap support:
1. Define the Service Interface:
Start by defining the service interface that both the client and server will use. This interface should contain the methods that the client will invoke remotely.
public interface MyRemoteService {
String sayHello(String name);
}
2. Implement the Service:
Create a class that implements the service interface. This class will provide the actual implementation of the remote methods.
public class MyRemoteServiceImpl implements MyRemoteService {
@Override
public String sayHello(String name) {
return "Hello, " + name + "!";
}
}
3. Configure the Burlap Service:
In your Spring configuration, define the Burlap service by using the BurlapServiceExporter
class. This class exposes the service over HTTP using the Burlap protocol.
<bean id="myRemoteService" class="com.example.MyRemoteServiceImpl"/>
<bean id="burlapServiceExporter" class="org.springframework.remoting.caucho.BurlapServiceExporter">
<property name="service" ref="myRemoteService"/>
<property name="serviceInterface" value="com.example.MyRemoteService"/>
</bean>
4. Create the Burlap Client:
On the client side, configure Spring to create a Burlap proxy for the remote service.
<bean id="myRemoteServiceProxy" class="org.springframework.remoting.caucho.BurlapProxyFactoryBean">
<property name="serviceUrl" value="http://localhost:8080/myRemoteService"/>
<property name="serviceInterface" value="com.example.MyRemoteService"/>
</bean>
In this example, we specify the URL of the Burlap service (including the host and port) and the service interface. The myRemoteServiceProxy
bean can be injected into your client code for remote method invocation.
5. Use the Burlap Service:
Now you can use the myRemoteServiceProxy
bean to invoke methods on the remote service just like you would with a local bean.
public class MyBurlapClient {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
MyRemoteService remoteService = (MyRemoteService) context.getBean("myRemoteServiceProxy");
String response = remoteService.sayHello("John");
System.out.println(response);
}
}
6. Run the Burlap Server and Client:
Start the Burlap server (where the service is hosted) and then run the Burlap client to make remote method calls.
7. Security Considerations:
As with Hessian, when using Burlap, especially in a production environment or over the internet, it’s important to consider security. You may need to secure your Burlap endpoints, configure authentication and authorization mechanisms, and use HTTPS for secure communication.
This example provides a basic introduction to using Spring’s Burlap support. Spring simplifies the configuration and usage of Burlap, making it easier to develop distributed Java applications over HTTP with binary serialization.