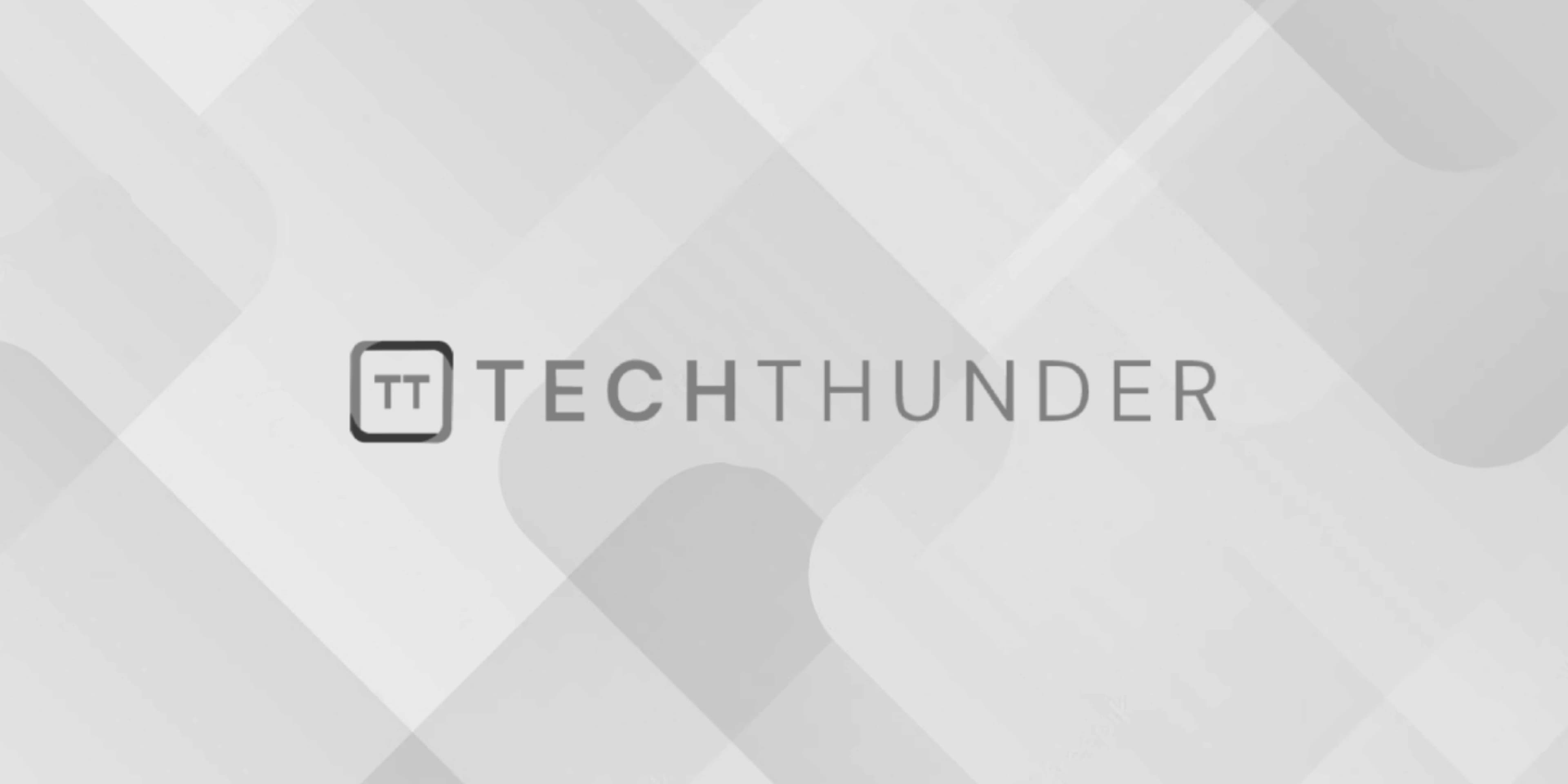
Spring SimpleJdbcTemplate
SimpleJdbcTemplate
was a class provided by Spring Framework for simplifying database operations using JDBC (Java Database Connectivity). However, it’s important to note that SimpleJdbcTemplate
has been deprecated starting from Spring Framework 3.1, and it’s not recommended to use it in modern Spring applications. Instead, you should use the JdbcTemplate
class, which provides more advanced features and better integration with the Spring ecosystem.
Here’s an example of how you can use the JdbcTemplate
class to perform database operations in a Spring application:
- Configure DataSource: You need to configure a
DataSource
bean in your Spring configuration file. This bean provides the database connection information.
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/mydatabase" />
<property name="username" value="username" />
<property name="password" value="password" />
</bean>
- Create
JdbcTemplate
Bean: Define aJdbcTemplate
bean in your Spring configuration. Spring will inject theDataSource
bean automatically.
<bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<constructor-arg ref="dataSource" />
</bean>
- Use
JdbcTemplate
in Your DAO or Service: In your DAO (Data Access Object) or service class, inject theJdbcTemplate
bean, and use it to perform database operations.
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Repository;
@Repository
public class MyDao {
private final JdbcTemplate jdbcTemplate;
@Autowired
public MyDao(JdbcTemplate jdbcTemplate) {
this.jdbcTemplate = jdbcTemplate;
}
public String findNameById(int id) {
String sql = "SELECT name FROM users WHERE id = ?";
return jdbcTemplate.queryForObject(sql, String.class, id);
}
// Other database operations go here
}
In this example, the JdbcTemplate
is injected into the MyDao
class, and it’s used to execute SQL queries.
- Use Transactions (Optional): You can also configure transactions for your database operations by using Spring’s transaction management. You can annotate your service methods with
@Transactional
to enable transactional behavior.
import org.springframework.transaction.annotation.Transactional;
@Service
public class MyService {
private final MyDao myDao;
@Autowired
public MyService(MyDao myDao) {
this.myDao = myDao;
}
@Transactional
public String findNameById(int id) {
return myDao.findNameById(id);
}
// Other service methods go here
}
By annotating methods with @Transactional
, Spring will automatically manage transactions for those methods.
- Use Spring Configuration: Don’t forget to configure your Spring application context to scan for components (e.g.,
@Repository
,@Service
, etc.) and set up appropriate package scans to discover your DAO and service beans. - Application Setup: Set up your Spring application configuration and create the necessary beans, including the
JdbcTemplate
and your DAO and service beans.
With this approach, you can perform database operations using the JdbcTemplate
class in a Spring application. It offers better control, flexibility, and integration compared to the deprecated SimpleJdbcTemplate
.