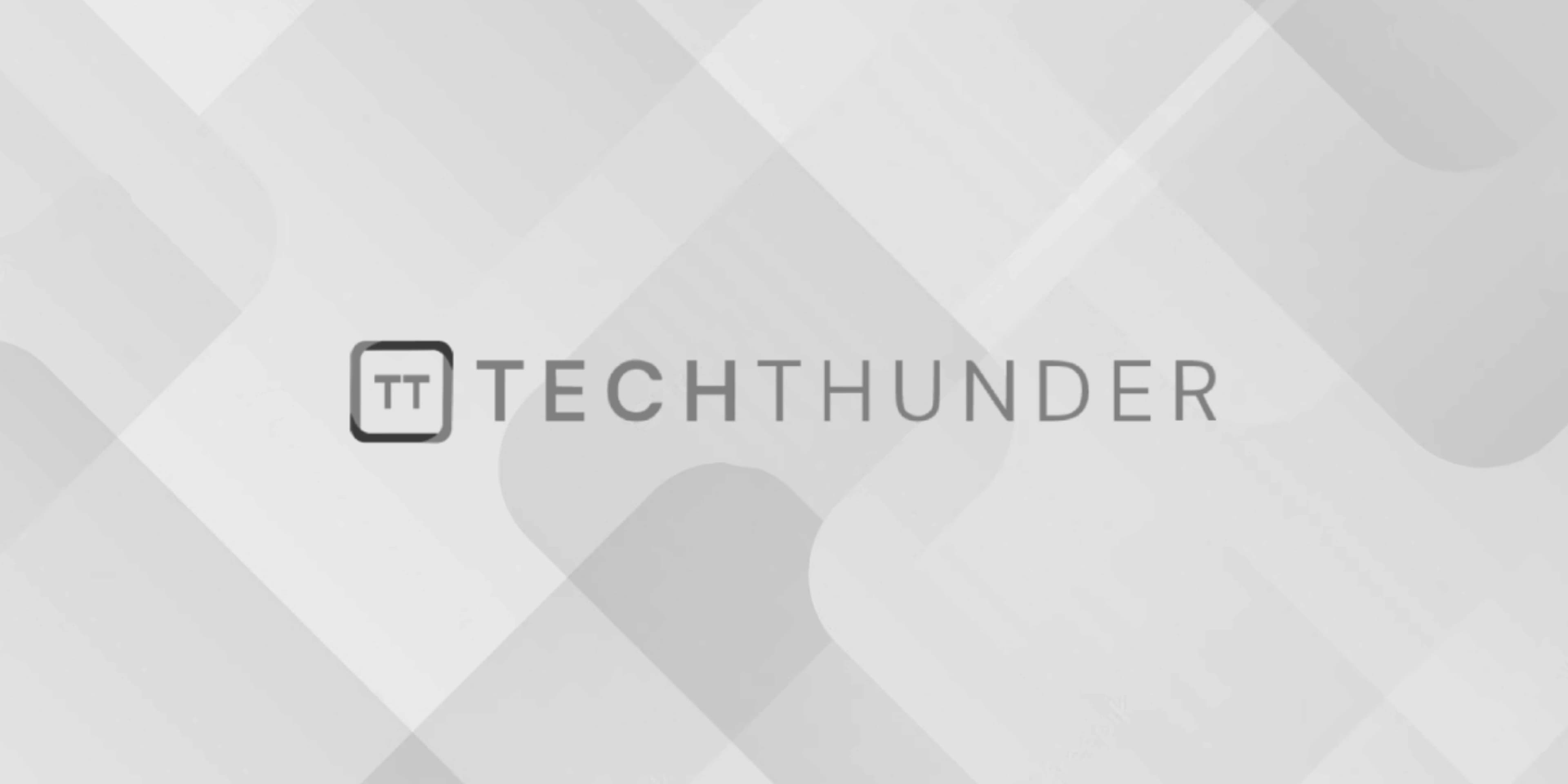
Spring PreparedStatement
The Spring JDBC, you can use PreparedStatement
to execute parameterized SQL queries safely and efficiently. A PreparedStatement
is a precompiled SQL statement that allows you to bind parameters to the query, which helps prevent SQL injection attacks and can improve query performance. Here’s how to use PreparedStatement
with Spring JdbcTemplate:
1. Configure DataSource and JdbcTemplate:
First, configure your DataSource and JdbcTemplate as explained in the previous example.
2. Create a DAO Class:
Create a DAO (Data Access Object) class that uses JdbcTemplate and PreparedStatement to perform parameterized queries. For example, let’s create a UserDao class to query user information based on a username:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Repository;
@Repository
public class UserDao {
private final JdbcTemplate jdbcTemplate;
@Autowired
public UserDao(JdbcTemplate jdbcTemplate) {
this.jdbcTemplate = jdbcTemplate;
}
public User getUserByUsername(String username) {
String sql = "SELECT * FROM users WHERE username = ?";
return jdbcTemplate.queryForObject(sql, new Object[]{username}, new UserRowMapper());
}
// Other database operations go here
}
In this example, we create a parameterized query using ?
as a placeholder for the username. We pass the actual username value as an argument when executing the query.
3. Create a RowMapper:
You may need to create a custom RowMapper to map the query result to your domain object. Here’s an example of a UserRowMapper class:
import org.springframework.jdbc.core.RowMapper;
import java.sql.ResultSet;
import java.sql.SQLException;
public class UserRowMapper implements RowMapper<User> {
@Override
public User mapRow(ResultSet rs, int rowNum) throws SQLException {
User user = new User();
user.setId(rs.getLong("id"));
user.setUsername(rs.getString("username"));
user.setEmail(rs.getString("email"));
// Map other fields as needed
return user;
}
}
4. Use the DAO in Your Application:
You can use the UserDao in your application to retrieve user information based on a username:
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Main {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
UserDao userDao = context.getBean(UserDao.class);
String username = "john_doe";
User user = userDao.getUserByUsername(username);
System.out.println("User ID: " + user.getId());
System.out.println("Username: " + user.getUsername());
System.out.println("Email: " + user.getEmail());
}
}
In this example, we pass the username
as a parameter to the getUserByUsername
method, which uses a PreparedStatement
to safely query the database based on the provided parameter.
Using PreparedStatement
with Spring JdbcTemplate is a recommended practice for executing parameterized SQL queries, as it helps protect against SQL injection and improves performance compared to executing raw SQL queries with concatenated parameters.