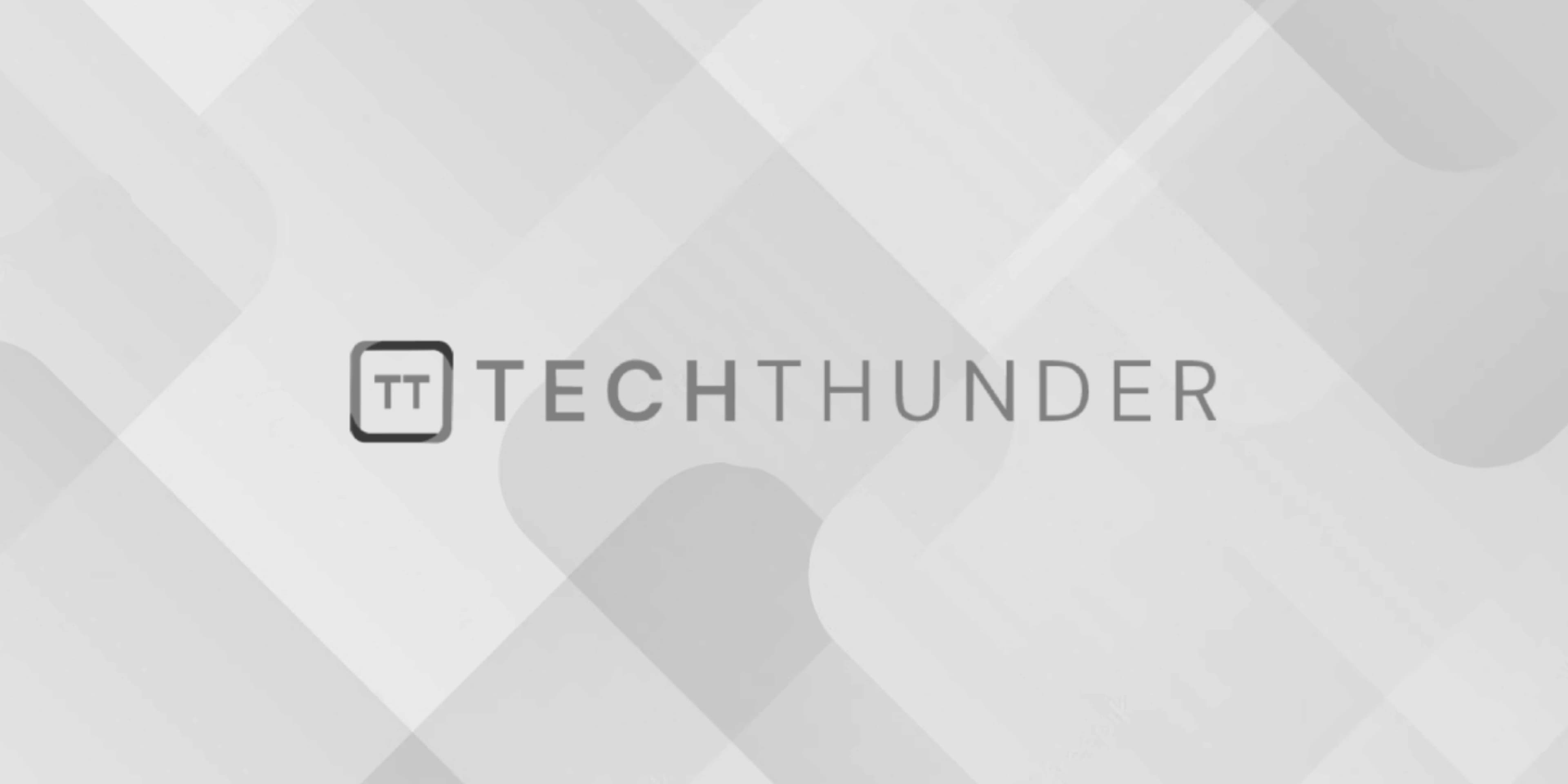
Spring Constructor Injection Dependent Object
The Spring, constructor injection with dependent objects involves injecting one or more dependencies (beans) into a target bean’s constructor. These dependent objects are then used within the target bean to perform various tasks. Here’s how you can perform constructor injection with dependent objects in Spring:
1. Create Dependent Objects:
First, create the dependent objects (beans) that you want to inject into another bean. These dependent objects should be defined as separate Spring beans. For example, let’s create a Person
class and a Address
class:
public class Person {
private String name;
private Address address;
// Constructor with dependencies
public Person(String name, Address address) {
this.name = name;
this.address = address;
}
// Getter and setter methods for 'name' and 'address'
}
public class Address {
private String street;
private String city;
// Constructor
public Address(String street, String city) {
this.street = street;
this.city = city;
}
// Getter and setter methods for 'street' and 'city'
}
2. Configure Beans in XML:
In your Spring configuration file (e.g., applicationContext.xml
), define the beans and specify the constructor injection by providing the constructor arguments. In this case, you’ll inject the Address
bean into the Person
bean’s constructor.
<bean id="johnsAddress" class="com.example.Address">
<constructor-arg value="123 Main St" />
<constructor-arg value="Springfield" />
</bean>
<bean id="john" class="com.example.Person">
<constructor-arg value="John" />
<constructor-arg ref="johnsAddress" />
</bean>
In this XML configuration:
- We define a “johnsAddress” bean of class
com.example.Address
and provide constructor arguments for thestreet
andcity
. - We define a “john” bean of class
com.example.Person
and inject “John” as the name and the “johnsAddress” bean as theAddress
dependency.
3. Access the Target Bean:
You can access the target bean, “john,” by retrieving it from the Spring application context. The Address
bean will be injected into the Person
bean’s constructor when it is created.
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
Person john = context.getBean("john", Person.class);
// Use the 'john' bean, which now has an 'Address' dependency
Constructor injection with dependent objects allows you to ensure that a bean has all its required dependencies when it is instantiated. It’s a useful approach for creating more complex beans that rely on other beans to perform their tasks.