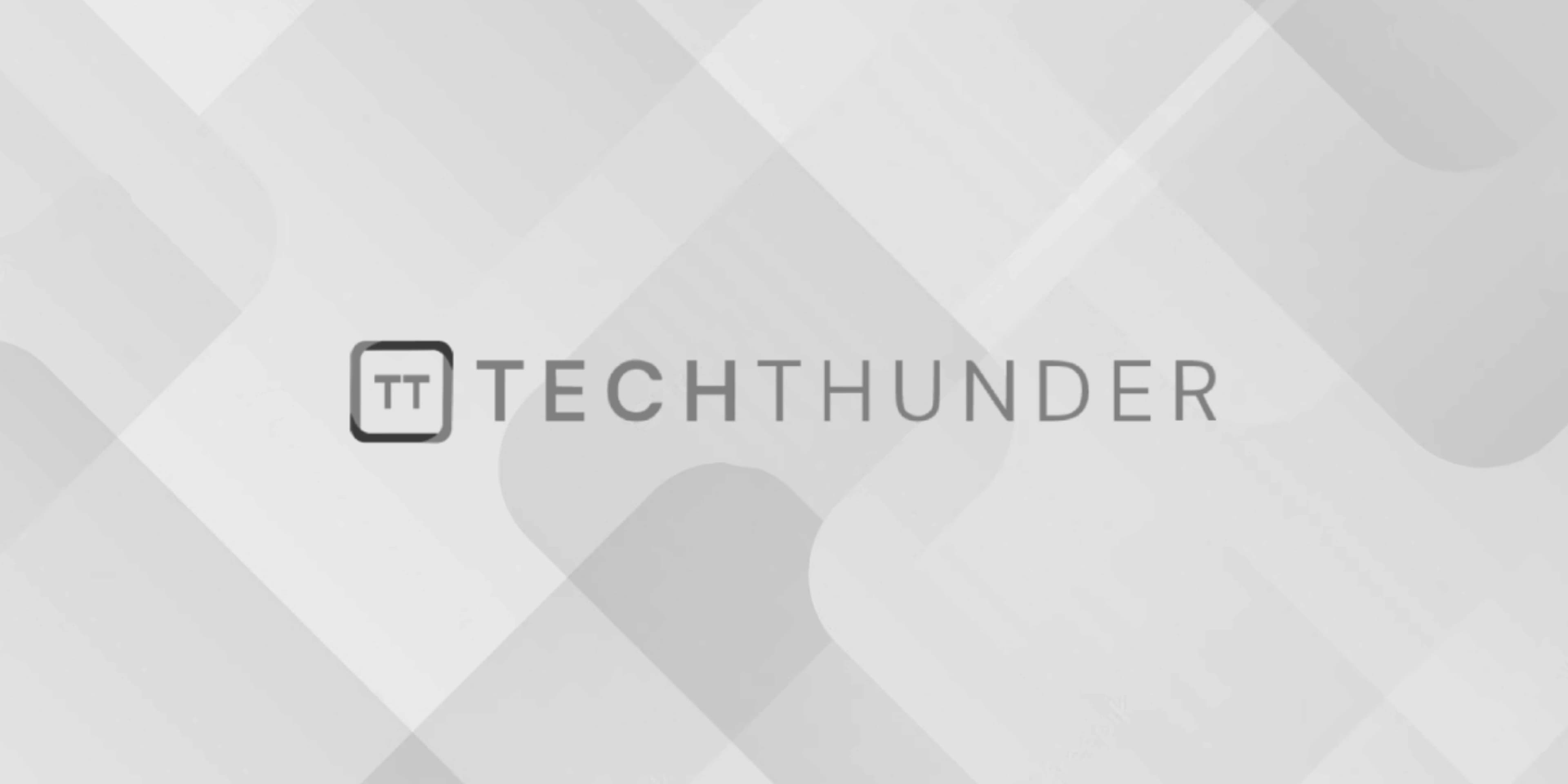
Spring Multiple Controller
The Spring MVC application, you can have multiple controllers to handle different parts of your web application. Each controller is responsible for handling requests related to specific functionality or features. Here’s how you can create and manage multiple controllers in a Spring MVC application:
1. Create Controller Classes:
Create multiple controller classes, each responsible for handling a specific set of related requests. Controller classes should be annotated with @Controller
or @RestController
to indicate that they are Spring MVC controllers. For example:
@Controller
public class HomeController {
@GetMapping("/")
public String home() {
return "home";
}
}
@Controller
public class UserController {
@GetMapping("/users")
public String listUsers() {
// Logic to retrieve and display a list of users
return "user/list";
}
@GetMapping("/users/{id}")
public String viewUser(@PathVariable Long id, Model model) {
// Logic to retrieve and display a user by ID
return "user/view";
}
}
In this example, we have two controllers: HomeController
and UserController
. HomeController
handles requests to the root URL (“/”), while UserController
handles requests related to user management.
2. Configure Component Scanning:
Ensure that Spring is scanning the packages containing your controller classes. This is typically done through component scanning in your Spring configuration, either using XML configuration or Java-based configuration (using @ComponentScan
). For example, in a Spring Boot application, component scanning is automatically enabled:
@SpringBootApplication
public class YourApplication {
public static void main(String[] args) {
SpringApplication.run(YourApplication.class, args);
}
}
3. Create Views:
Create view templates (e.g., JSP, Thymeleaf, or FreeMarker templates) for each controller’s views. Make sure the view names returned by your controller methods match the names of the view templates. For example, if a controller method returns “user/list,” create a corresponding “list.jsp” or “list.html” view template.
4. Define Request Mappings:
Define request mappings within each controller class using annotations like @GetMapping
, @PostMapping
, @RequestMapping
, etc., to specify which methods should handle specific URL patterns. For example:
@Controller
@RequestMapping("/users")
public class UserController {
@GetMapping("/list")
public String listUsers() {
// Logic to retrieve and display a list of users
return "user/list";
}
@GetMapping("/{id}")
public String viewUser(@PathVariable Long id, Model model) {
// Logic to retrieve and display a user by ID
return "user/view";
}
}
In this example, all methods in the UserController
class are mapped under the “/users” URL pattern.
5. Access Different Controllers:
Now you can access different controllers and their respective actions by navigating to the defined URLs. For example:
- Access the home page:
http://localhost:8080/
- List users:
http://localhost:8080/users/list
- View a user:
http://localhost:8080/users/{id}
Each controller handles its designated set of requests, and the appropriate view templates are rendered.
That’s how you can create and manage multiple controllers in a Spring MVC application to handle various parts of your web application’s functionality. Each controller class is responsible for specific actions and associated views.