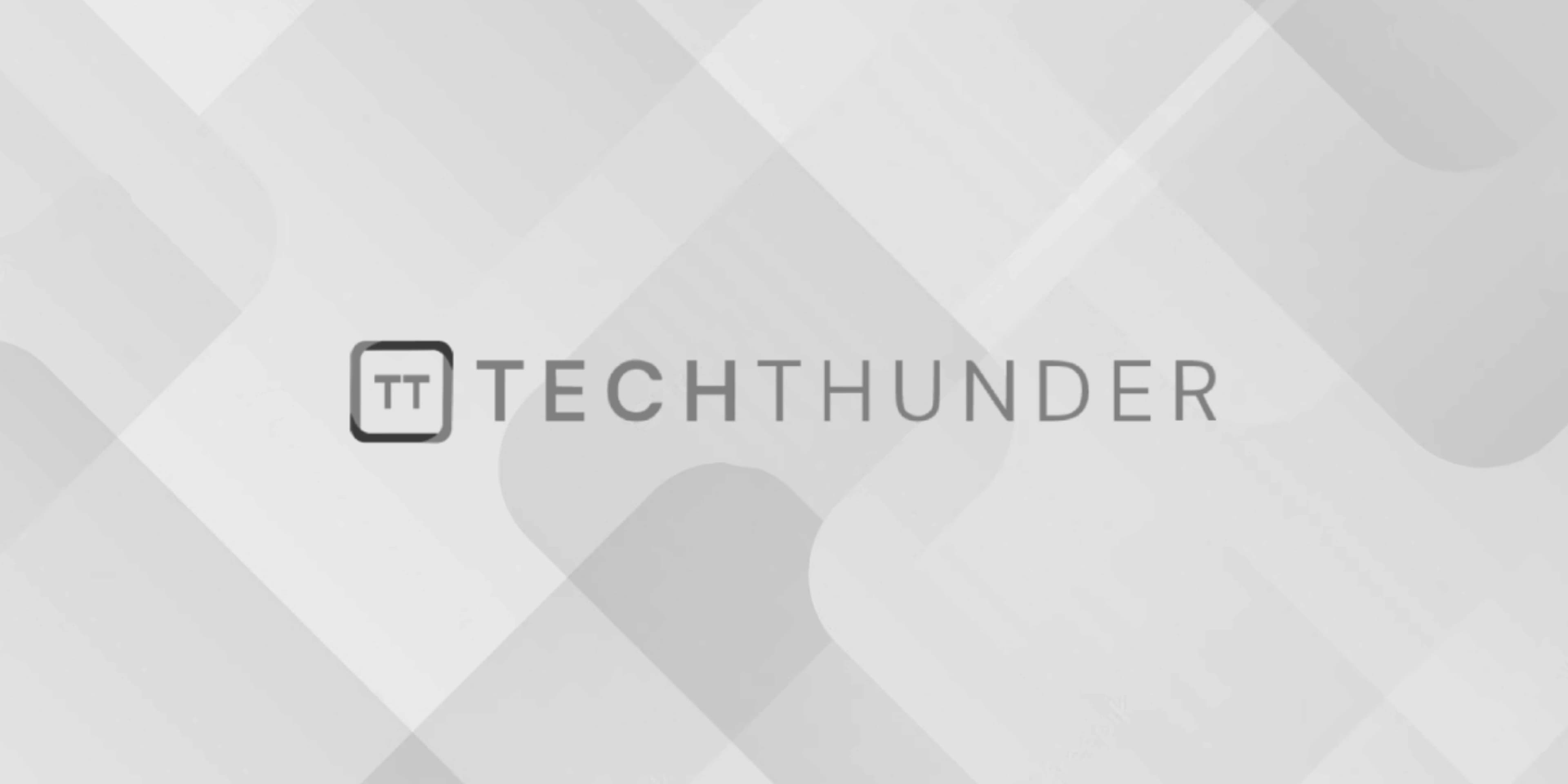
Spring Model Interface
The Spring MVC, the Model
interface is a fundamental part of the framework’s model-view-controller architecture. It’s used to pass data from a controller to a view, allowing you to display dynamic information in your web pages. The Model
interface is typically provided to your controller methods as a parameter, allowing you to add attributes that will be used in the view rendering process.
Here’s how you can use the Model
interface in a Spring MVC application:
- Injecting Model into Controller Methods: In your Spring MVC controller methods, you can add a
Model
parameter to access theModel
interface. When the controller method is invoked, Spring automatically provides an instance ofModel
to your method.
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class MyController {
@GetMapping("/example")
public String example(Model model) {
// Add data to the model
model.addAttribute("message", "Hello, Spring MVC!");
// Return the view name
return "example-view";
}
}
- Adding Data to the Model: Inside your controller method, you can use the
addAttribute
method of theModel
interface to add data that you want to pass to the view. The data is typically stored as key-value pairs, where the key is a string identifier and the value is the actual data.
model.addAttribute("message", "Hello, Spring MVC!");
model.addAttribute("user", currentUser);
- Accessing Data in the View: In your view templates (e.g., JSP, Thymeleaf, FreeMarker), you can access the data added to the
Model
by using the key you provided when adding the attribute. For example, in a Thymeleaf template:
<html>
<body>
<h1 th:text="${message}"></h1>
<p th:text="${user.name}"></p>
</body>
</html>
Here, ${message}
and ${user.name}
are placeholders that will be replaced with the data stored in the Model
.
- Returning the View Name: Finally, your controller method should return the name of the view that should be rendered after the data is added to the
Model
. This view name corresponds to the actual view template you want to display.
return "example-view";
Spring MVC will then look for a view template with this name (e.g., “example-view.jsp” or “example-view.html”) and render it, passing the data from the Model
to the view.
The Model
interface is a powerful tool for passing data between controllers and views in Spring MVC, allowing you to create dynamic web pages with ease. It’s a core part of the framework’s support for the model-view-controller pattern.