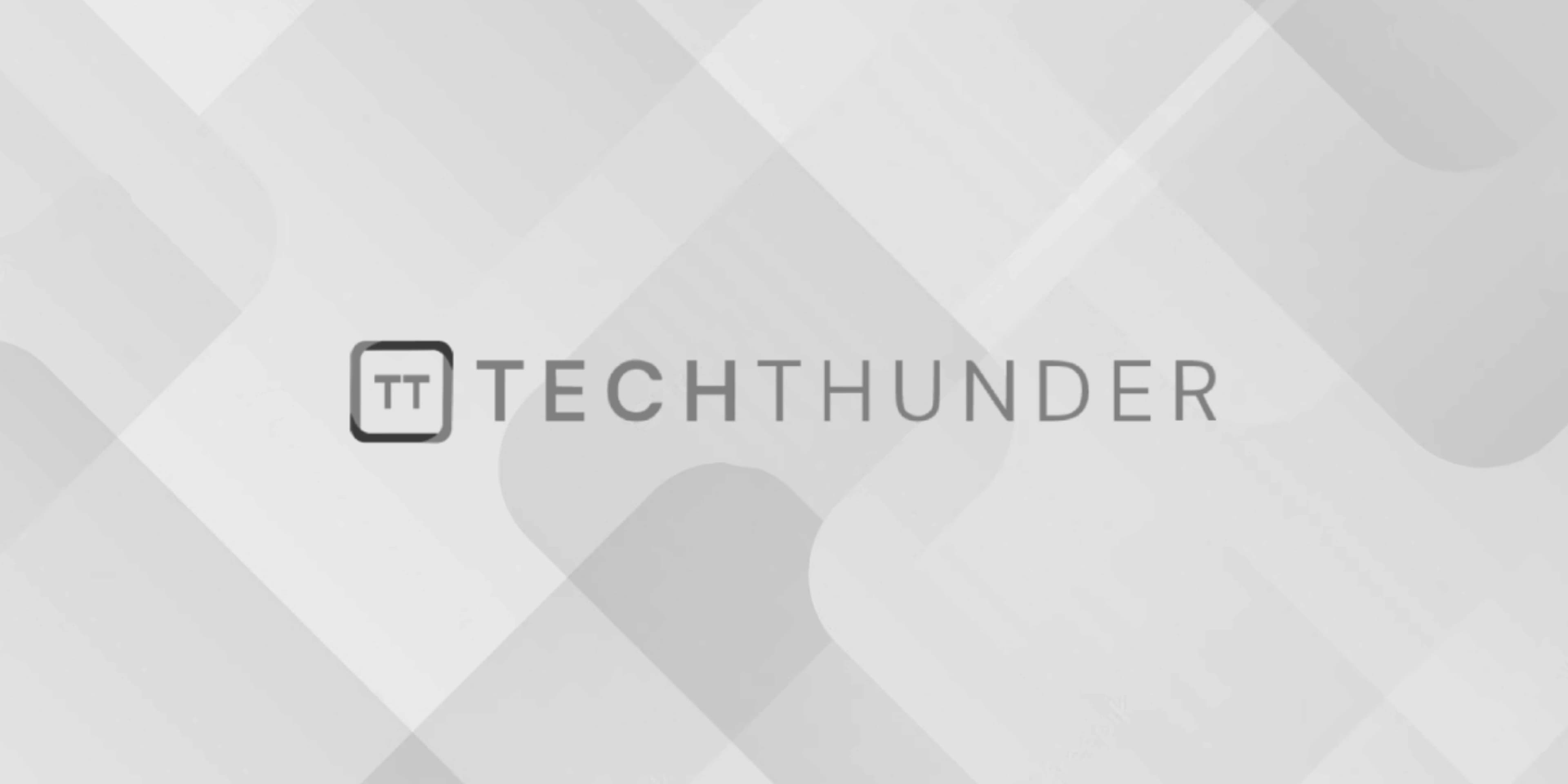
Spring Security Java Example
The example of configuring Spring Security using Java-based configuration. In this example, we’ll set up a basic Spring Security configuration for form-based authentication.
1. Create a Spring Boot Project:
Start by creating a new Spring Boot project using Spring Initializr or your preferred development environment.
2. Configure Spring Security using Java:
Create a security configuration class that extends WebSecurityConfigurerAdapter
. This class will contain your Spring Security configuration using Java annotations.
src/main/java/com/example/demo/config/SecurityConfig.java
:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/home").permitAll()
.antMatchers("/admin").hasRole("ADMIN")
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.defaultSuccessUrl("/home")
.permitAll()
.and()
.logout()
.logoutSuccessUrl("/login?logout")
.permitAll();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password("{noop}password").roles("USER")
.and()
.withUser("admin").password("{noop}adminpassword").roles("ADMIN");
}
}
In this configuration class, we define access rules, authentication settings, and user roles.
3. Create Controllers and Templates:
Create the necessary controllers and Thymeleaf templates for handling login, logout, and different pages, as explained in the XML example.
4. Run the Application:
Run your Spring Boot application and navigate to http://localhost:8080/login
to see the login page. You can log in with the provided user and admin credentials.
Remember that the {noop}
prefix in the passwords indicates that the passwords are stored in plain text for this example. In a real application, you should use a proper password encoder for security.
Using Java-based configuration offers greater flexibility and is more aligned with modern Spring practices. It allows you to take advantage of the full power of the Java language and IDE features while configuring your security settings.