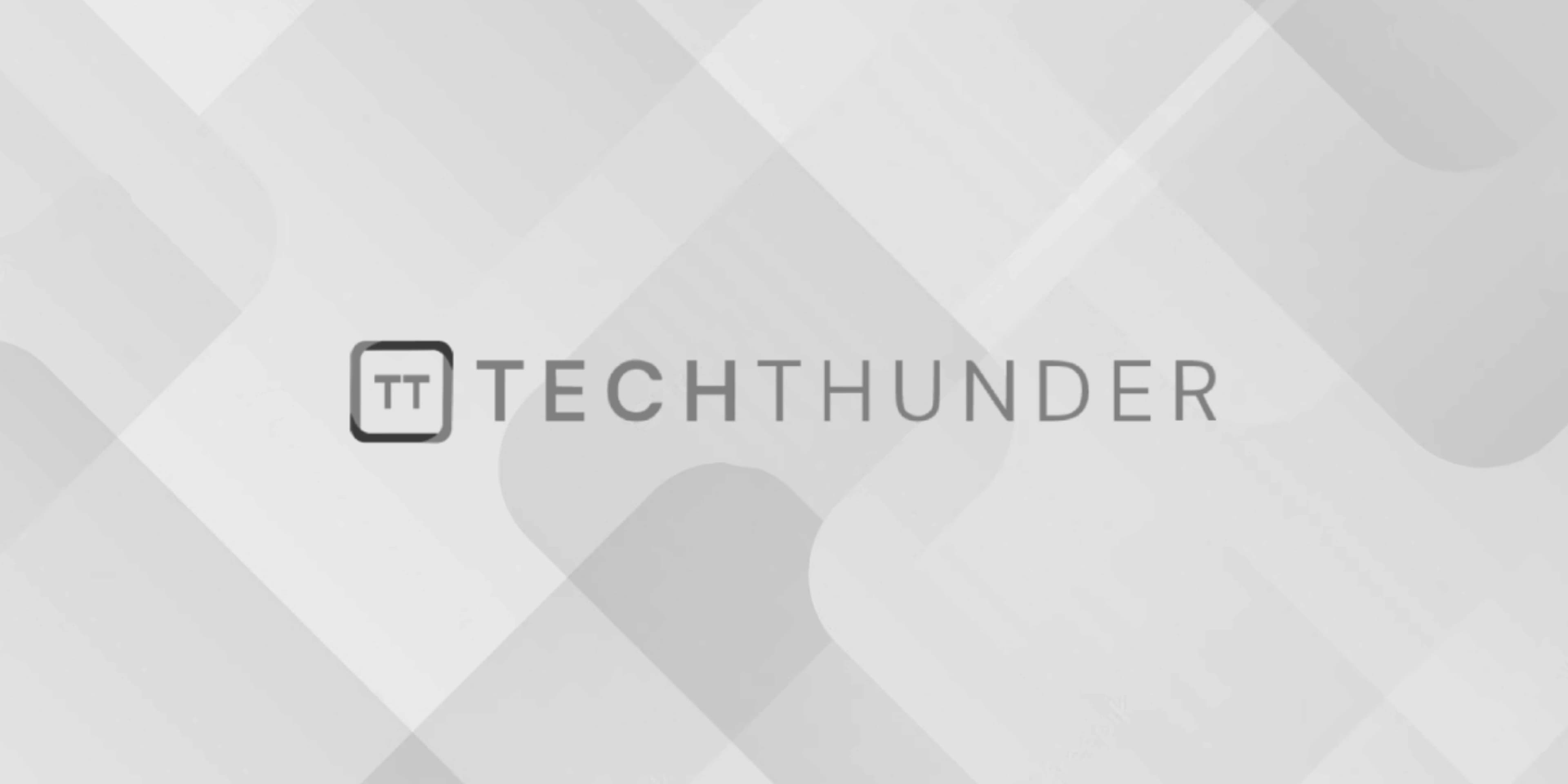
Spring File Upload Example
The example will show you how to create a Spring MVC application that allows users to upload files. We’ll use Spring Boot for simplicity, but the same principles apply to a standard Spring MVC project.
1. Set Up Your Project:
Create a new Spring Boot project or add the required dependencies to your existing project. You’ll need the following dependencies:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
2. Create a File Upload Form:
Create an HTML form that allows users to select and upload files. You can use Thymeleaf or plain HTML. Here’s a simple HTML form:
<!DOCTYPE html>
<html>
<head>
<title>File Upload Example</title>
</head>
<body>
<h1>Upload a File</h1>
<form method="POST" action="/upload" enctype="multipart/form-data">
<input type="file" name="file" accept=".txt, .pdf, .doc">
<input type="submit" value="Upload">
</form>
</body>
</html>
3. Create a Controller:
Create a Spring MVC controller to handle file uploads. The controller should have a method to display the upload form and another method to process the uploaded file.
@Controller
public class FileUploadController {
@GetMapping("/upload-form")
public String uploadForm() {
return "upload-form";
}
@PostMapping("/upload")
public String uploadFile(@RequestParam("file") MultipartFile file, RedirectAttributes redirectAttributes) {
if (file.isEmpty()) {
redirectAttributes.addFlashAttribute("message", "Please select a file to upload.");
return "redirect:/upload-form";
}
try {
// You can save the file or process it as needed.
// For example, you can save it to a directory or store it in a database.
// Here, we'll just print the file information.
System.out.println("Uploaded File Name: " + file.getOriginalFilename());
System.out.println("File Size: " + file.getSize());
redirectAttributes.addFlashAttribute("message", "File uploaded successfully.");
} catch (Exception e) {
redirectAttributes.addFlashAttribute("message", "Failed to upload the file.");
e.printStackTrace();
}
return "redirect:/upload-form";
}
}
4. Configure Application Properties:
Ensure that you have configured the maximum file upload size in your application.properties
or application.yml
:
spring.servlet.multipart.max-file-size=10MB
spring.servlet.multipart.max-request-size=10MB
5. Create a Success Page:
Create an HTML page (e.g., success.html
) to display a success message after a file is uploaded successfully.
6. Run Your Application:
Run your Spring Boot application, and you can access the file upload form at /upload-form
. Users can select a file, click the “Upload” button, and the uploaded file’s information will be displayed. If there’s an error, an appropriate message will be shown.
That’s it! You’ve created a simple Spring File Upload example. You can extend this example to handle various file types, save files to a specific directory, or store file metadata in a database, depending on your application’s requirements.