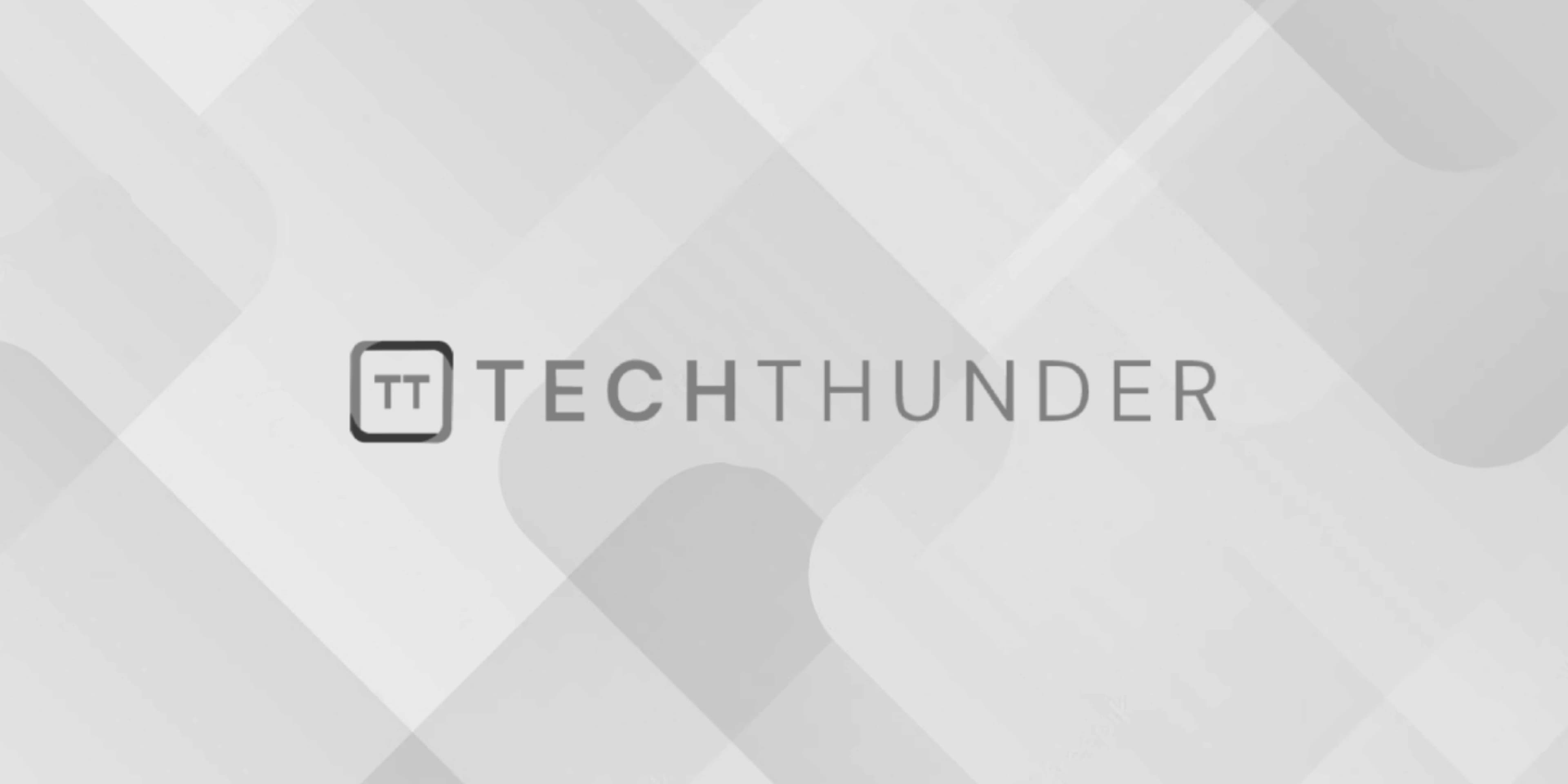
Spring Form Radio Button
The Spring MVC, you can create radio buttons in a form using the <form:radiobutton>
tag from the Spring Form Tag Library. Radio buttons are typically used when you want the user to choose a single option from a list of mutually exclusive options. Here’s how to create radio buttons in a Spring form:
- Include the Spring Form Tag Library: At the top of your JSP file, include the taglib declaration for the Spring Form Tag Library:
<%@ taglib prefix="form" uri="http://www.springframework.org/tags/form" %>
- Create a Form and Radio Buttons: In your JSP file, use the
<form:form>
tag to create a form, and then use the<form:radiobutton>
tag to create radio buttons. You can bind the selected value to a property of a model object using thepath
attribute. Also, set thevalue
attribute to define the value of each radio button and thelabel
attribute to provide a label for the option.
<form:form modelAttribute="user" method="POST" action="/submit">
<div>
<label>Gender:</label>
<form:radiobutton path="gender" value="male" label="Male" />
<form:radiobutton path="gender" value="female" label="Female" />
<form:radiobutton path="gender" value="other" label="Other" />
</div>
<!-- Other form fields go here -->
<div>
<input type="submit" value="Submit" />
</div>
</form:form>
In this example, we’re creating radio buttons for the “gender” property of the “user” model object.
- Handle Form Submission in a Controller: In your Spring MVC controller, handle the form submission and bind the form data to a model object. You can then process the data as needed.
@Controller
public class UserController {
@GetMapping("/form")
public String showForm(Model model) {
model.addAttribute("user", new User()); // Initialize the model object
return "form";
}
@PostMapping("/submit")
public String submitForm(@ModelAttribute("user") User user) {
// Process the user object (e.g., save to a database)
return "result"; // Redirect to a result page
}
}
In this example, the @ModelAttribute
annotation is used to bind the form data to a “user” model object.
- Validation and Error Handling (Optional): You can add validation rules to your model object using annotations (e.g.,
@NotNull
,@Size
, etc.). If validation fails, Spring MVC will automatically handle error messages. You can display these error messages next to the radio buttons in your JSP using<form:errors>
tags.
public class User {
@NotNull(message = "Gender is required")
private String gender;
// Getters and setters
}
<div>
<label>Gender:</label>
<form:radiobutton path="gender" value="male" label="Male" />
<form:radiobutton path="gender" value="female" label="Female" />
<form:radiobutton path="gender" value="other" label="Other" />
<form:errors path="gender" cssClass="error" />
</div>
This setup allows you to create radio buttons in a Spring MVC form, bind them to a model object, and handle form submissions, including validation and error handling. Users can select one option from the radio button group, and the selected value will be bound to the model object’s property.