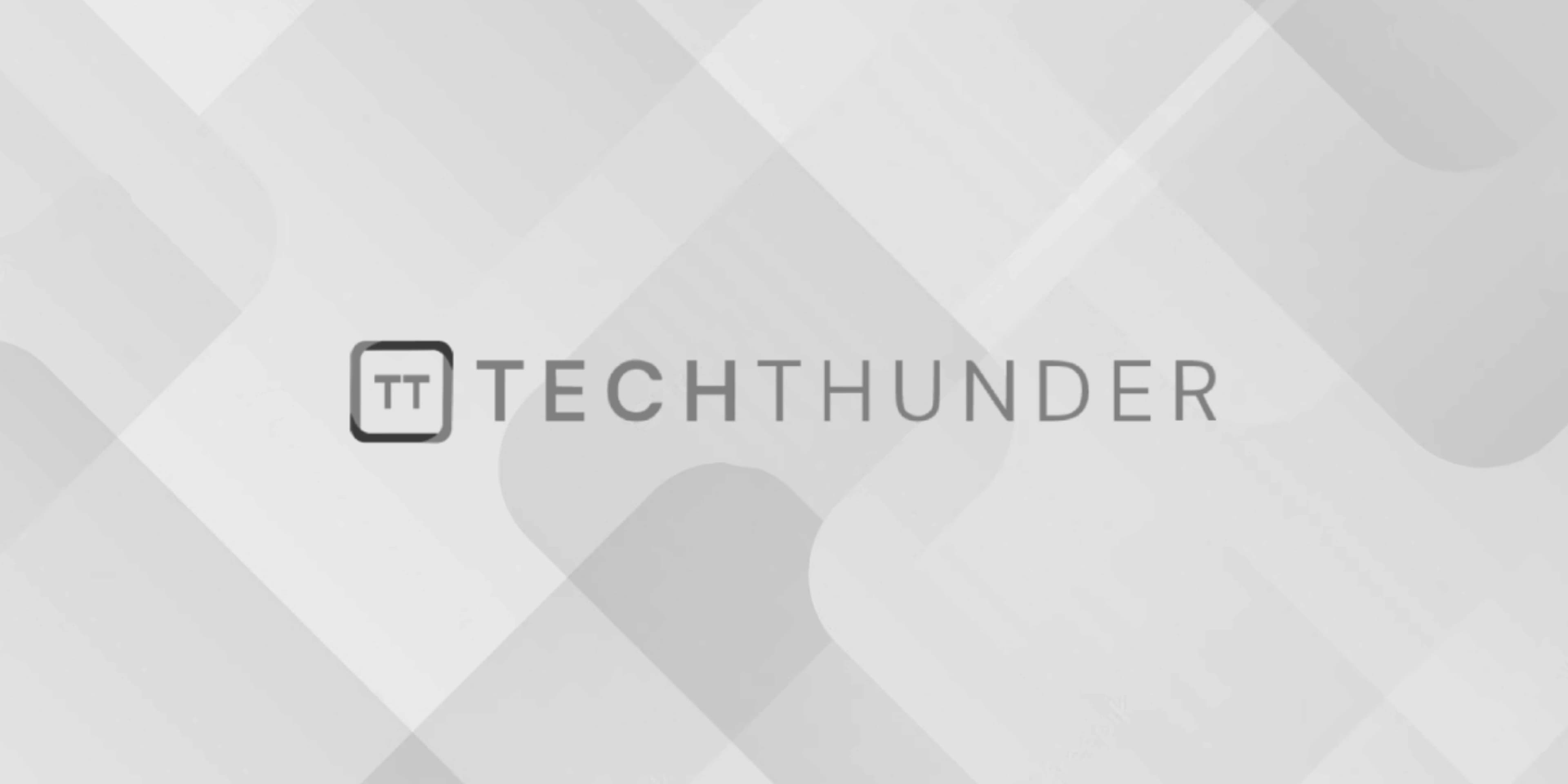
Spring MVC Validation
Spring MVC provides robust support for data validation using annotations, custom validation logic, and error handling mechanisms. In this example, I’ll show you how to perform validation in a Spring MVC application using annotations and how to handle validation errors.
1. Set Up Your Project:
Create a new Spring MVC project or add the necessary dependencies to your existing project. You’ll need the following dependencies:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
2. Create a Model Class:
Create a Java class to represent the data you want to validate. For this example, let’s assume you’re creating a registration form, and you have a User
class:
public class User {
@NotEmpty(message = "Username is required")
private String username;
@Email(message = "Invalid email format")
private String email;
@Size(min = 6, message = "Password must be at least 6 characters long")
private String password;
// Getters and setters
}
In the User
class, we’ve used annotation-based validation from the javax.validation.constraints
package to specify validation rules.
3. Create a Controller:
Create a Spring MVC controller to handle form submissions and validation.
@Controller
public class RegistrationController {
@GetMapping("/register")
public String showRegistrationForm(Model model) {
model.addAttribute("user", new User());
return "registration-form";
}
@PostMapping("/register")
public String processRegistrationForm(
@Valid @ModelAttribute("user") User user,
BindingResult bindingResult) {
if (bindingResult.hasErrors()) {
return "registration-form";
}
// Save the user data or perform other actions here
return "registration-success";
}
}
In the processRegistrationForm
method, we use the @Valid
annotation to trigger validation based on the annotations defined in the User
class. We also include a BindingResult
parameter to capture validation errors.
4. Create a Thymeleaf Template:
Create an HTML form using Thymeleaf that corresponds to your model class and validation rules.
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Registration Form</title>
</head>
<body>
<h1>Registration Form</h1>
<form th:object="${user}" th:action="@{/register}" method="post">
<div>
<label for="username">Username:</label>
<input type="text" id="username" name="username" th:field="*{username}">
<span th:if="${#fields.hasErrors('username')}" th:errors="*{username}"></span>
</div>
<div>
<label for="email">Email:</label>
<input type="text" id="email" name="email" th:field="*{email}">
<span th:if="${#fields.hasErrors('email')}" th:errors="*{email}"></span>
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" name="password" th:field="*{password}">
<span th:if="${#fields.hasErrors('password')}" th:errors="*{password}"></span>
</div>
<div>
<button type="submit">Register</button>
</div>
</form>
</body>
</html>
In this Thymeleaf template, we use the th:field
attribute to bind form fields to the User
object, and we display validation error messages using th:errors
when validation fails.
5. Configure Validation Properties:
In your application.properties
or application.yml
file, configure the default message source for validation messages:
spring.messages.basename=messages
6. Create Validation Messages:
Create a messages.properties
file in your resources
directory to define custom validation error messages:
NotEmpty.user.username=Username is required
Email.user.email=Invalid email format
Size.user.password=Password must be at least {min} characters long
7. Run Your Application:
Run your Spring MVC application, and access the registration form at /register
. When you submit the form with validation errors, the error messages will be displayed. When the form is submitted successfully, it will redirect to a success page.
That’s it! You’ve implemented Spring MVC validation with annotation-based validation rules, error messages, and form binding. You can extend this example to include more complex validation logic and error handling as needed for your application.