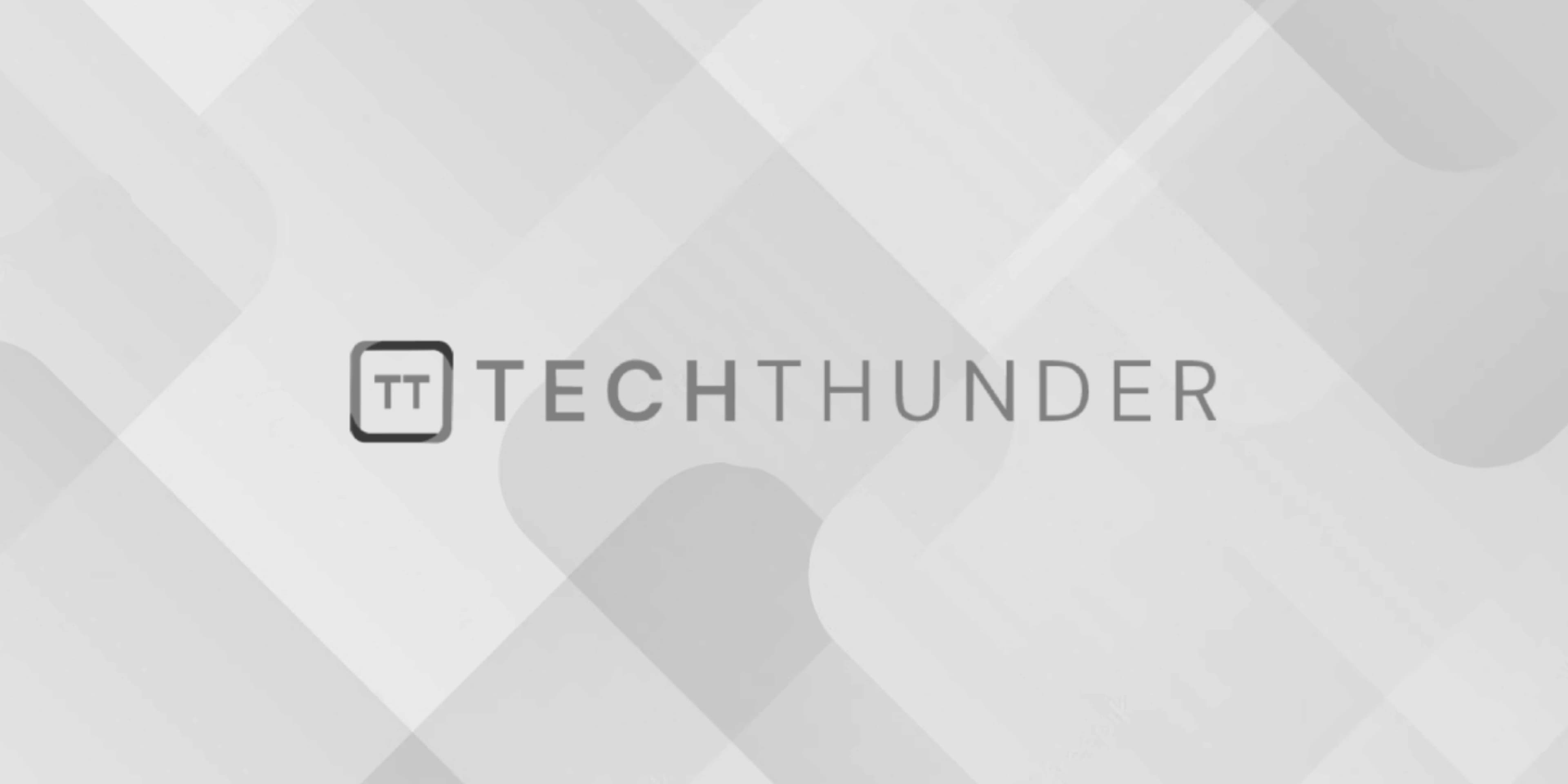
Spring Setter Injection with Map
The Spring, you can perform setter injection with a Map
to inject a collection of key-value pairs into a bean. Each entry in the Map
represents a key-value pair where the key is a unique identifier, and the value is an object or bean. Here’s how to perform setter injection with a Map
in Spring:
1. Create the Dependent Objects:
First, create the dependent objects or beans that you want to inject into another bean. For example, let’s create a Person
class:
public class Person {
private String name;
// Getter and setter methods for 'name'
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
2. Create the Target Bean:
Next, create the target bean that will depend on a Map
of Person
objects. The target bean should provide a setter method to receive the Map
. For example:
import java.util.Map;
public class Team {
private Map<String, Person> members;
// Setter method for injecting the 'members' map
public void setMembers(Map<String, Person> members) {
this.members = members;
}
public void printTeam() {
for (Map.Entry<String, Person> entry : members.entrySet()) {
System.out.println("Role: " + entry.getKey() + ", Name: " + entry.getValue().getName());
}
}
}
In this example, the Team
bean has a Map<String, Person>
property, and a setter method setMembers
is provided for setter injection.
3. Configure Spring Beans:
Configure the beans in your Spring configuration file (e.g., applicationContext.xml
) to define the Person
objects and the Team
bean. Configure the setter injection by specifying the <property>
element in the Team
bean definition. Use the <map>
element to define the map of Person
objects:
<bean id="person1" class="com.example.Person">
<property name="name" value="John" />
</bean>
<bean id="person2" class="com.example.Person">
<property name="name" value="Alice" />
</bean>
<bean id="team" class="com.example.Team">
<!-- Setter injection with a map -->
<property name="members">
<map>
<entry key="developer" value-ref="person1" />
<entry key="designer" value-ref="person2" />
</map>
</property>
</bean>
This XML configuration defines two Person
beans (“person1” and “person2”) and configures the Team
bean to inject a Map<String, Person>
where each entry represents a team member’s role (key) and the corresponding Person
bean reference (value).
4. Access the Target Bean:
Now, you can access the Team
bean, which has the map of Person
objects injected into it:
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Main {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
Team team = context.getBean(Team.class);
team.printTeam();
}
}
In this example, we retrieve the Team
bean from the Spring container and call its printTeam()
method to display the team members and their roles using the injected map.
Setter injection with a Map
allows you to inject a collection of key-value pairs into a bean, which is useful when you need to manage dependencies with named identifiers or roles in your Spring application.