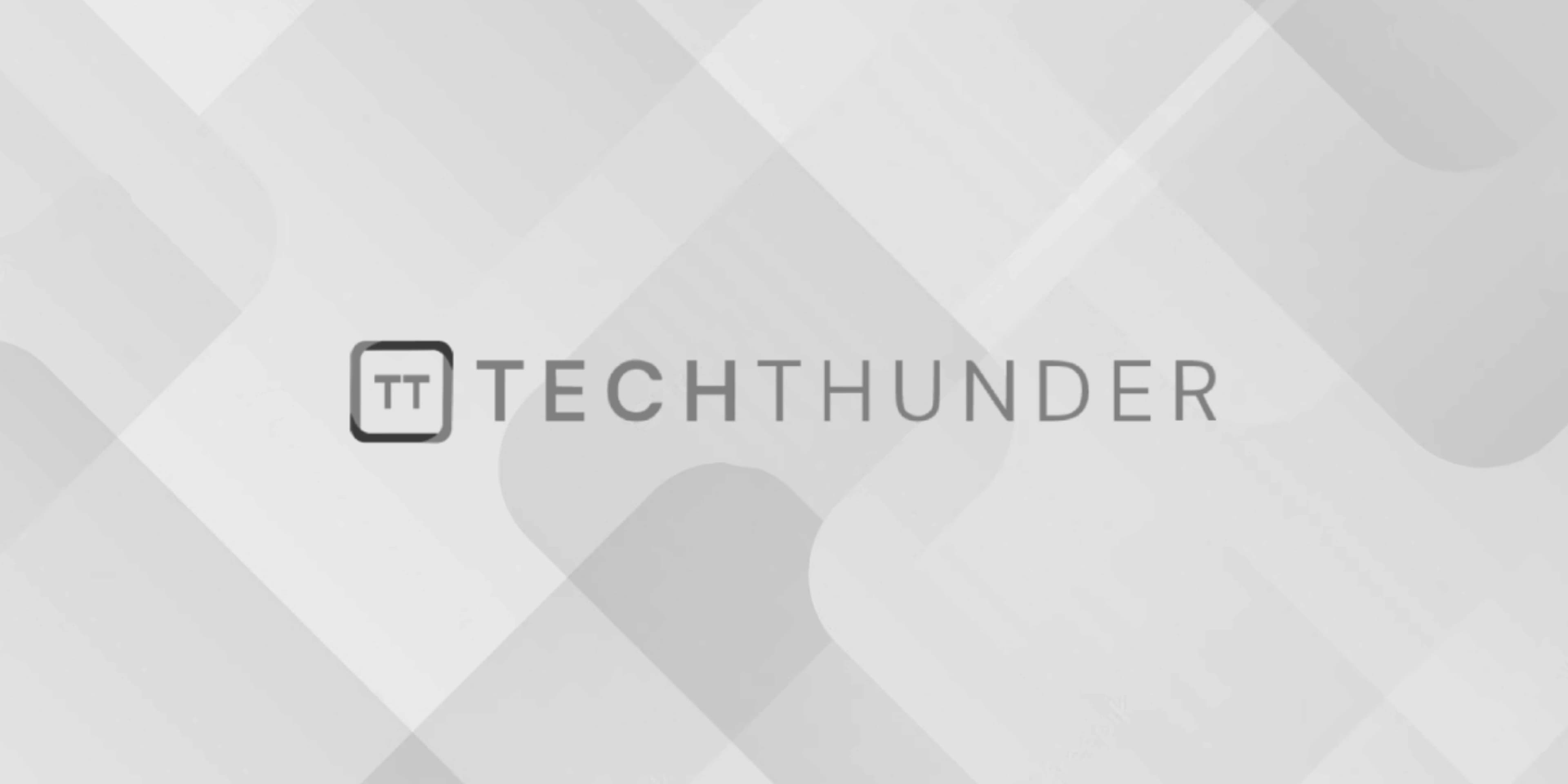
112 views
Spring AspectJ Annotation
AspectJ annotations are used in Spring AOP to define aspects and apply cross-cutting concerns to your Spring-managed beans. Spring AOP allows you to use AspectJ-style annotations to define aspects and apply them to specific methods or classes. AspectJ annotations provide a more concise and readable way to define aspects compared to traditional XML-based configuration. Here are some commonly used AspectJ annotations in Spring:
- @Aspect: This annotation is used to declare a class as an aspect. An aspect is a module that encapsulates cross-cutting concerns. It should be annotated with
@Aspect
.
@Aspect
public class LoggingAspect {
// Aspect code here
}
- @Before: This annotation is used to define advice that runs before a method execution. You can specify a pointcut expression to determine which methods should trigger this advice.
@Before("execution(* com.example.service.*.*(..))")
public void beforeAdvice() {
// Advice code before method execution
}
- @AfterReturning: This annotation is used to define advice that runs after a method returns a value successfully. You can specify a pointcut expression to determine which methods should trigger this advice.
@AfterReturning(pointcut = "execution(* com.example.service.*.*(..))", returning = "result")
public void afterReturningAdvice(Object result) {
// Advice code after method returns successfully
}
- @AfterThrowing: This annotation is used to define advice that runs after a method throws an exception. You can specify a pointcut expression to determine which methods should trigger this advice.
@AfterThrowing(pointcut = "execution(* com.example.service.*.*(..))", throwing = "ex")
public void afterThrowingAdvice(Exception ex) {
// Advice code after an exception is thrown
}
- @After: This annotation is used to define advice that runs after a method execution (whether it returns successfully or throws an exception). You can specify a pointcut expression to determine which methods should trigger this advice.
@After("execution(* com.example.service.*.*(..))")
public void afterAdvice() {
// Advice code after method execution (including exceptions)
}
- @Around: This annotation is used to define advice that can fully control the method’s execution. You can use
@Around
to wrap the target method and execute custom code before and after the method call.
@Around("execution(* com.example.service.*.*(..))")
public Object aroundAdvice(ProceedingJoinPoint joinPoint) throws Throwable {
// Advice code before method execution
Object result = joinPoint.proceed(); // Execute the target method
// Advice code after method execution
return result;
}
- @Pointcut: This annotation is used to define reusable pointcut expressions. You can create a method annotated with
@Pointcut
to define a pointcut and then refer to it in advice methods.
@Pointcut("execution(* com.example.service.*.*(..))")
public void myPointcut() {
// Empty method; serves as a pointcut definition
}
@Before("myPointcut()")
public void beforeAdvice() {
// Advice code before method execution
}
Using AspectJ annotations in Spring AOP provides a more modern and concise way to define aspects and apply them to your Spring beans. It allows you to easily separate cross-cutting concerns from your business logic and apply them selectively to specific methods or classes.