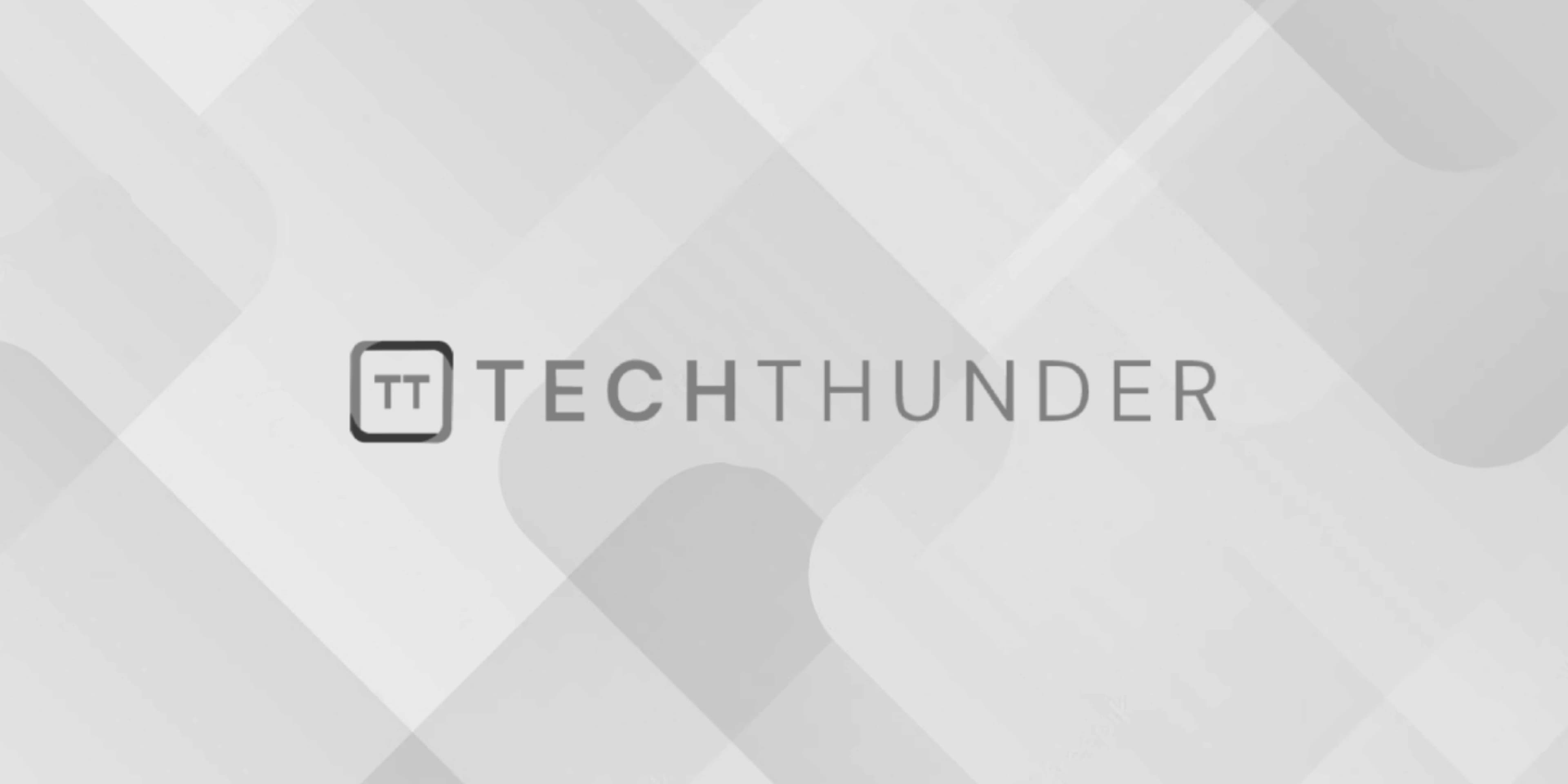
Spring Setter Injection Dependent Object
The Spring, you can perform setter injection to inject dependent objects or beans into another bean. Setter injection involves providing a setter method in the target bean and configuring the dependency through XML or Java-based configuration. Here’s a step-by-step guide on how to use setter injection to inject a dependent object into a Spring bean:
1. Create the Dependent Object:
First, create the dependent object or bean that you want to inject into another bean. This dependent object should have its own properties and behaviors. Here’s an example of a Person
class:
public class Person {
private String name;
// Getter and setter methods for the 'name' property
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
2. Create the Target Bean:
Next, create the target bean that will depend on the Person
object. The target bean should provide a setter method to receive the Person
object. For example:
public class GreetingService {
private Person person;
// Setter method for injecting the 'Person' object
public void setPerson(Person person) {
this.person = person;
}
public String greet() {
return "Hello, " + person.getName() + "!";
}
}
In this example, the GreetingService
bean has a Person
property, and a setter method setPerson
is provided for setter injection.
3. Configure Spring Beans:
Configure the beans in your Spring configuration file (e.g., applicationContext.xml
) to define the Person
and GreetingService
beans. Also, configure the setter injection by specifying the <property>
element in the GreetingService
bean definition:
<bean id="person" class="com.example.Person">
<property name="name" value="John" />
</bean>
<bean id="greetingService" class="com.example.GreetingService">
<!-- Setter injection -->
<property name="person" ref="person" />
</bean>
In this XML configuration, we define a Person
bean with the name “person” and a GreetingService
bean with the name “greetingService.” We use the <property>
element to specify the setter injection of the person
property in the GreetingService
bean.
4. Access the Target Bean:
Now, you can access the GreetingService
bean, which has the Person
object injected into it:
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Main {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
GreetingService greetingService = context.getBean(GreetingService.class);
System.out.println(greetingService.greet());
}
}
In this example, we retrieve the GreetingService
bean from the Spring container and call its greet()
method, which uses the injected Person
object to generate a greeting.
Setter injection allows you to inject dependent objects or beans into other beans, making your Spring application more modular and easier to manage. It also promotes loose coupling between components, as you can easily change the injected dependencies without modifying the target bean’s code.