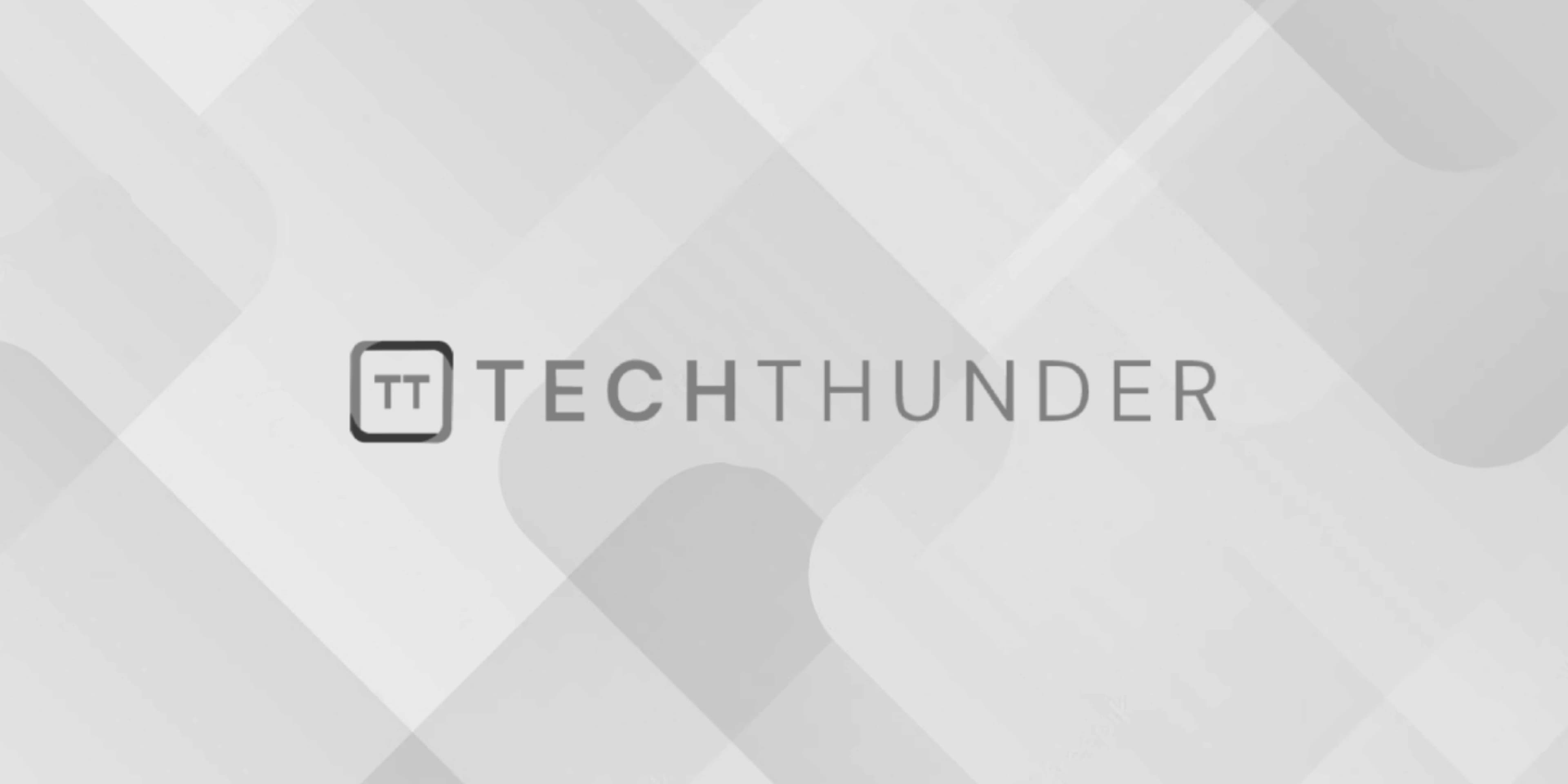
Spring Number Validation
Spring Number Validation ensures the accuracy and integrity of numeric data within Spring applications by enforcing specific constraints on numeric fields. This validation mechanism is crucial for preventing invalid inputs and maintaining data consistency. Spring provides a set of annotations that developers can use to define rules and constraints for numeric values, ensuring that they adhere to application-specific criteria. The primary annotations for number validation in Spring are:
- @Min: Specifies the minimum allowed value for a numeric field.
- @Max: Specifies the maximum allowed value for a numeric field.
- @DecimalMin: Specifies the minimum allowed decimal value for a numeric field.
- @DecimalMax: Specifies the maximum allowed decimal value for a numeric field.
Developers can apply these annotations to relevant fields or method parameters in their classes. During form submissions or API calls, Spring automatically validates the numeric inputs against the specified constraints. This process helps prevent incorrect or out-of-range numeric data from entering the system, contributing to the overall data quality and reliability of the application.
By incorporating Spring’s number validation, developers can streamline the validation process for numeric fields, ensuring that user inputs meet the necessary criteria. This not only enhances the user experience by providing immediate feedback on invalid inputs but also contributes to the robustness of the application by maintaining accurate and consistent data. Customizing these constraints allows developers to tailor the validation rules to the specific needs of their application, ensuring that numeric data is handled with precision and integrity throughout the development lifecycle.
Certainly! Here’s a simple example of Spring Number Validation using annotations in a Spring MVC application:
import javax.validation.constraints.Min;
import javax.validation.constraints.Max;
public class Product {
// Applying @Min and @Max annotations for number validation
@Min(value = 1, message = "Product ID must be at least 1")
@Max(value = 1000, message = "Product ID cannot exceed 1000")
private int productId;
// Other fields and methods...
// Getters and setters...
}
In this example, the Product
class has a field productId
that represents the ID of a product. The @Min
annotation specifies that the minimum allowed value for productId
is 1, and the @Max
annotation indicates that the maximum allowed value is 1000.
Now, let’s use this class in a Spring MVC controller:
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
@RequestMapping("/products")
public class ProductController {
@PostMapping("/add")
public String addProduct(@ModelAttribute("product") Product product, BindingResult bindingResult, Model model) {
// Validate the product using Spring's validation framework
// Errors will be stored in the bindingResult
if (bindingResult.hasErrors()) {
return "product-form";
}
// Process the product (save to database, etc.)
return "redirect:/products/list";
}
// Other methods...
}
In this controller, the @PostMapping("/add")
method receives the Product
object submitted from a form. Spring’s validation framework automatically applies the number validation constraints specified in the Product
class. If validation fails, errors are captured in the bindingResult
, allowing for appropriate handling (e.g., returning to the form page with error messages).
This is a basic example, and in a real-world scenario, you would likely have more complex validation rules and may use additional annotations or custom validators based on your specific requirements.