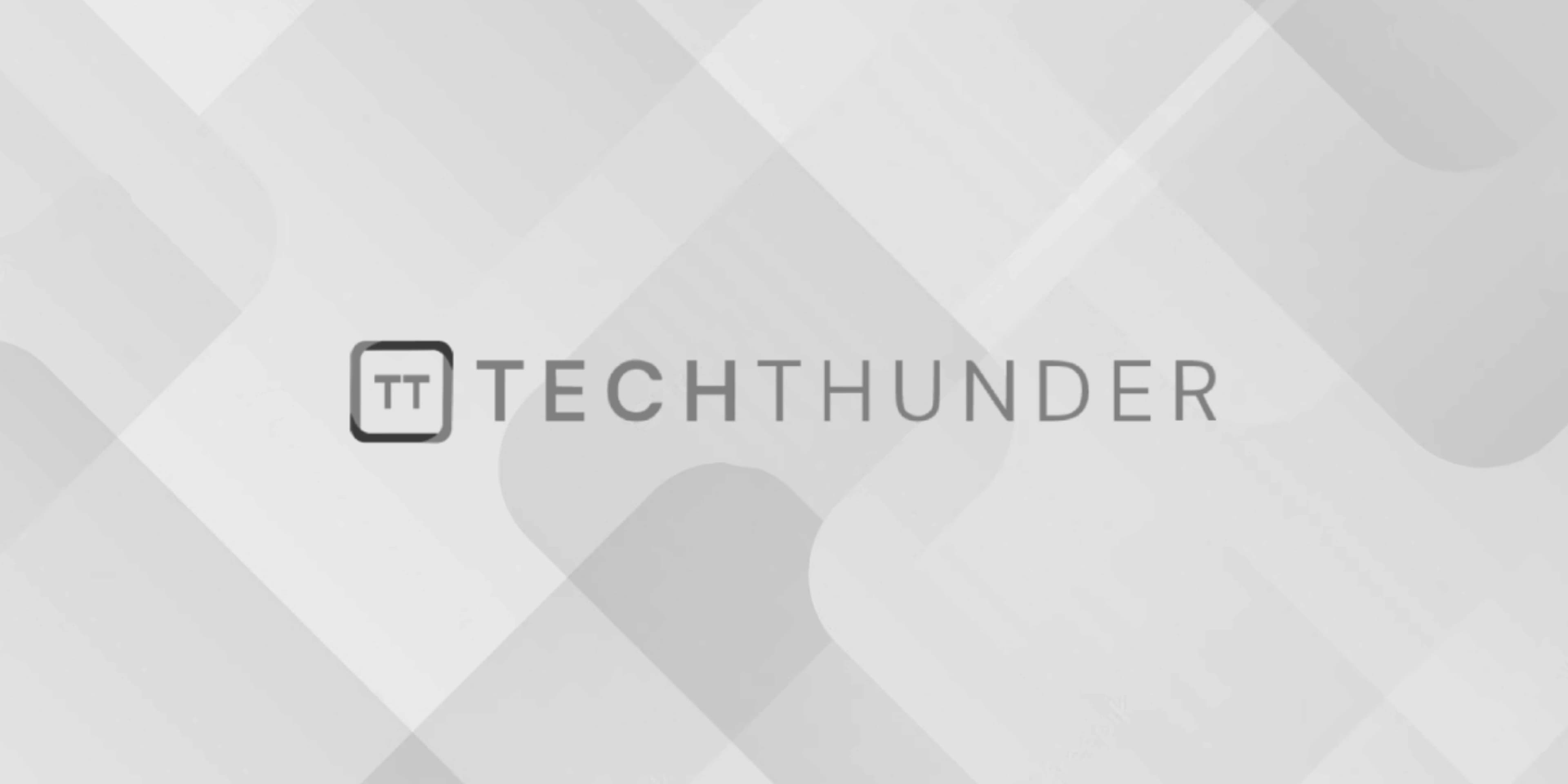
Spring AspectJ XML
The Spring AOP, you can configure aspects and pointcuts using XML configuration, often referred to as “XML-based AspectJ.” This approach allows you to define aspects and their associated advice using XML rather than annotations. Here’s how to configure AspectJ-style aspects in Spring using XML:
1. Create an Aspect Class:
First, create a regular Java class that defines your aspect. This class will contain the advice methods that you want to apply to specific join points in your application.
public class LoggingAspect {
public void beforeAdvice() {
// Advice code to be executed before a method call
System.out.println("Before method execution...");
}
}
2. Configure the Aspect in XML:
In your Spring XML configuration file (e.g., applicationContext.xml
), define the aspect using the <bean>
element with the id
attribute and specify the aspect class as well as the advice methods using the <property>
element. You’ll also need to configure the aspect as a bean using the <bean>
element.
<bean id="loggingAspect" class="com.example.LoggingAspect" />
<aop:config>
<aop:aspect id="myAspect" ref="loggingAspect">
<aop:before method="beforeAdvice" pointcut="execution(* com.example.*.*(..))" />
</aop:aspect>
</aop:config>
In this XML configuration:
- We define a bean with the ID “loggingAspect” for the
LoggingAspect
class. - Within the
<aop:config>
element, we declare an aspect with the ID “myAspect” and reference the “loggingAspect” bean using theref
attribute. - We configure a “before” advice that calls the “beforeAdvice” method of the “loggingAspect” when the specified pointcut expression is matched. In this case, the pointcut expression targets all methods in the
com.example
package.
3. Define the Target Bean:
Now, you can define the target bean on which the aspect will be applied.
<bean id="myService" class="com.example.MyService" />
4. Access the Target Bean:
You can access and use the target bean as usual in your application. The configured aspect will apply the advice specified in the XML configuration to the target bean’s methods based on the defined pointcut.
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
MyService myService = context.getBean("myService", MyService.class);
myService.doSomething(); // Advice will be applied before this method call
In this example, when the “doSomething” method of the “myService” bean is called, the “beforeAdvice” defined in the aspect will execute before the method call.
This XML-based AspectJ configuration allows you to define aspects and their advice in a separate XML file, providing a way to modularize cross-cutting concerns and apply them to specific join points in your application.