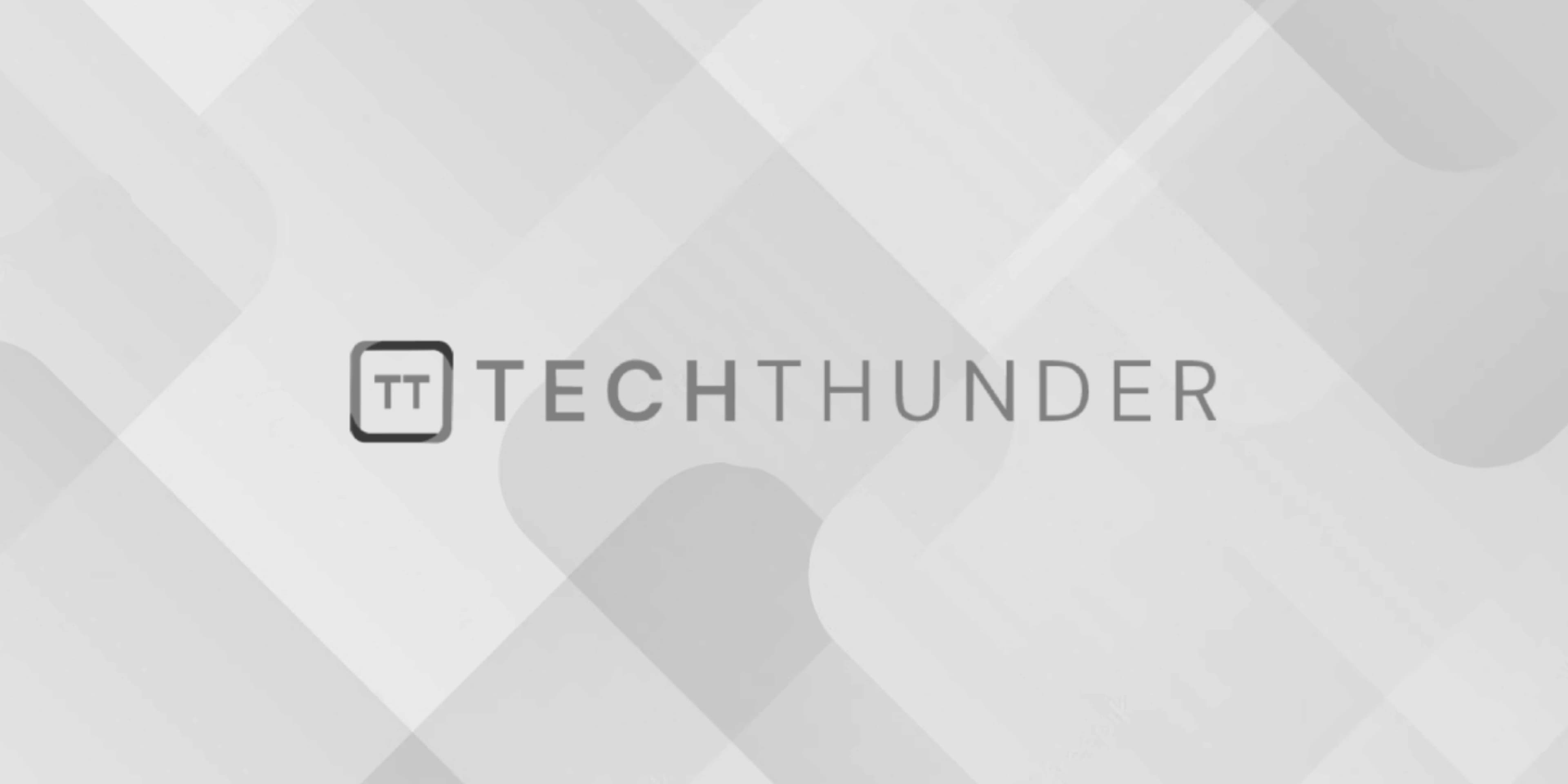
Spring JdbcTemplate Example
Spring JdbcTemplate is a part of the Spring JDBC framework that simplifies database access in Java applications. It provides a higher-level abstraction over plain JDBC, making it easier to work with databases. Here’s a simple example of how to use Spring JdbcTemplate to perform database operations.
1. Add Dependencies:
First, make sure you have the necessary dependencies in your project. If you are using Maven, include the following dependencies in your pom.xml
:
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.3.10</version> <!-- Use the appropriate version -->
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-tx</artifactId>
<version>5.3.10</version> <!-- Use the appropriate version -->
</dependency>
<!-- Add your database driver dependency (e.g., MySQL, PostgreSQL) -->
</dependencies>
2. Configure DataSource:
Configure a DataSource bean in your Spring configuration file (e.g., applicationContext.xml
):
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.cj.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/mydatabase" />
<property name="username" value="your_username" />
<property name="password" value="your_password" />
</bean>
3. Configure JdbcTemplate:
Configure a JdbcTemplate bean that uses the DataSource:
<bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<property name="dataSource" ref="dataSource" />
</bean>
4. Create a DAO Class:
Create a DAO (Data Access Object) class that uses the JdbcTemplate to perform database operations. For example:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Repository;
@Repository
public class UserDao {
private final JdbcTemplate jdbcTemplate;
@Autowired
public UserDao(JdbcTemplate jdbcTemplate) {
this.jdbcTemplate = jdbcTemplate;
}
public int getUserCount() {
String sql = "SELECT COUNT(*) FROM users";
return jdbcTemplate.queryForObject(sql, Integer.class);
}
// Other database operations go here
}
5. Use the DAO in Your Application:
You can now use the UserDao in your application:
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Main {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
UserDao userDao = context.getBean(UserDao.class);
int userCount = userDao.getUserCount();
System.out.println("User Count: " + userCount);
}
}
In this example, we’ve created a simple UserDao class that uses the JdbcTemplate to query the database and retrieve the user count. You can add more methods to perform other database operations as needed.
This is a basic example of how to use Spring JdbcTemplate to interact with a database in a Spring application. You can expand upon this foundation to build more complex database access functionality for your application.