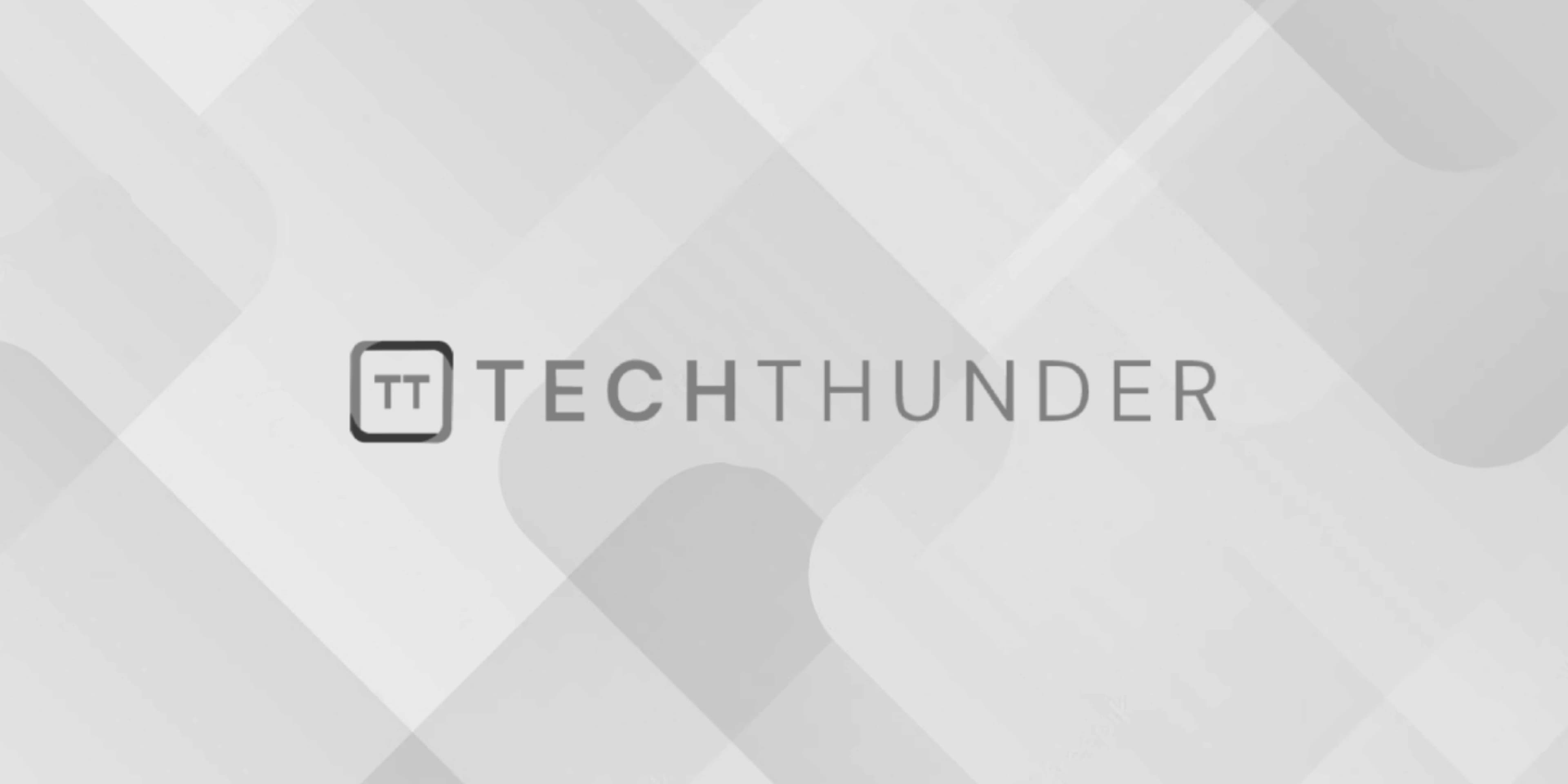
Spring ResultSetExtractor
The Spring JDBC, a ResultSetExtractor
is an interface that allows you to process the results of a JDBC query and extract data from the ResultSet
in a custom way. It provides more flexibility than the standard RowMapper
interface, as it enables you to perform more complex operations when dealing with query results. Here’s how you can use a ResultSetExtractor
in a Spring application:
1. Define a ResultSetExtractor
Implementation:
To use a ResultSetExtractor
, you need to implement the extractData(ResultSet rs)
method of the ResultSetExtractor
interface. This method is responsible for processing the ResultSet
and returning the desired result.
Here’s an example of a ResultSetExtractor
that extracts a list of user objects from a query result:
import org.springframework.dao.DataAccessException;
import org.springframework.jdbc.core.ResultSetExtractor;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
public class UserResultSetExtractor implements ResultSetExtractor<List<User>> {
@Override
public List<User> extractData(ResultSet rs) throws SQLException, DataAccessException {
List<User> users = new ArrayList<>();
while (rs.next()) {
User user = new User();
user.setId(rs.getLong("id"));
user.setUsername(rs.getString("username"));
user.setEmail(rs.getString("email"));
// Populate other fields as needed
users.add(user);
}
return users;
}
}
In this example, the UserResultSetExtractor
extracts user data from the ResultSet
and populates a list of User
objects.
2. Use the ResultSetExtractor
with JdbcTemplate:
To use the ResultSetExtractor
, you can pass it as an argument to the query
method of the JdbcTemplate
when executing a query. Here’s an example:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public class UserDao {
private final JdbcTemplate jdbcTemplate;
@Autowired
public UserDao(JdbcTemplate jdbcTemplate) {
this.jdbcTemplate = jdbcTemplate;
}
public List<User> getAllUsers() {
String sql = "SELECT * FROM users";
return jdbcTemplate.query(sql, new UserResultSetExtractor());
}
// Other database operations go here
}
In this example, the UserDao
class uses the UserResultSetExtractor
when querying the database to retrieve a list of all users.
3. Use the ResultSetExtractor
in Your Application:
You can then use the UserDao
in your application to retrieve and process the results:
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Main {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
UserDao userDao = context.getBean(UserDao.class);
List<User> users = userDao.getAllUsers();
for (User user : users) {
System.out.println("User ID: " + user.getId());
System.out.println("Username: " + user.getUsername());
System.out.println("Email: " + user.getEmail());
}
}
}
In this example, the getAllUsers
method of the UserDao
returns a list of users using the UserResultSetExtractor
, and you can iterate over the list to process the user data.
Using a ResultSetExtractor
is beneficial when you need to perform more complex data extraction and processing from a ResultSet
. It allows you to encapsulate the extraction logic in a reusable component, making your code more maintainable and easier to understand.