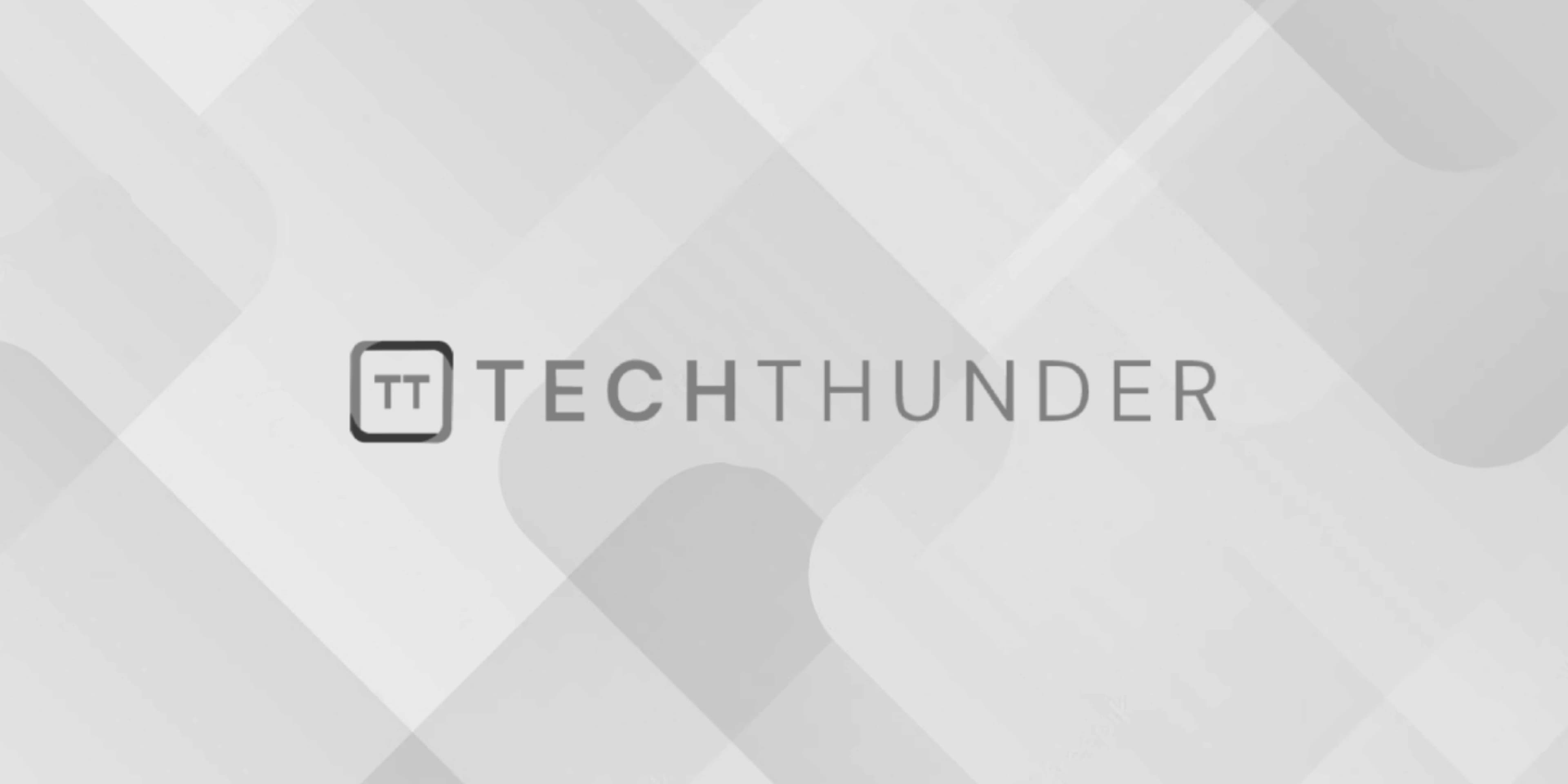
Spring MVC Tiles
Spring MVC Tiles integration allows you to create reusable and consistent layouts for your web application. Apache Tiles is a framework that provides a way to define templates (layouts) and use them across multiple pages in your web application. In this example, I’ll show you how to set up Spring MVC with Apache Tiles for creating layout templates.
1. Set Up Your Project:
Start by creating a Spring MVC project using Spring Boot or a standard Spring MVC project. Ensure you have the necessary dependencies for Spring MVC and Apache Tiles.
Here’s an example of how to include Apache Tiles in your Maven pom.xml
:
<dependency>
<groupId>org.apache.tiles</groupId>
<artifactId>tiles-jsp</artifactId>
<version>3.0.8</version>
</dependency>
2. Configure Apache Tiles:
Create a tiles.xml
configuration file in your project’s resources directory. This file defines your layout templates and their respective fragments.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE tiles-definitions PUBLIC
"-//Apache Software Foundation//DTD Tiles Configuration 3.0//EN"
"http://tiles.apache.org/dtds/tiles-config_3_0.dtd">
<tiles-definitions>
<definition name="base" template="/WEB-INF/views/layout/base.jsp">
<put-attribute name="title" value="" />
<put-attribute name="header" value="/WEB-INF/views/layout/header.jsp" />
<put-attribute name="footer" value="/WEB-INF/views/layout/footer.jsp" />
</definition>
<definition name="home" extends="base">
<put-attribute name="title" value="Home Page" />
<put-attribute name="body" value="/WEB-INF/views/home.jsp" />
</definition>
<!-- Define other templates as needed -->
</tiles-definitions>
In this example, we’ve defined a base template that includes header and footer fragments and a specific home
template that extends the base template and includes the home.jsp
as the body content.
3. Create Layout Templates:
Create your layout templates as JSP files in the /WEB-INF/views/layout/
directory. For example, base.jsp
, header.jsp
, and footer.jsp
.
4. Create Content Pages:
Create your content JSP pages that will be included in the layout templates. For example, home.jsp
.
5. Configure Tiles in Spring MVC:
In your Spring configuration (Java or XML-based), configure the Tiles View Resolver and TilesConfigurer.
Java-based configuration example:
@Configuration
public class TilesConfig extends WebMvcConfigurerAdapter {
@Bean
public TilesConfigurer tilesConfigurer() {
TilesConfigurer tilesConfigurer = new TilesConfigurer();
tilesConfigurer.setDefinitions(new String[] { "/WEB-INF/tiles/tiles.xml" });
tilesConfigurer.setCheckRefresh(true);
return tilesConfigurer;
}
@Bean
public ViewResolver viewResolver() {
TilesViewResolver resolver = new TilesViewResolver();
return resolver;
}
}
6. Create Spring Controllers:
Create Spring controllers for your application. These controllers will return the logical view names defined in the Tiles configuration.
@Controller
public class HomeController {
@GetMapping("/home")
public String home() {
return "home"; // Matches the definition name in tiles.xml
}
// Define other controllers as needed
}
7. Create Navigation Links:
Create navigation links in your JSP pages that correspond to the controller mappings. For example, in home.jsp
:
<a href="<c:url value="/home" />">Home</a>
8. Run Your Application:
Run your Spring MVC application and access the URLs defined in your controllers (e.g., /home
). You should see your layout templates applied to your content pages.
That’s it! You’ve set up Spring MVC with Apache Tiles for creating layout templates and achieving a consistent look and feel across your web application. You can create additional templates and pages as needed, and extend the layout templates to suit your application’s requirements.