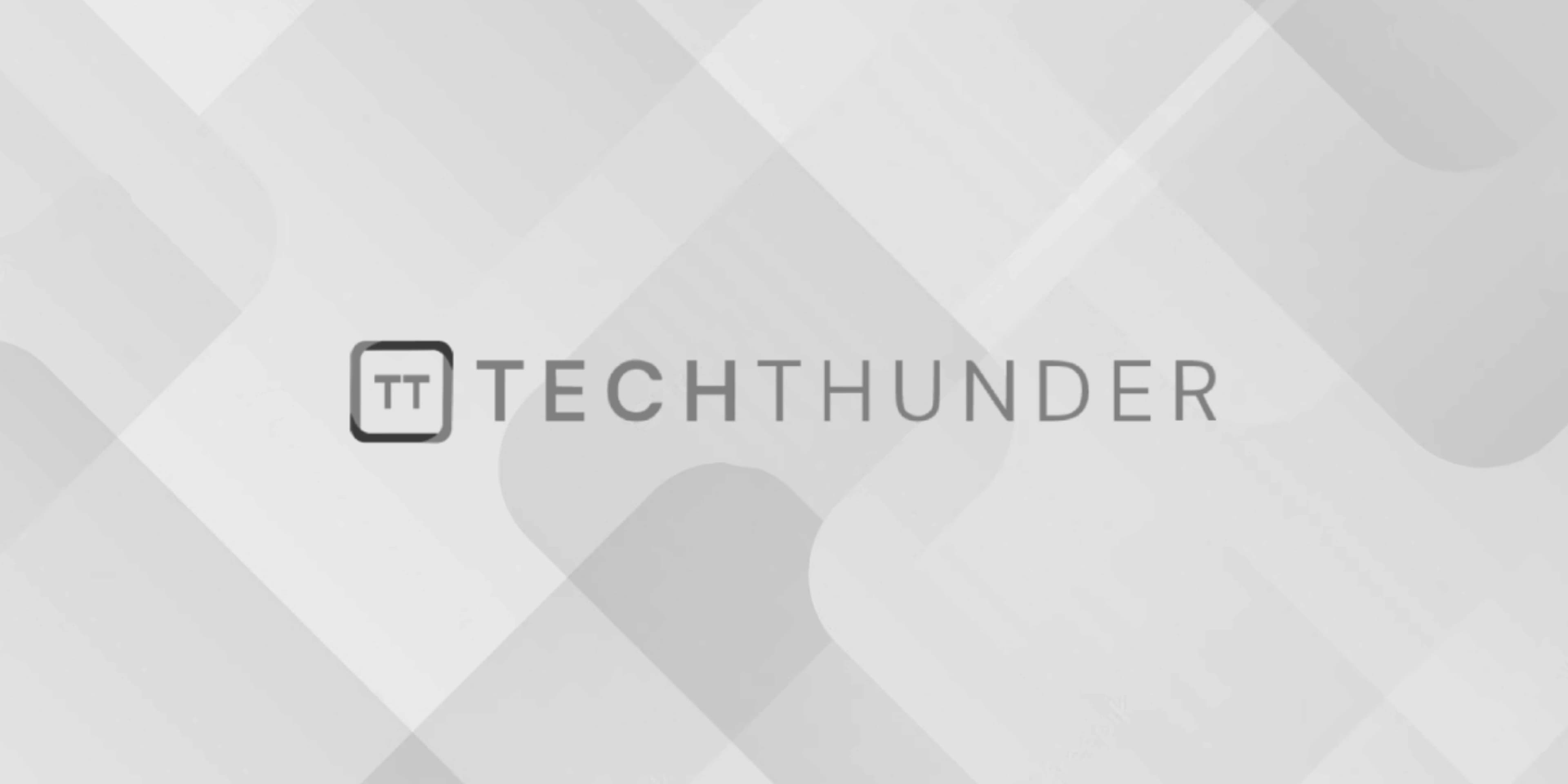
Spring with Struts2
Integrating Spring with Struts 2 is a common practice to combine the power of the Spring framework for dependency injection and Struts 2 for web application development. Here’s how you can integrate Spring with Struts 2 in your web application:
1. Set Up Your Project:
Create a new web application project using a Java EE or Servlet-based web framework. You can use Maven or Gradle for project management, or set up a web project manually.
2. Add Dependencies:
Include the necessary dependencies in your project. You’ll need the Spring Framework and Struts 2 libraries. Here’s a sample Maven dependency section for your pom.xml
:
<dependencies>
<!-- Spring Framework -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>5.3.11</version> <!-- Use the latest version available -->
</dependency>
<!-- Struts 2 -->
<dependency>
<groupId>org.apache.struts</groupId>
<artifactId>struts2-core</artifactId>
<version>2.5.26</version> <!-- Use the latest version available -->
</dependency>
</dependencies>
3. Configure the Spring Context:
Create a Spring configuration file (e.g., spring-config.xml
) and define your Spring beans, including the beans you want to inject into your Struts 2 actions. Here’s a simple example:
<!-- Define a simple Spring bean -->
<bean id="messageService" class="com.example.MessageService">
<property name="message" value="Hello, Spring!"/>
</bean>
4. Configure Struts 2:
Create a Struts 2 configuration file (e.g., struts.xml
) in your web application’s src
directory. Configure your Struts actions and results in this file. Here’s an example:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.5//EN"
"http://struts.apache.org/dtds/struts-2.5.dtd">
<struts>
<!-- Define a Struts action -->
<package name="default" namespace="/" extends="struts-default">
<action name="hello" class="com.example.HelloAction">
<result name="success">/hello.jsp</result>
</action>
</package>
</struts>
5. Create Struts 2 Action with Spring Injection:
Create a Struts 2 action class (e.g., HelloAction
) that you want to inject with Spring beans. Use Spring annotations for dependency injection.
import com.opensymphony.xwork2.ActionSupport;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
@Controller
public class HelloAction extends ActionSupport {
private MessageService messageService;
@Autowired
public void setMessageService(MessageService messageService) {
this.messageService = messageService;
}
public String execute() {
return SUCCESS;
}
public String getMessage() {
return messageService.getMessage();
}
}
In this example, we use Spring’s @Autowired
annotation to inject the MessageService
into the HelloAction
.
6. Create a JSP Page:
Create a JSP page (e.g., hello.jsp
) to display the results. You can access the injected Spring bean in the JSP page using Struts 2 tags.
<!DOCTYPE html>
<html>
<head>
<title>Hello World</title>
</head>
<body>
<h1>${message}</h1>
</body>
</html>
7. Configure Spring and Struts 2 Integration:
In your web.xml
, configure the ContextLoaderListener
to load the Spring application context:
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/spring-config.xml</param-value>
</context-param>
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
Also, configure the Struts 2 filter:
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.filter.StrutsPrepareAndExecuteFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
8. Run Your Application:
With everything configured, you can now deploy and run your Spring and Struts 2 web application. Access the hello
action (e.g., http://localhost:8080/your-app/hello
) to see the result.
This integration allows you to leverage Spring’s powerful features, such as dependency injection and AOP, in your Struts 2 web application, making it more maintainable and easier to test.