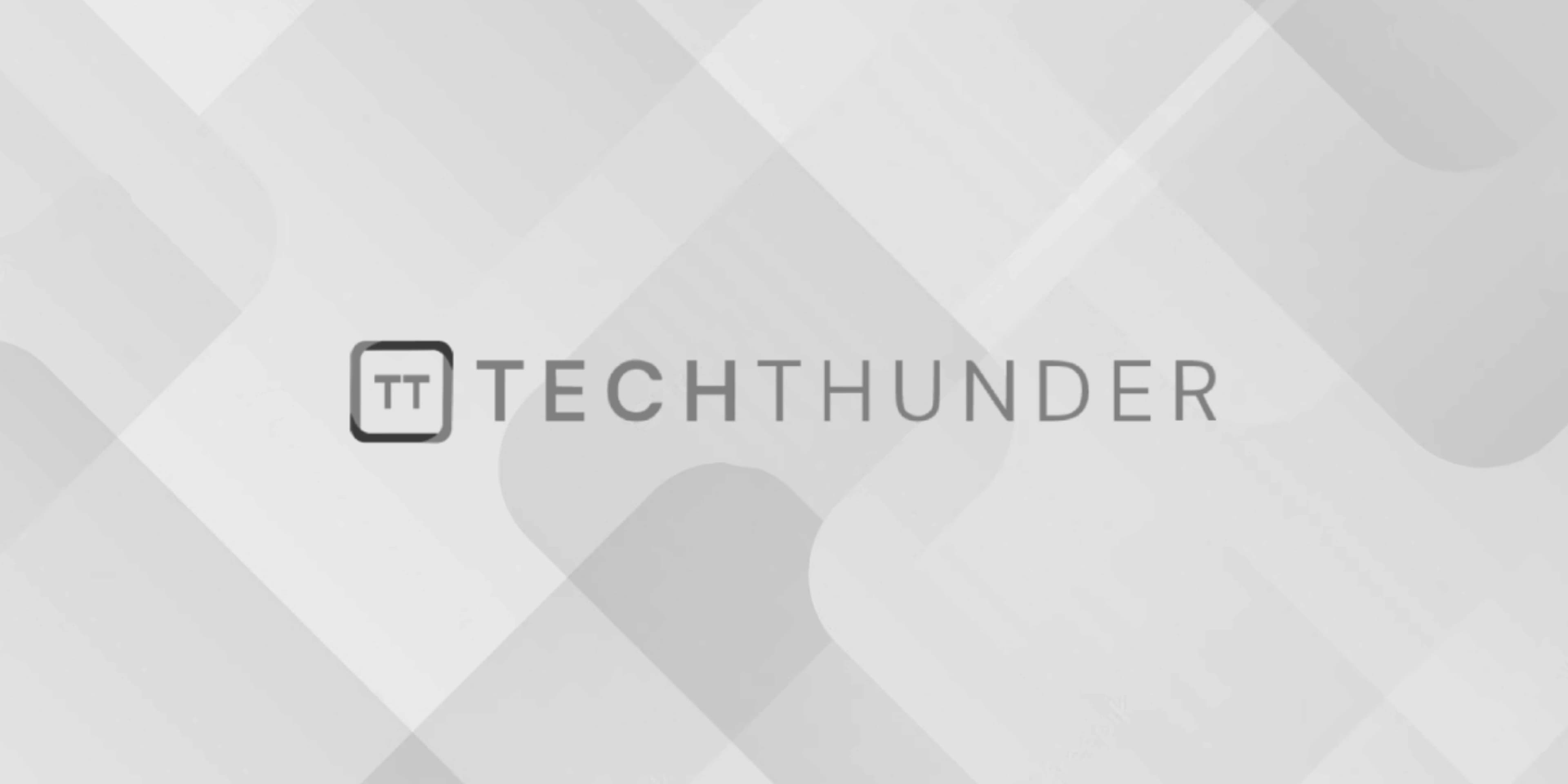
Spring Security XML Example
While Spring Security is often configured using Java-based configuration, you can also configure it using XML-based configuration. Here’s an example of configuring Spring Security using XML. In this example, we’ll set up a basic Spring Security configuration for form-based authentication.
1. Create a Spring Boot Project:
Start by creating a new Spring Boot project using Spring Initializr or your preferred development environment.
2. Configure Spring Security using XML:
Create a security.xml
file in the src/main/resources
directory. This file will contain your Spring Security configuration in XML format.
src/main/resources/security.xml
:
<?xml version="1.0" encoding="UTF-8"?>
<beans:beans xmlns="http://www.springframework.org/schema/security"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:beans="http://www.springframework.org/schema/beans"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/security
http://www.springframework.org/schema/security/spring-security.xsd">
<http auto-config="true">
<intercept-url pattern="/home" access="permitAll"/>
<intercept-url pattern="/admin" access="hasRole('ROLE_ADMIN')"/>
<form-login login-page="/login" default-target-url="/home"/>
<logout logout-success-url="/login?logout"/>
</http>
<authentication-manager>
<authentication-provider>
<user-service>
<user name="user" password="{noop}password" authorities="ROLE_USER"/>
<user name="admin" password="{noop}adminpassword" authorities="ROLE_ADMIN"/>
</user-service>
</authentication-provider>
</authentication-manager>
</beans:beans>
3. Create Controllers and Templates:
Create the necessary controllers for handling login, logout, and different pages.
src/main/java/com/example/demo/controller/LoginController.java
:
@Controller
public class LoginController {
@GetMapping("/login")
public String login() {
return "login";
}
}
src/main/java/com/example/demo/controller/HomeController.java
:
@Controller
public class HomeController {
@GetMapping("/home")
public String home() {
return "home";
}
}
src/main/java/com/example/demo/controller/AdminController.java
:
@Controller
public class AdminController {
@GetMapping("/admin")
public String admin() {
return "admin";
}
}
src/main/resources/templates/login.html
:
<!DOCTYPE html>
<html>
<head>
<title>Login Page</title>
</head>
<body>
<h2>Login Page</h2>
<form th:action="@{/login}" method="post">
<div>
<label for="username">Username:</label>
<input type="text" id="username" name="username">
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" name="password">
</div>
<button type="submit">Login</button>
</form>
</body>
</html>
src/main/resources/templates/home.html
:
<!DOCTYPE html>
<html>
<head>
<title>Home Page</title>
</head>
<body>
<h2>Welcome to the Home Page</h2>
<p>You are logged in as a user.</p>
<a href="/logout">Logout</a>
</body>
</html>
src/main/resources/templates/admin.html
:
<!DOCTYPE html>
<html>
<head>
<title>Admin Page</title>
</head>
<body>
<h2>Welcome to the Admin Page</h2>
<p>You are logged in as an admin.</p>
<a href="/logout">Logout</a>
</body>
</html>
4. Run the Application:
Run your Spring Boot application and navigate to http://localhost:8080/login
to see the login page. You can log in with the provided user and admin credentials.
Please note that the {noop}
prefix in the passwords indicates that the passwords are stored in plain text for this example. In a real application, you should use a proper password encoder for security.
Remember that while XML-based configuration is still supported in Spring Security, modern Spring Boot applications often use Java-based configuration for its flexibility and improved readability.