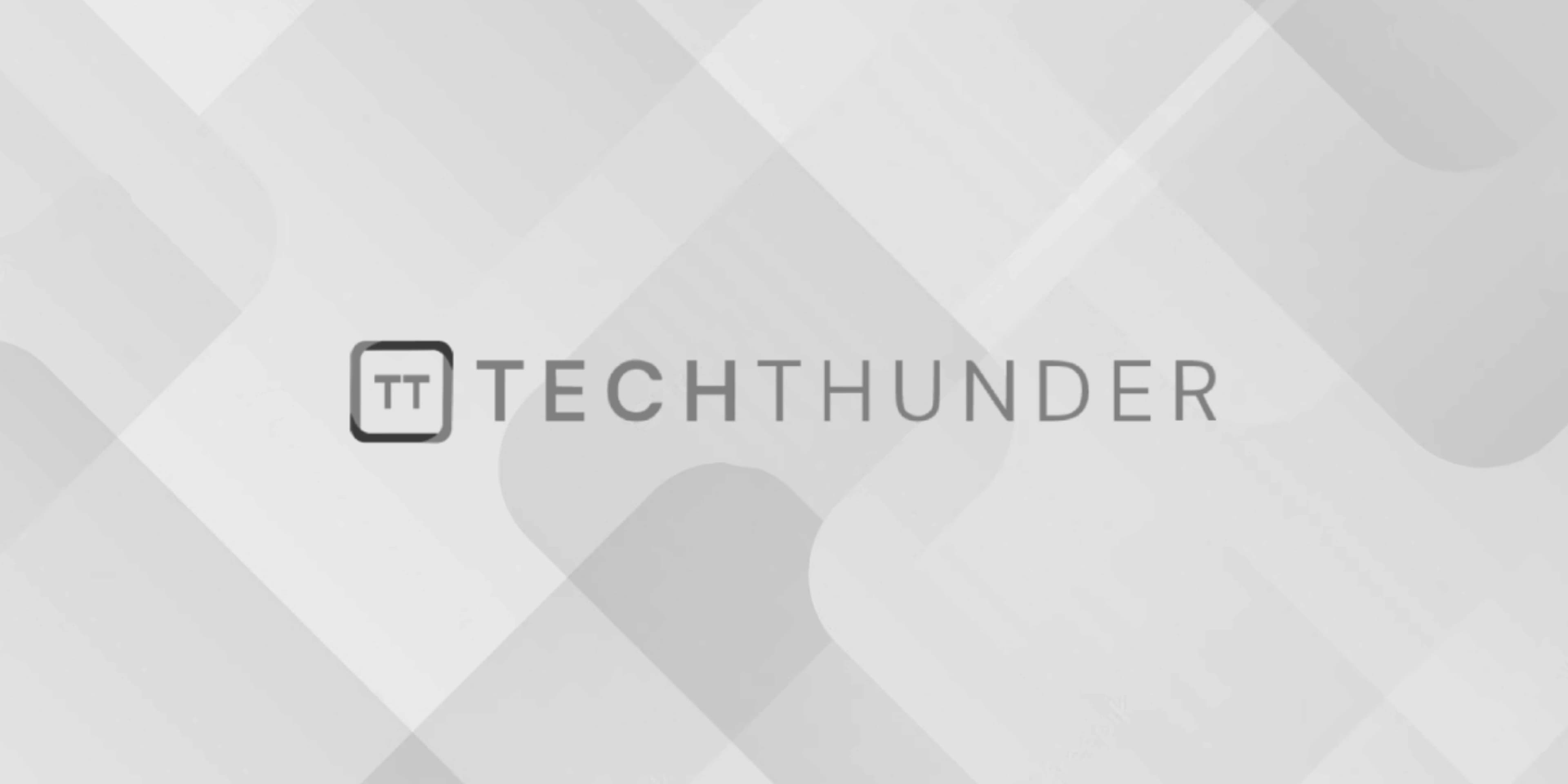
Spring Setter Injection with Map 2
The Spring, you can perform setter injection with a Map
using Java-based configuration. This approach allows you to define your Spring beans and their dependencies programmatically. Here’s how to perform setter injection with a Map
using Java-based configuration:
1. Create the Dependent Objects:
First, create the dependent objects or beans that you want to inject into another bean. For example, let’s create a Person
class:
public class Person {
private String name;
// Getter and setter methods for 'name'
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
2. Create the Configuration Class:
Next, create a Java configuration class that defines your Spring beans and their dependencies. In this example, we’ll define the Person
objects and the Team
bean, injecting a Map
of Person
objects:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import java.util.HashMap;
import java.util.Map;
@Configuration
public class AppConfig {
@Bean
public Person person1() {
Person person = new Person();
person.setName("John");
return person;
}
@Bean
public Person person2() {
Person person = new Person();
person.setName("Alice");
return person;
}
@Bean
public Team team() {
Team team = new Team();
Map<String, Person> members = new HashMap<>();
members.put("developer", person1());
members.put("designer", person2());
team.setMembers(members);
return team;
}
}
In this configuration class, we define two Person
beans (“person1” and “person2”) and a Team
bean. We inject a Map
of Person
objects into the Team
bean’s members
property.
3. Access the Target Bean:
Now, you can access the Team
bean, which has the map of Person
objects injected into it:
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class Main {
public static void main(String[] args) {
ApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class);
Team team = context.getBean(Team.class);
team.printTeam();
}
}
In above example, we create an AnnotationConfigApplicationContext
and specify the AppConfig
class to load the Spring configuration. Then, we retrieve the Team
bean from the context and call its printTeam()
method to display the team members and their roles using the injected map.
Setter injection with a Map
using Java-based configuration provides a programmatic way to define Spring beans and their dependencies, making it flexible and easy to manage dependencies in your Spring application.