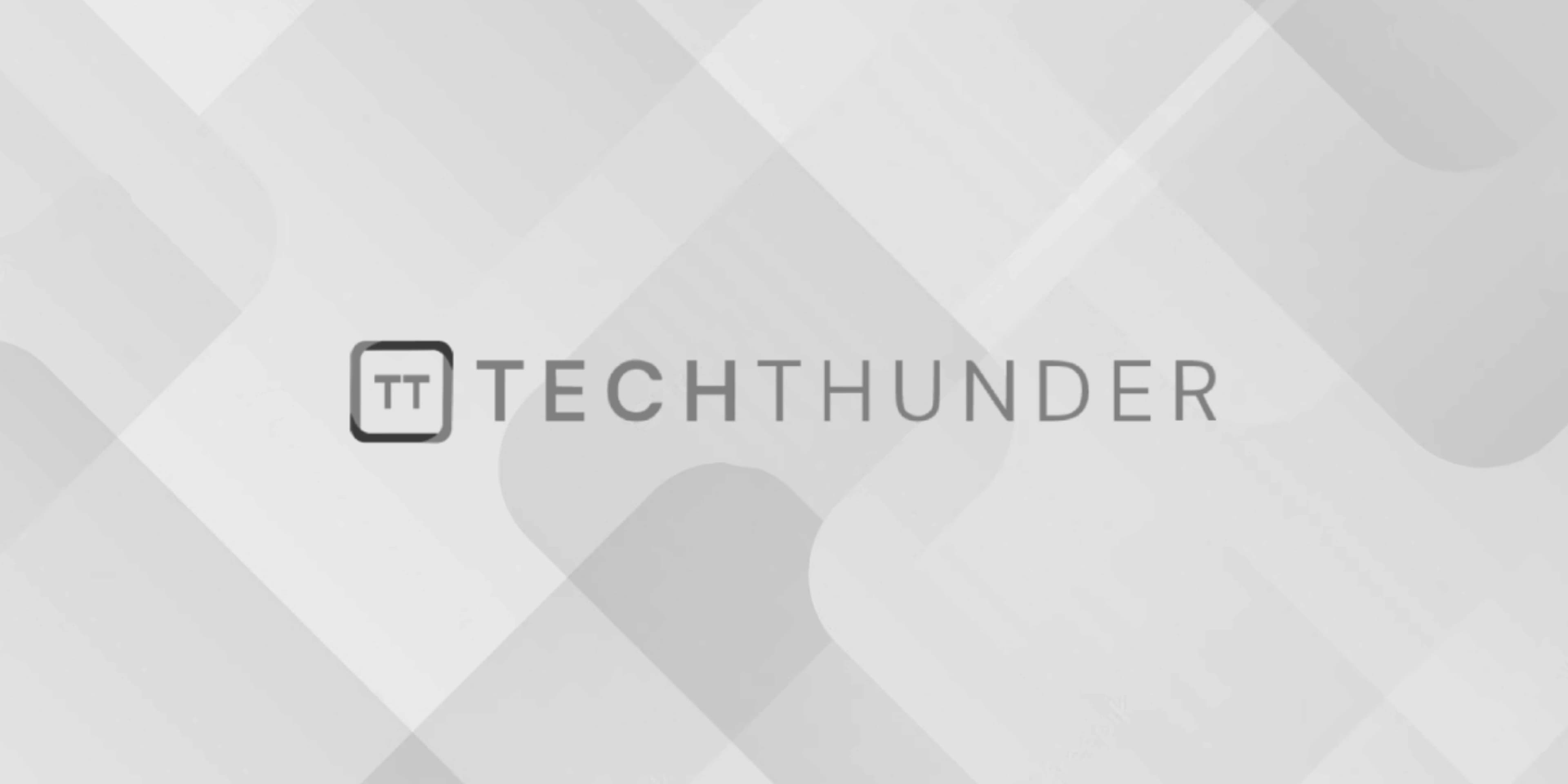
Spring with JPA
Integrating Spring with JPA (Java Persistence API) is a common practice for building Java web applications that need to interact with relational databases. JPA is a standard specification for ORM (Object-Relational Mapping) in Java, and Spring provides a convenient way to integrate and manage JPA-based database operations. Here’s a step-by-step guide on how to integrate Spring with JPA:
1. Set Up Your Development Environment:
Ensure you have the necessary development tools and libraries installed, including Java, Spring, and your JPA provider (e.g., Hibernate, EclipseLink, or OpenJPA).
2. Configure DataSource:
Define a DataSource
bean in your Spring configuration file (e.g., applicationContext.xml
). This bean provides the database connection information.
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.cj.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/mydatabase" />
<property name="username" value="your_username" />
<property name="password" value="your_password" />
</bean>
3. Configure JPA EntityManagerFactory:
Define an EntityManagerFactory
bean to configure JPA. This bean is responsible for creating EntityManager
instances.
<bean id="entityManagerFactory" class="org.springframework.orm.jpa.LocalContainerEntityManagerFactoryBean">
<property name="dataSource" ref="dataSource" />
<property name="packagesToScan" value="com.example.model" />
<property name="jpaVendorAdapter">
<bean class="org.springframework.orm.jpa.vendor.HibernateJpaVendorAdapter">
<property name="showSql" value="true" />
<property name="databasePlatform" value="org.hibernate.dialect.MySQL5Dialect" />
</bean>
</property>
</bean>
Make sure to replace "com.example.model"
with the package where your JPA entity classes are located.
4. Create JPA Entity Classes:
Create Java classes that represent your database tables and annotate them with JPA annotations, such as @Entity
, @Table
, @Id
, @GeneratedValue
, and others.
@Entity
@Table(name = "user")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String email;
// Getters and setters
}
5. Configure Spring’s Transaction Management:
Enable Spring’s transaction management by adding @EnableTransactionManagement
to your Spring configuration class or XML configuration file.
@Configuration
@EnableTransactionManagement
@ComponentScan(basePackages = "com.example")
public class AppConfig {
// Other configuration settings
}
6. Use @Transactional
Annotation:
Use the @Transactional
annotation on service methods to specify transaction boundaries. This ensures that database operations are performed within a transaction.
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Transactional
public User getUserById(Long id) {
return userRepository.findById(id).orElse(null);
}
// Other service methods
}
7. Create Spring Configuration and Main Application:
Define a Spring configuration class and set up the application context. Create an instance of the Spring ApplicationContext
to bootstrap your application.
8. Run Your Spring Application:
Run your Spring application, and it will now use JPA for ORM operations.
With these steps, you have successfully integrated Spring with JPA. Your Spring application can now interact with a relational database using JPA for object-relational mapping.