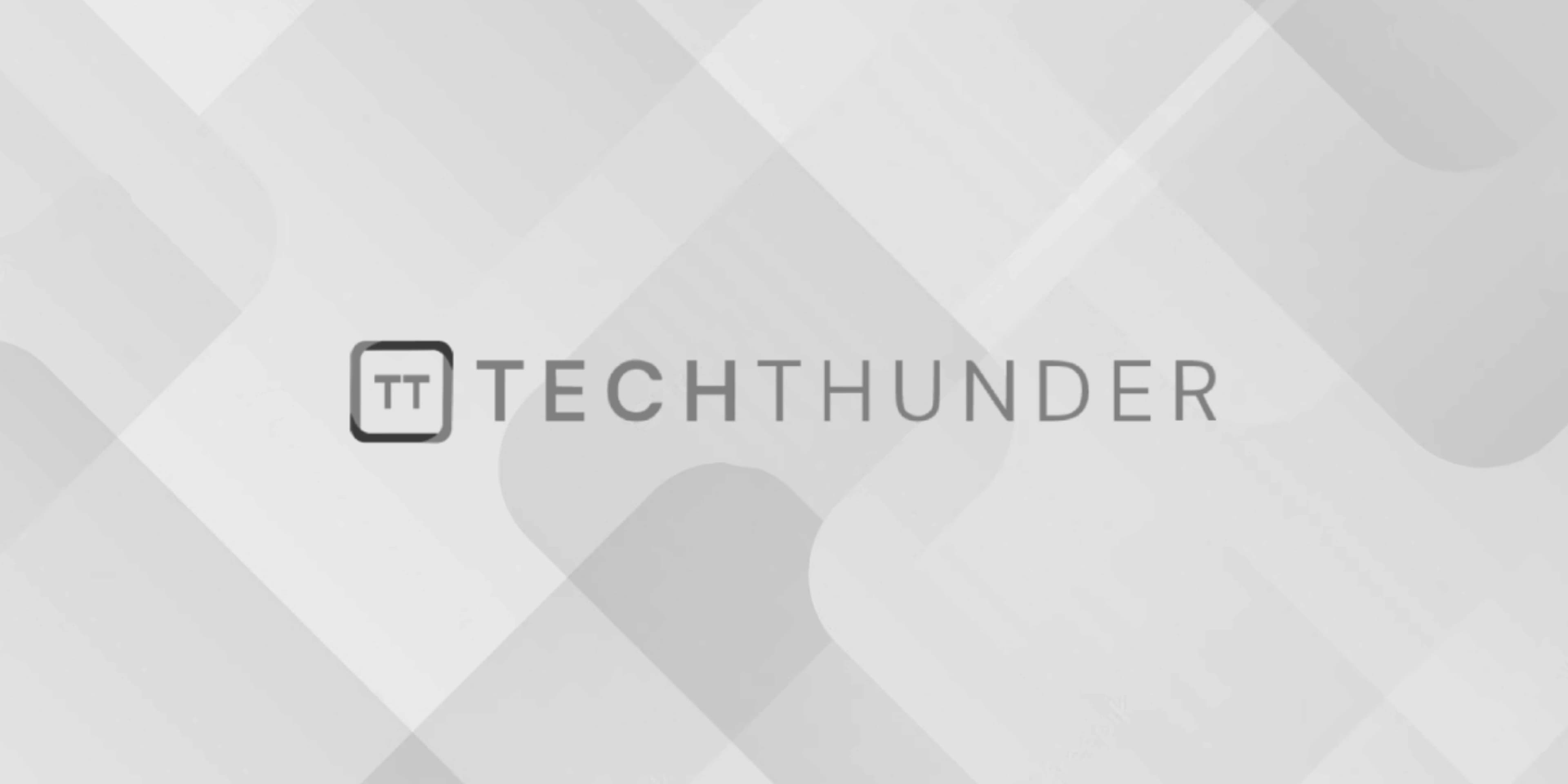
Spring Regular Expression Validation
The Spring, you can perform regular expression (regex) validation using the @Pattern
annotation. This annotation allows you to specify a regex pattern that a field in your model should match. If the input doesn’t match the specified pattern, Spring will consider it invalid. Here’s how to use regular expression validation in a Spring application:
1. Set Up Your Spring Project:
Create a Spring project using your preferred method (e.g., Spring Boot or a standard Spring MVC project).
2. Create a Model Class:
Create a Java class that represents the data you want to validate. For this example, let’s assume you have a Person
class with a field that should match a specific regex pattern.
public class Person {
@Pattern(regexp = "^\\d{3}-\\d{2}-\\d{4}$", message = "Invalid SSN format (XXX-XX-XXXX)")
private String ssn;
// Getters and setters
}
In the Person
class, we’ve used the @Pattern
annotation to specify a regular expression pattern that represents a Social Security Number (SSN) in the format XXX-XX-XXXX
.
3. Create a Controller:
Create a Spring MVC controller to handle form submissions and validation.
@Controller
public class PersonController {
@GetMapping("/person-form")
public String showPersonForm(Model model) {
model.addAttribute("person", new Person());
return "person-form";
}
@PostMapping("/submit-person")
public String submitPersonForm(@Valid @ModelAttribute("person") Person person, BindingResult bindingResult) {
if (bindingResult.hasErrors()) {
return "person-form";
}
// Process the valid input here (e.g., save to a database)
return "person-success";
}
}
In the submitPersonForm
method, we use the @Valid
annotation to trigger validation based on the annotations defined in the Person
class. We also include a BindingResult
parameter to capture validation errors.
4. Create a Thymeleaf Template:
Create an HTML form using Thymeleaf that corresponds to your model class and validation rules.
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Person Form</title>
</head>
<body>
<h1>Person Form</h1>
<form th:object="${person}" th:action="@{/submit-person}" method="post">
<div>
<label for="ssn">SSN:</label>
<input type="text" id="ssn" name="ssn" th:field="*{ssn}">
<span th:if="${#fields.hasErrors('ssn')}" th:errors="*{ssn}"></span>
</div>
<div>
<button type="submit">Submit</button>
</div>
</form>
</body>
</html>
In this Thymeleaf template, we use the th:field
attribute to bind the form field to the Person
object and display validation error messages using th:errors
when validation fails.
5. Run Your Application:
Run your Spring application, and access the person form at /person-form
. When you submit the form with an SSN that doesn’t match the specified regex pattern, you will see a validation error message. When you submit a valid SSN, it will redirect to a success page.
That’s how you can perform regular expression validation in a Spring application using the @Pattern
annotation. You can adjust the regex pattern and validation messages to fit your specific requirements.