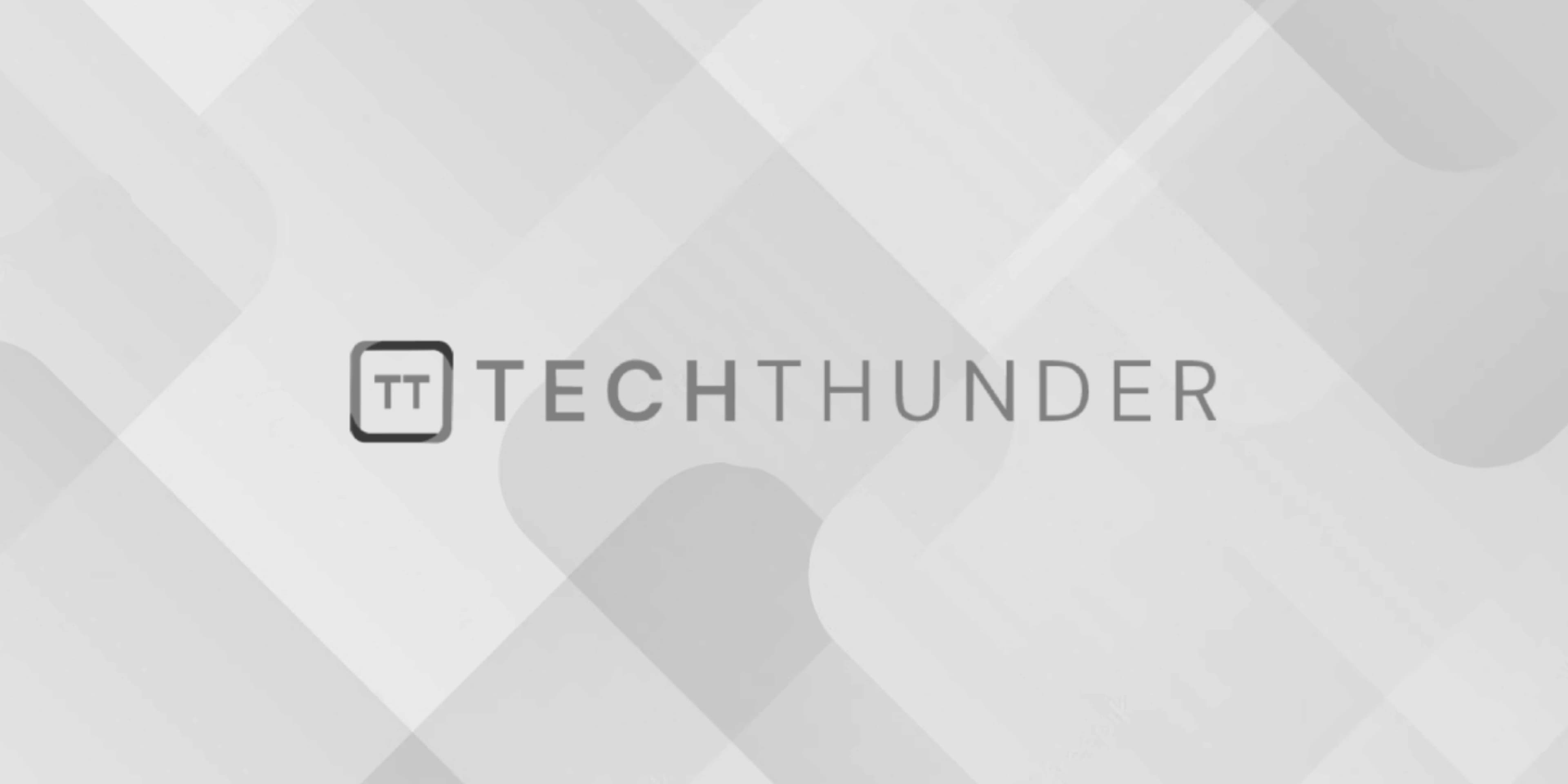
Spring Java Mail
Spring provides excellent support for sending emails using the JavaMail API. You can use Spring’s JavaMailSender
and related classes to simplify the process of sending emails in your Spring-based applications. Here’s how to use Spring with JavaMail for sending emails:
1. Add JavaMail Dependency:
First, make sure you have the JavaMail library as a dependency in your project. You can add it to your project’s build configuration (e.g., Maven or Gradle) as follows:
For Maven:
<dependency>
<groupId>javax.mail</groupId>
<artifactId>javax.mail-api</artifactId>
<version>1.6.2</version> <!-- Use the latest version available -->
</dependency>
2. Configure JavaMail in Spring:
In your Spring configuration file (e.g., applicationContext.xml
), configure the JavaMailSender
bean. You’ll also need to set up a JavaMailSenderImpl
bean, which is an implementation of the JavaMailSender
interface.
<bean id="mailSender" class="org.springframework.mail.javamail.JavaMailSenderImpl">
<property name="host" value="smtp.example.com"/>
<property name="port" value="587"/>
<property name="username" value="your-username"/>
<property name="password" value="your-password"/>
<property name="javaMailProperties">
<props>
<prop key="mail.smtp.auth">true</prop>
<prop key="mail.smtp.starttls.enable">true</prop>
</props>
</property>
</bean>
In this configuration:
- Set the SMTP server host, port, username, and password according to your email provider’s settings.
- Configure additional properties like authentication and TLS settings as needed.
3. Create and Send Emails:
Inject the JavaMailSender
bean into your Spring components or services where you need to send emails.
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.SimpleMailMessage;
public class EmailService {
private JavaMailSender mailSender;
public void setMailSender(JavaMailSender mailSender) {
this.mailSender = mailSender;
}
public void sendEmail(String to, String subject, String text) {
SimpleMailMessage message = new SimpleMailMessage();
message.setTo(to);
message.setSubject(subject);
message.setText(text);
mailSender.send(message);
}
}
4. Configure the Application Context:
Ensure that you have an application context XML file that includes the configuration for the JavaMailSender
bean and any other Spring beans that use JavaMail for sending emails.
5. Use JavaMailSender in Your Application:
Now you can use the EmailService
or any other component that utilizes JavaMailSender
for sending emails in your Spring application.
public class MainApp {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
EmailService emailService = (EmailService) context.getBean("emailService");
// Send an email
emailService.sendEmail("[email protected]", "Hello from Spring", "This is a test email from Spring.");
}
}
In this example, we create an EmailService
that sends an email using the JavaMailSender
. You can customize the email subject, recipient, and content as needed.
Spring’s integration with JavaMail simplifies the process of sending emails in your Spring-based applications, providing a consistent and easy-to-use API for email operations.