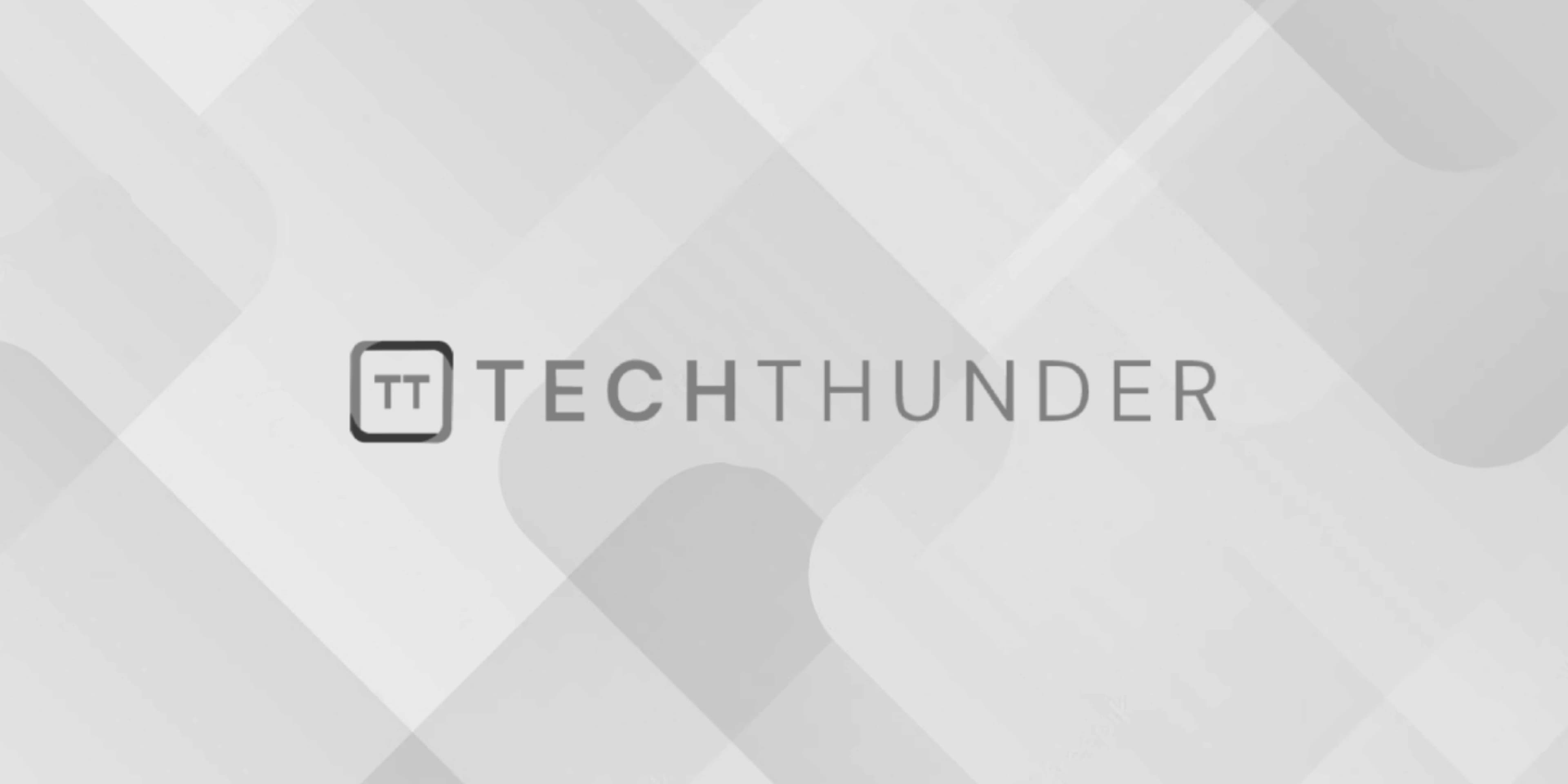
Spring Security Remember Me
The “Remember Me” functionality in Spring Security allows users to stay logged in even after they close their browser or log out. This is achieved by using a persistent token mechanism that allows the user to be automatically authenticated on subsequent visits to the website. Here’s how you can implement the “Remember Me” feature in a Spring Boot application using Spring Security:
1. Configure Spring Security:
In your Spring Security configuration class, you can enable the “Remember Me” functionality by using the rememberMe()
method.
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(HttpSecurity http) throws Exception {
http
// ... other configurations ...
.rememberMe()
.userDetailsService(userDetailsService)
.tokenValiditySeconds(86400) // Remember Me token valid for 24 hours
.key("mySecretKey"); // Key for hashing Remember Me tokens
}
// ... other configurations ...
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
In this example, we’re configuring Remember Me to use the UserDetailsService
to load the user details, setting the validity of the Remember Me token to 24 hours, and specifying a secret key for hashing the Remember Me tokens.
2. Create Login and Logout Controllers:
You can create login and logout controllers similar to the previous examples.
3. Create Remember Me Token Repository:
Spring Security requires a repository to store Remember Me tokens. You can configure this by creating a bean of type PersistentTokenRepository
.
import org.springframework.security.web.authentication.rememberme.PersistentTokenRepository;
import org.springframework.security.web.authentication.rememberme.JdbcTokenRepositoryImpl;
@Configuration
public class RememberMeConfig {
@Autowired
private DataSource dataSource;
@Bean
public PersistentTokenRepository persistentTokenRepository() {
JdbcTokenRepositoryImpl tokenRepository = new JdbcTokenRepositoryImpl();
tokenRepository.setDataSource(dataSource);
return tokenRepository;
}
}
4. Configure Remember Me Token Storage:
You need to include the PersistentTokenRepository
in your Spring Security configuration.
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Autowired
private PersistentTokenRepository tokenRepository;
@Override
protected void configure(HttpSecurity http) throws Exception {
http
// ... other configurations ...
.rememberMe()
.userDetailsService(userDetailsService)
.tokenValiditySeconds(86400)
.tokenRepository(tokenRepository)
.key("mySecretKey");
}
// ... other configurations ...
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
5. Create Templates and Controllers:
Create login and logout templates and controllers to handle the login and logout processes. These can be similar to the examples provided earlier.
6. Run the Application:
Run your Spring Boot application and test the “Remember Me” functionality. After logging in and checking the “Remember Me” checkbox, close your browser and reopen it. You should be automatically logged in.
Remember that the “Remember Me” feature involves security considerations, so it’s important to keep your application secure, including protecting the Remember Me key and managing persistent tokens securely.