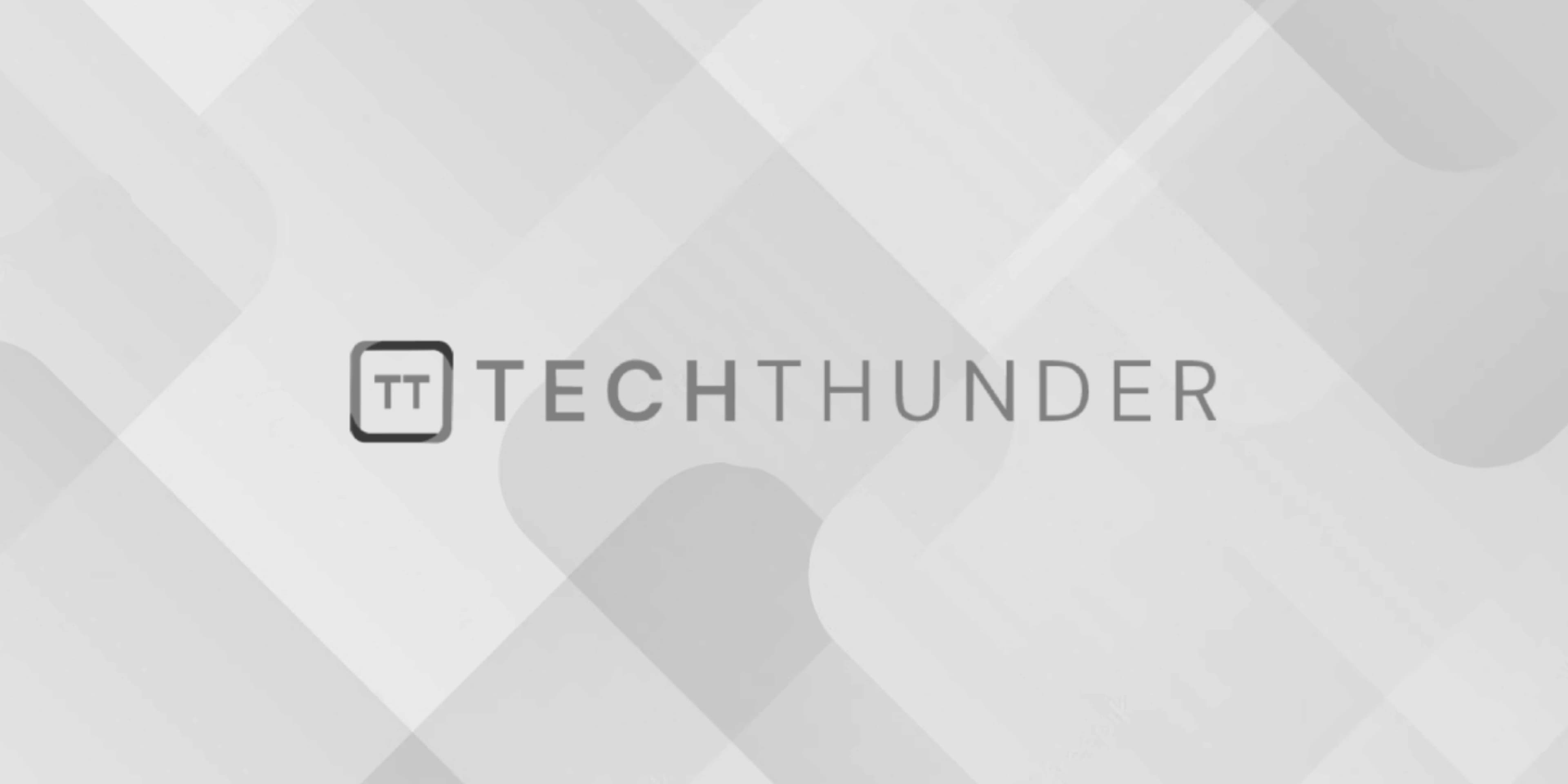
Spring RequestParam Annotation
The Spring MVC, the @RequestParam
annotation is used to extract values from request parameters in HTTP requests. It’s commonly used in Spring controllers to obtain values submitted by clients as part of a URL query string or as form parameters in POST requests. Here’s how to use the @RequestParam
annotation:
1. Basic Usage:
To use the @RequestParam
annotation, you typically add it as a parameter to your controller method. The annotation is used to bind the value of a request parameter to a method parameter. For example:
@Controller
public class MyController {
@GetMapping("/example")
public String example(@RequestParam("paramName") String paramValue, Model model) {
// Use the paramValue in your controller logic
model.addAttribute("message", "Received parameter value: " + paramValue);
return "example-view";
}
}
In this example, we’re using @RequestParam
to extract the value of a parameter named “paramName” from the incoming HTTP request’s query string.
2. Optional Parameters:
You can make request parameters optional by specifying the required
attribute of the @RequestParam
annotation. By default, required
is set to true
, which means that the parameter must be present in the request. If required
is set to false
, the parameter is considered optional.
@GetMapping("/optional")
public String optionalParam(@RequestParam(value = "paramName", required = false) String paramValue, Model model) {
if (paramValue != null) {
model.addAttribute("message", "Received parameter value: " + paramValue);
} else {
model.addAttribute("message", "Parameter not provided");
}
return "optional-view";
}
3. Default Values:
You can specify default values for request parameters using the defaultValue
attribute of the @RequestParam
annotation. If the parameter is not provided in the request, it will take on the specified default value.
@GetMapping("/default")
public String defaultParam(@RequestParam(value = "paramName", defaultValue = "Default Value") String paramValue, Model model) {
model.addAttribute("message", "Received parameter value: " + paramValue);
return "default-view";
}
4. Multi-Valued Parameters:
If you expect multiple values for a single parameter (e.g., when dealing with checkboxes or multi-select dropdowns), you can use an array or a List
as the parameter type. Spring will automatically bind multiple values to the array or list.
@GetMapping("/multi")
public String multiValuedParam(@RequestParam("paramName") List<String> paramValues, Model model) {
model.addAttribute("message", "Received parameter values: " + paramValues);
return "multi-view";
}
5. Mapping to Java Objects:
You can also use the @RequestParam
annotation to map request parameters to fields of Java objects. This is often used in conjunction with form submissions.
@PostMapping("/submit")
public String submitForm(@RequestParam("name") String name, @RequestParam("age") int age, Model model) {
// Create a User object from request parameters
User user = new User(name, age);
// Process the user object
// ...
model.addAttribute("user", user);
return "result-view";
}
In this example, the @RequestParam
annotation is used to map request parameters to fields of a User
object.
The @RequestParam
annotation is a powerful tool for handling request parameters in Spring MVC. It provides flexibility in extracting, validating, and using parameter values in your controller methods.