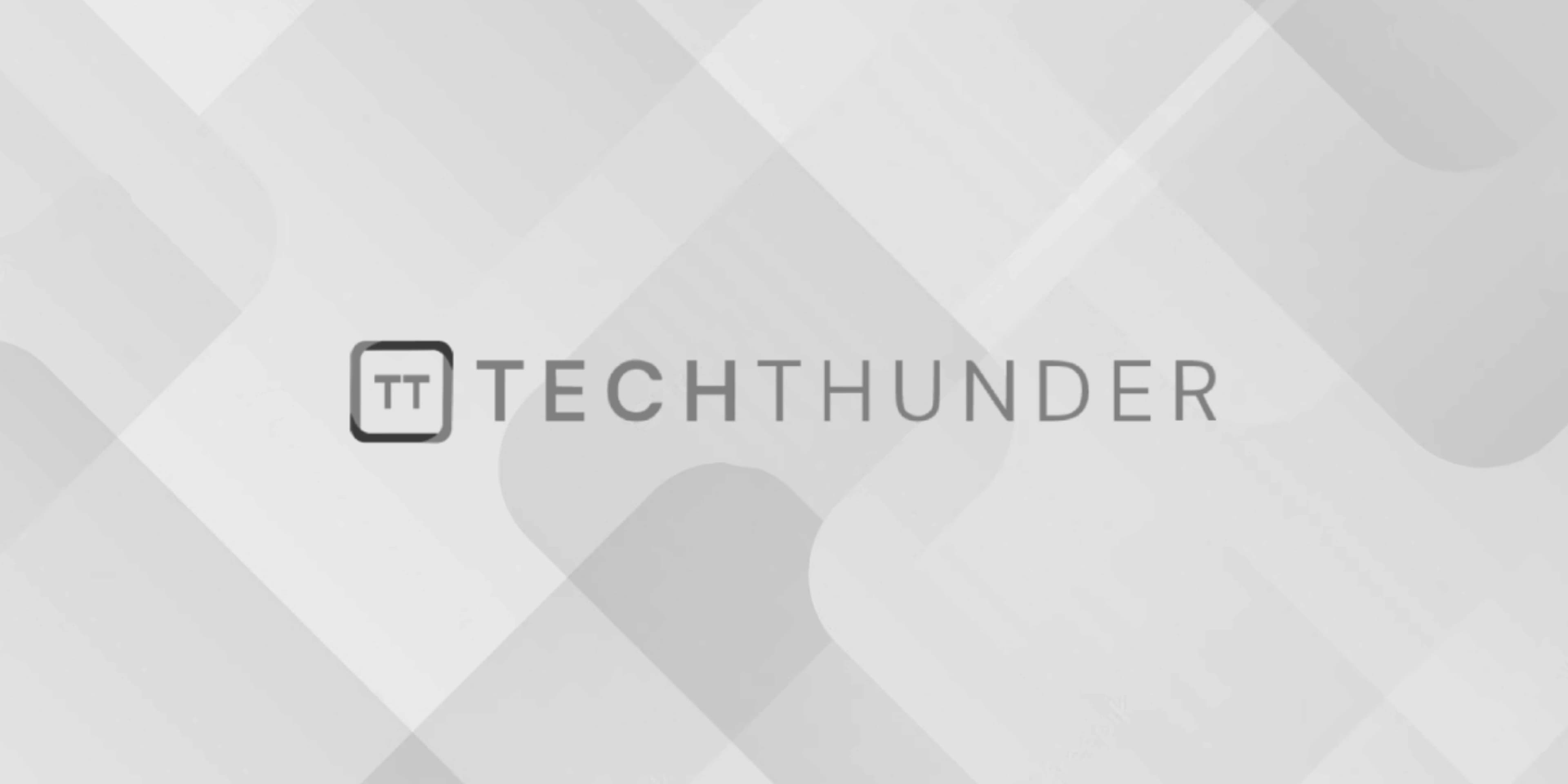
Spring with JAXB
Spring can be integrated with JAXB (Java Architecture for XML Binding) to simplify the process of marshalling and unmarshalling XML data in your Spring-based applications. JAXB allows you to convert XML data into Java objects and vice versa. Here’s how to use Spring with JAXB:
1. Set Up JAXB Annotations:
To use JAXB, you need to annotate your Java classes with JAXB annotations to specify how XML elements and attributes should be mapped to Java objects. For example:
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement
public class Book {
private String title;
private String author;
@XmlElement
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
@XmlElement
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
}
2. Configure JAXB Marshalling and Unmarshalling:
In your Spring configuration, you can set up JAXB for marshalling (converting Java objects to XML) and unmarshalling (converting XML to Java objects) using the Jaxb2Marshaller
class. Configure it as a Spring bean:
<bean id="jaxbMarshaller" class="org.springframework.oxm.jaxb.Jaxb2Marshaller">
<property name="classesToBeBound">
<list>
<value>com.example.Book</value>
<!-- Add more classes to be bound if needed -->
</list>
</property>
</bean>
In this configuration, you specify the Java classes that should be bound to XML using the classesToBeBound
property.
3. Use JAXB Marshaller and Unmarshaller:
Inject the Jaxb2Marshaller
bean into your Spring components or services where you need to perform marshalling or unmarshalling operations. For example:
import org.springframework.oxm.jaxb.Jaxb2Marshaller;
public class XmlService {
private Jaxb2Marshaller jaxbMarshaller;
public void setJaxbMarshaller(Jaxb2Marshaller jaxbMarshaller) {
this.jaxbMarshaller = jaxbMarshaller;
}
public String marshalObjectToXml(Object object) {
StringWriter writer = new StringWriter();
jaxbMarshaller.marshal(object, new StreamResult(writer));
return writer.toString();
}
public Object unmarshalXmlToObject(String xml) {
return jaxbMarshaller.unmarshal(new StreamSource(new StringReader(xml)));
}
}
In the above example, the marshalObjectToXml
method converts a Java object to XML, and the unmarshalXmlToObject
method converts XML to a Java object using the injected Jaxb2Marshaller
.
4. Configure the Application Context:
Ensure that you have an application context XML file that includes the configuration for the Jaxb2Marshaller
bean and any other Spring beans that use JAXB.
5. Use JAXB in Your Application:
Now you can use the XmlService
or any other component that utilizes JAXB for marshalling and unmarshalling XML data in your Spring application.
public class MainApp {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
XmlService xmlService = (XmlService) context.getBean("xmlService");
// Create a book object
Book book = new Book();
book.setTitle("Spring in Action");
book.setAuthor("Craig Walls");
// Marshal the book object to XML
String xml = xmlService.marshalObjectToXml(book);
System.out.println("XML representation of the book:\n" + xml);
// Unmarshal the XML back to a book object
Book unmarshalledBook = (Book) xmlService.unmarshalXmlToObject(xml);
System.out.println("Unmarshalled book: " + unmarshalledBook.getTitle() + " by " + unmarshalledBook.getAuthor());
}
}
In this example, we create a Book
object, marshal it to XML, and then unmarshal it back to a Java object.
Spring’s integration with JAXB makes it convenient to work with XML data in your Spring applications, providing a seamless way to convert XML documents to Java objects and vice versa.