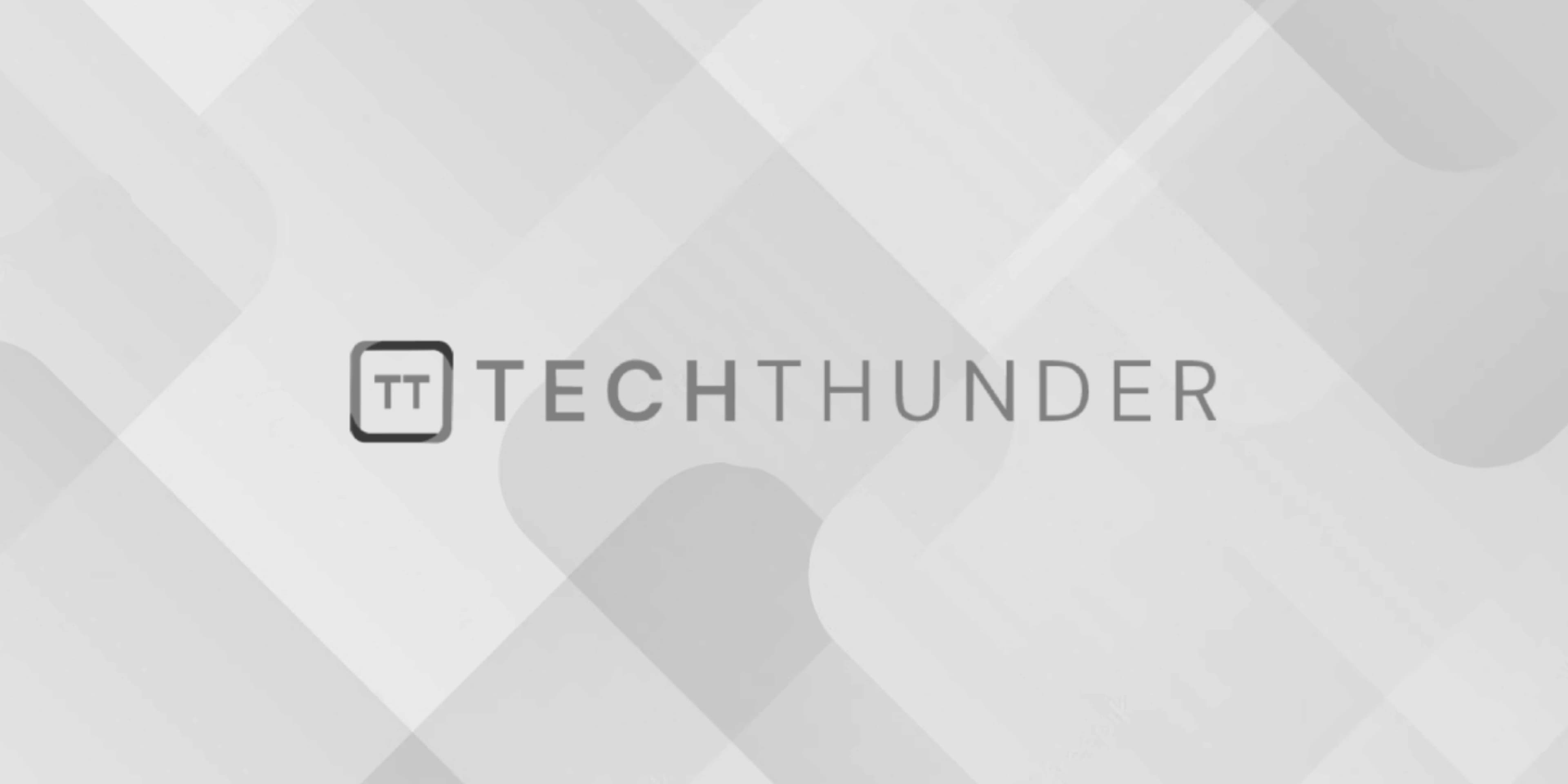
Spring MVC CRUD Example
Creating a Spring MVC CRUD (Create, Read, Update, Delete) example involves building a web application that allows users to perform these basic database operations on a specific entity (e.g., a “Product” entity). Here’s a step-by-step guide to creating a simple Spring MVC CRUD example:
1. Set Up Your Project:
You can use a build tool like Maven or Gradle to set up your Spring MVC project. Ensure you have the required dependencies, including Spring Web, Spring Data JPA (for database operations), and a database driver (e.g., H2 for simplicity).
2. Create Entity Class:
Create a Java class representing the entity you want to manage, e.g., Product
. Annotate it with JPA annotations to define its structure and relationships with the database.
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private double price;
// Getters and setters
}
3. Create a Repository Interface:
Create a repository interface for your entity by extending JpaRepository
(or another repository interface provided by Spring Data JPA).
public interface ProductRepository extends JpaRepository<Product, Long> {
}
4. Create a Controller:
Create a controller to handle CRUD operations. Define methods for creating, reading, updating, and deleting Product
objects.
@Controller
@RequestMapping("/products")
public class ProductController {
@Autowired
private ProductRepository productRepository;
@GetMapping
public String listProducts(Model model) {
List<Product> products = productRepository.findAll();
model.addAttribute("products", products);
return "product/list";
}
@GetMapping("/create")
public String createProductForm(Model model) {
model.addAttribute("product", new Product());
return "product/create";
}
@PostMapping("/create")
public String createProduct(@ModelAttribute Product product) {
productRepository.save(product);
return "redirect:/products";
}
@GetMapping("/edit/{id}")
public String editProductForm(@PathVariable Long id, Model model) {
Product product = productRepository.findById(id).orElse(null);
model.addAttribute("product", product);
return "product/edit";
}
@PostMapping("/edit")
public String editProduct(@ModelAttribute Product product) {
productRepository.save(product);
return "redirect:/products";
}
@GetMapping("/delete/{id}")
public String deleteProduct(@PathVariable Long id) {
productRepository.deleteById(id);
return "redirect:/products";
}
}
5. Create Views:
Create HTML templates (Thymeleaf or JSP) for listing, creating, editing, and deleting products. These views should correspond to the methods defined in your controller.
6. Configure Data Source:
Configure the data source and JPA properties in your application.properties
or application.yml
file.
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=password
spring.jpa.hibernate.ddl-auto=update
7. Run Your Application:
Run your Spring MVC CRUD application, and access it through a web browser. You should be able to perform CRUD operations on your Product
entity using the provided views and controllers.
This is a basic outline of creating a Spring MVC CRUD example. You can expand upon this foundation to include features like validation, security, and more complex relationships between entities as needed for your project.