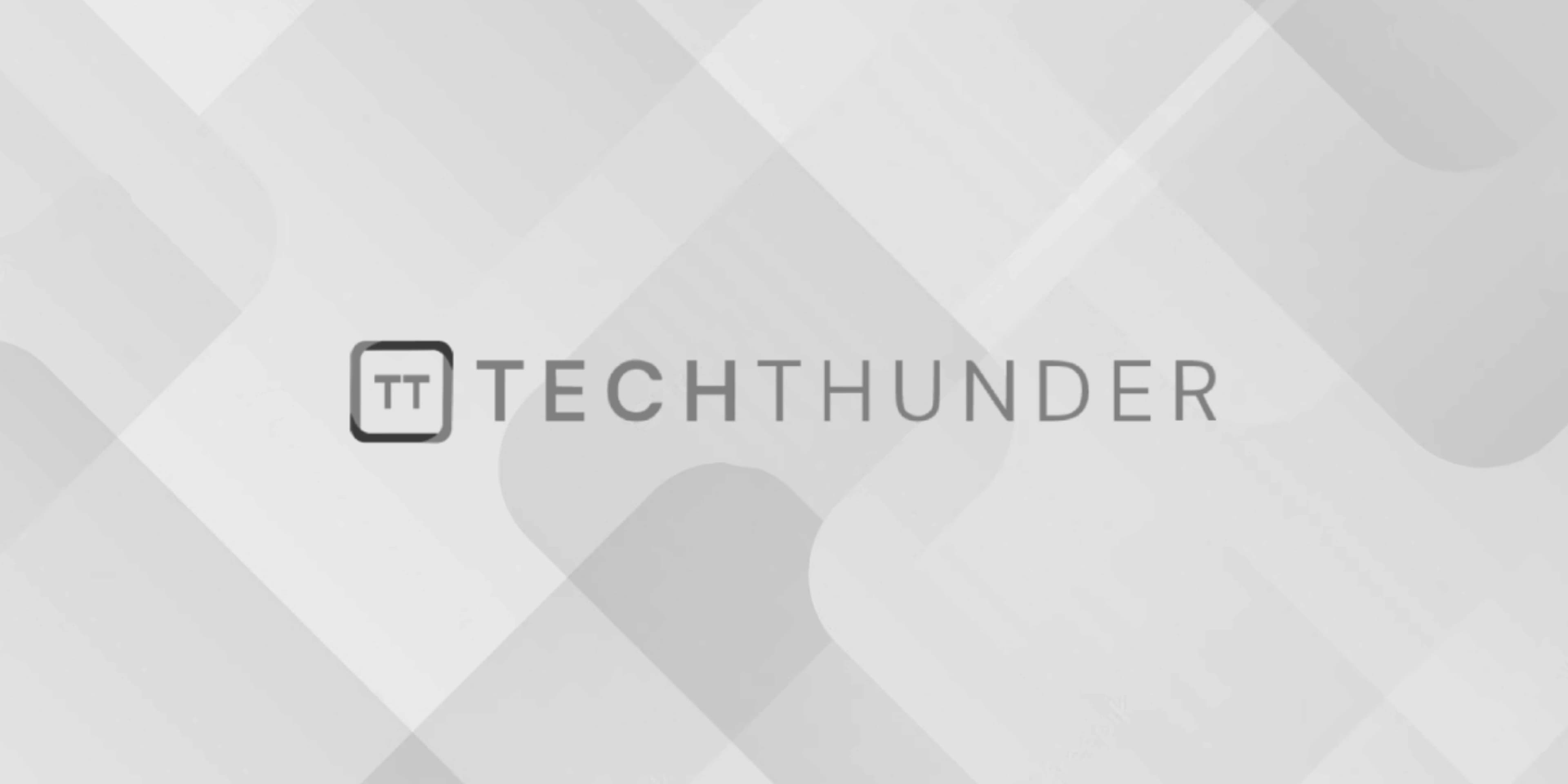
192 views
Spring Form Text Field
The Spring MVC, you can create a text input field in a form using the <form:input>
tag from the Spring Form Tag Library. This tag allows you to bind the text input field to a property of a model object and provides a way to display validation errors if necessary.
Here’s how to create a text input field using the <form:input>
tag:
- Include the Spring Form Tag Library: To use Spring Form tags in your JSP pages, you need to include the taglib declaration at the top of your JSP file:
<%@ taglib prefix="form" uri="http://www.springframework.org/tags/form" %>
- Create a Form and Input Field: In your JSP file, create a form using the
<form:form>
tag and add an<form:input>
tag to create a text input field. Bind the input field to a property of a model object using thepath
attribute.
<form:form modelAttribute="user" method="POST" action="/submit">
<div>
<label for="username">Username:</label>
<form:input path="username" id="username" />
</div>
<!-- Other form fields go here -->
<div>
<input type="submit" value="Submit" />
</div>
</form:form>
In this example, we’re creating a text input field for the “username” property of the “user” model object.
- Handle Form Submission in a Controller: In your Spring MVC controller, handle the form submission and bind the form data to a model object. You can then process the data as needed.
@Controller
public class UserController {
@GetMapping("/form")
public String showForm(Model model) {
model.addAttribute("user", new User()); // Initialize the model object
return "form";
}
@PostMapping("/submit")
public String submitForm(@ModelAttribute("user") User user) {
// Process the user object (e.g., save to a database)
return "result"; // Redirect to a result page
}
}
In this example, the @ModelAttribute
annotation is used to bind the form data to a “user” model object.
- Validation and Error Handling (Optional): You can add validation rules to your model object using annotations (e.g.,
@NotNull
,@Size
, etc.). If validation fails, Spring MVC will automatically handle error messages. You can display these error messages next to the input fields in your JSP using<form:errors>
tags.
public class User {
@NotEmpty(message = "Username is required")
private String username;
// Getters and setters
}
<div>
<label for="username">Username:</label>
<form:input path="username" id="username" />
<form:errors path="username" cssClass="error" />
</div>
This setup allows you to create a text input field in a Spring MVC form, bind it to a model object, and handle form submissions, including validation and error handling.