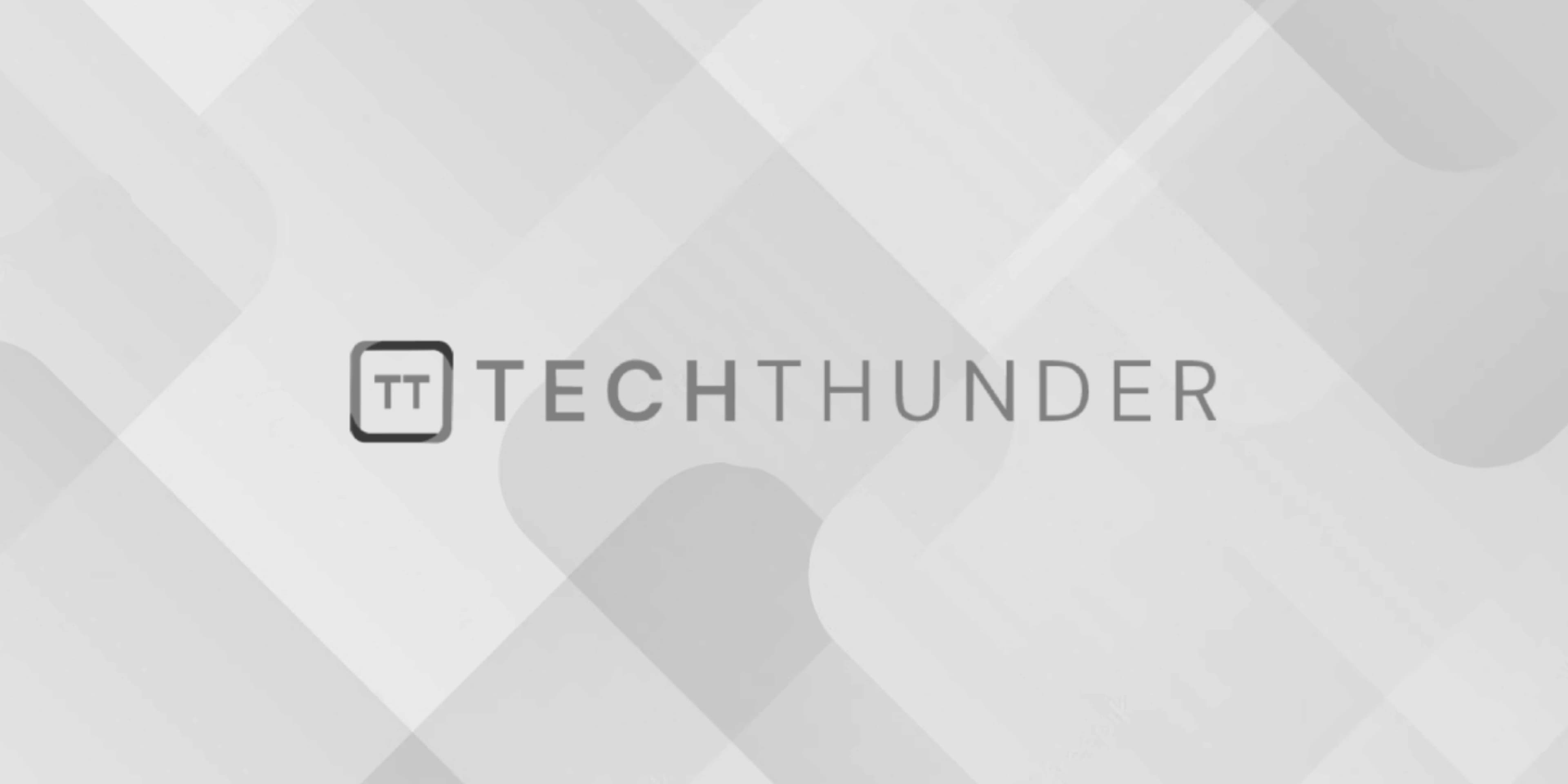
Spring with JMS
Spring provides excellent support for working with JMS (Java Messaging Service), which is a Java-based messaging standard for building enterprise-level messaging applications. JMS enables communication between different components of an application or between different applications in a loosely coupled and asynchronous manner. Below are the steps to use Spring with JMS:
1. Set Up Your Environment:
Ensure that you have a JMS provider installed and configured. Popular JMS providers include Apache ActiveMQ, RabbitMQ, and IBM MQ. You will need the necessary dependencies for your chosen JMS provider in your project’s build configuration.
2. Create a JMS Connection Factory Bean:
In your Spring configuration, define a ConnectionFactory
bean that represents the connection to your JMS provider.
<bean id="connectionFactory" class="org.springframework.jms.connection.SingleConnectionFactory">
<property name="targetConnectionFactory">
<!-- Configure the connection factory specific to your JMS provider -->
<!-- For example, for ActiveMQ: -->
<bean class="org.apache.activemq.spring.ActiveMQConnectionFactory">
<property name="brokerURL" value="tcp://localhost:61616"/>
</bean>
</property>
</bean>
This example uses ActiveMQ as the JMS provider, but you can replace it with the configuration for your chosen provider.
3. Configure a JMS Destination:
Define a JMS destination (queue or topic) that your application will send messages to or receive messages from.
<bean id="myQueue" class="org.apache.activemq.command.ActiveMQQueue">
<constructor-arg value="my-queue"/>
</bean>
4. Create a JMS Template:
Define a JMS template that simplifies JMS operations, such as sending and receiving messages.
<bean id="jmsTemplate" class="org.springframework.jms.core.JmsTemplate">
<property name="connectionFactory" ref="connectionFactory"/>
<property name="defaultDestination" ref="myQueue"/>
</bean>
5. Create a JMS Producer:
Define a component or service that sends messages to the JMS destination using the JMS template.
import org.springframework.jms.core.JmsTemplate;
public class JmsMessageProducer {
private JmsTemplate jmsTemplate;
public void setJmsTemplate(JmsTemplate jmsTemplate) {
this.jmsTemplate = jmsTemplate;
}
public void sendMessage(String message) {
jmsTemplate.convertAndSend(message);
}
}
6. Create a JMS Consumer:
Define a component or service that receives messages from the JMS destination using the JMS template.
import org.springframework.jms.core.JmsTemplate;
public class JmsMessageConsumer {
private JmsTemplate jmsTemplate;
public void setJmsTemplate(JmsTemplate jmsTemplate) {
this.jmsTemplate = jmsTemplate;
}
public String receiveMessage() {
return (String) jmsTemplate.receiveAndConvert();
}
}
7. Sending and Receiving Messages:
Now, you can use your JMS producer to send messages to the JMS destination and the JMS consumer to receive messages from it.
public class JmsApp {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
JmsMessageProducer producer = (JmsMessageProducer) context.getBean("jmsMessageProducer");
JmsMessageConsumer consumer = (JmsMessageConsumer) context.getBean("jmsMessageConsumer");
// Send a message
producer.sendMessage("Hello, JMS!");
// Receive a message
String message = consumer.receiveMessage();
System.out.println("Received: " + message);
}
}
8. Exception Handling and Error Handling:
Be sure to handle exceptions that may occur during JMS operations, and configure error handling strategies as needed.
9. Security and Configuration:
Depending on your JMS provider, you may need to configure security settings and other provider-specific configurations.
Spring’s JMS support simplifies the integration of JMS into your Spring-based application, providing a consistent and easy-to-use API for messaging operations.