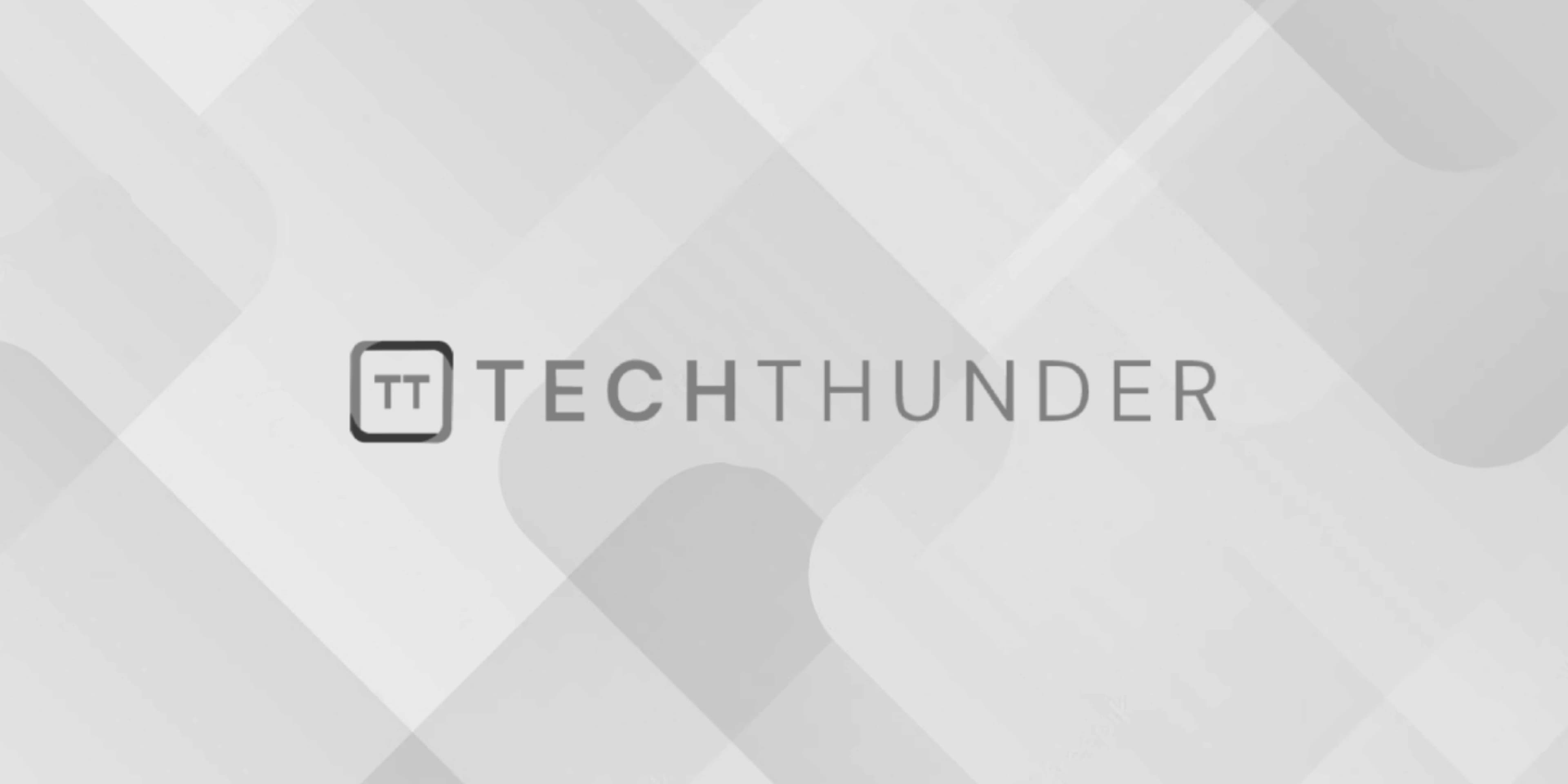
161 views
Spring Constructor Injection vs Setter Injection
The Spring, both constructor injection and setter injection are methods for injecting dependencies (i.e., other beans or objects) into a target bean. Each injection method has its advantages and use cases, and the choice between them depends on your application’s requirements and design preferences. Here’s a comparison of constructor injection and setter injection:
Constructor Injection:
- Advantages:
- Provides immutability: When using constructor injection, you can make the injected dependencies final or effectively final, ensuring that they cannot be changed after object creation. This helps enforce immutability and can lead to safer and more predictable behavior.
- Guarantees that dependencies are injected: Since dependencies are required when the object is created, it guarantees that the object is in a valid state from the beginning. This can help prevent null or uninitialized dependencies.
- Encourages better design practices: Constructor injection often encourages you to design classes with well-defined and required dependencies, promoting better separation of concerns and single responsibility principles.
- Use Cases:
- Use constructor injection when a bean has mandatory dependencies that must be provided at the time of creation.
- It’s suitable for cases where you want to ensure that the bean is always in a valid state.
- Example:
public class OrderService {
private final OrderRepository orderRepository;
public OrderService(OrderRepository orderRepository) {
this.orderRepository = orderRepository;
}
// Other methods
}
Setter Injection:
- Advantages:
- Provides flexibility: Setter injection allows you to change dependencies at runtime, which can be beneficial when you have optional dependencies or need to reconfigure a bean’s dependencies dynamically.
- Easier to work with a default configuration: Setter injection can be convenient when you have a default set of dependencies but want to allow them to be overridden if needed.
- Use Cases:
- Use setter injection when a bean has optional or mutable dependencies.
- It’s suitable for cases where you want to provide flexibility in configuring a bean’s dependencies.
- Example:
public class ShoppingCart {
private PaymentProcessor paymentProcessor;
public void setPaymentProcessor(PaymentProcessor paymentProcessor) {
this.paymentProcessor = paymentProcessor;
}
// Other methods
}
General Considerations:
- You can also use a combination of both constructor and setter injection in the same bean, which is known as mixed injection.
- Constructor injection is typically favored when dependencies are required, while setter injection is used when dependencies are optional or changeable.
- Consider using constructor injection when you want to minimize the complexity of configuration files and ensure that all required dependencies are explicitly provided.
- Setter injection is often chosen when you need more flexibility in configuring beans or when you want to configure dependencies dynamically.
Ultimately, the choice between constructor injection and setter injection should align with your application’s specific needs and design goals. Both approaches are valuable tools in the Spring framework, and you can select the one that best fits your use case.