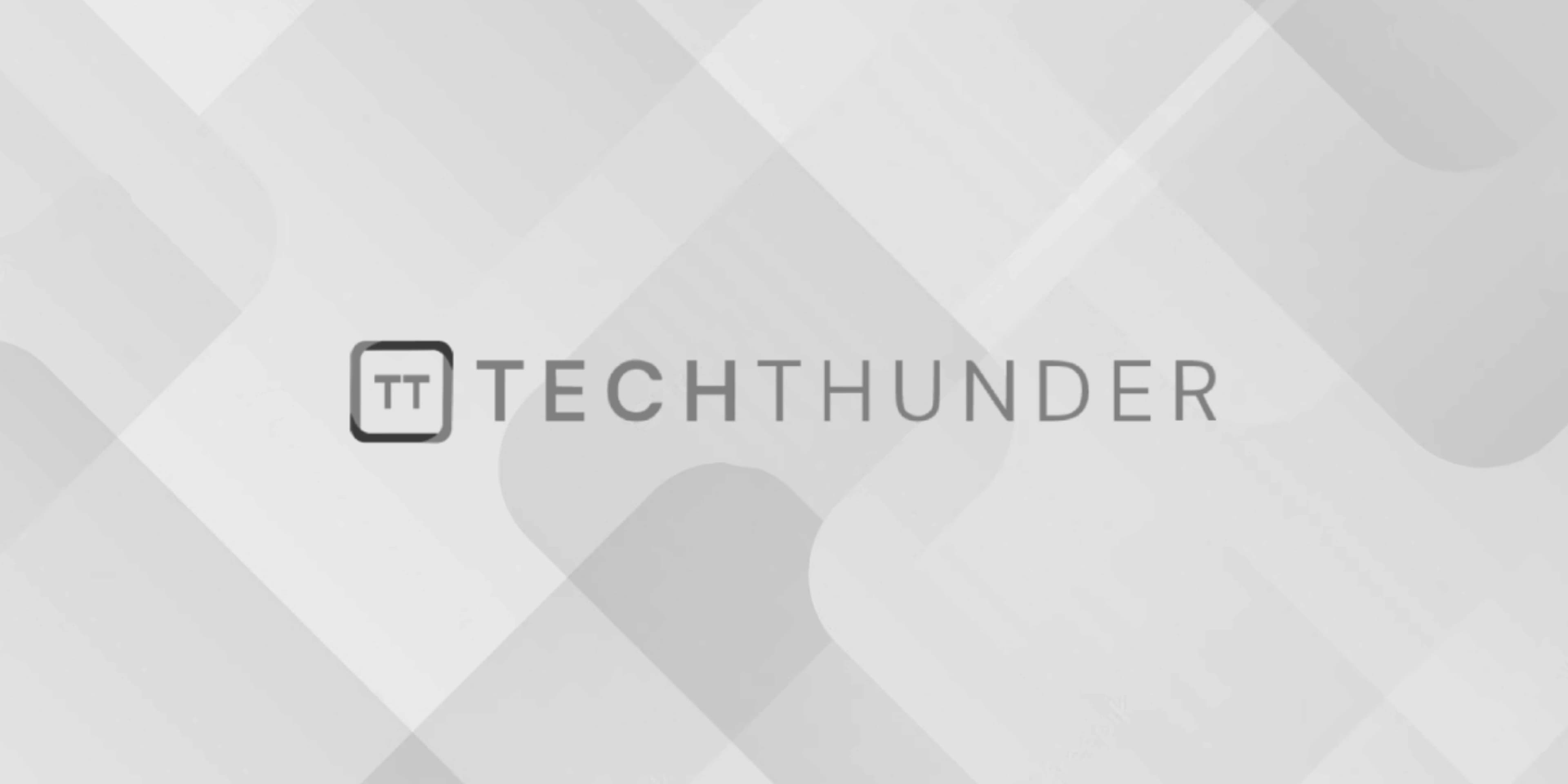
Spring Http Invoker
Spring provides support for creating and consuming remote services using HTTP Invoker, a protocol that allows remote method invocations over HTTP. HTTP Invoker is particularly useful for building distributed systems where the client and server communicate via HTTP. Here’s how to use Spring’s HTTP Invoker:
1. Define the Service Interface:
Start by defining the service interface that both the client and server will use. This interface should contain the methods that the client will invoke remotely.
public interface MyRemoteService {
String sayHello(String name);
}
2. Implement the Service:
Create a class that implements the service interface. This class will provide the actual implementation of the remote methods.
public class MyRemoteServiceImpl implements MyRemoteService {
@Override
public String sayHello(String name) {
return "Hello, " + name + "!";
}
}
3. Configure the HTTP Invoker Service:
In your Spring configuration, define the HTTP Invoker service by using the HttpInvokerServiceExporter
class. This class exposes the service over HTTP.
<bean id="myRemoteService" class="com.example.MyRemoteServiceImpl"/>
<bean id="httpInvokerServiceExporter" class="org.springframework.remoting.httpinvoker.HttpInvokerServiceExporter">
<property name="service" ref="myRemoteService"/>
<property name="serviceInterface" value="com.example.MyRemoteService"/>
</bean>
4. Create the HTTP Invoker Client:
On the client side, configure Spring to create an HTTP Invoker proxy for the remote service.
<bean id="myRemoteServiceProxy" class="org.springframework.remoting.httpinvoker.HttpInvokerProxyFactoryBean">
<property name="serviceUrl" value="http://localhost:8080/myRemoteService"/>
<property name="serviceInterface" value="com.example.MyRemoteService"/>
</bean>
In this example, we specify the URL of the HTTP Invoker service (including the host and port) and the service interface. The myRemoteServiceProxy
bean can be injected into your client code for remote method invocation.
5. Use the HTTP Invoker Service:
Now you can use the myRemoteServiceProxy
bean to invoke methods on the remote service just like you would with a local bean.
public class MyHttpInvokerClient {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
MyRemoteService remoteService = (MyRemoteService) context.getBean("myRemoteServiceProxy");
String response = remoteService.sayHello("John");
System.out.println(response);
}
}
6. Run the HTTP Invoker Server and Client:
Start the HTTP Invoker server (where the service is hosted) and then run the HTTP Invoker client to make remote method calls.
7. Security Considerations:
When using HTTP Invoker, it’s important to consider security, especially if your application operates over the internet. You may need to secure your HTTP Invoker endpoints and configure authentication and authorization mechanisms.
This example provides a basic introduction to using Spring’s HTTP Invoker. Spring simplifies the configuration and usage of HTTP Invoker, making it easier to develop distributed Java applications over HTTP.