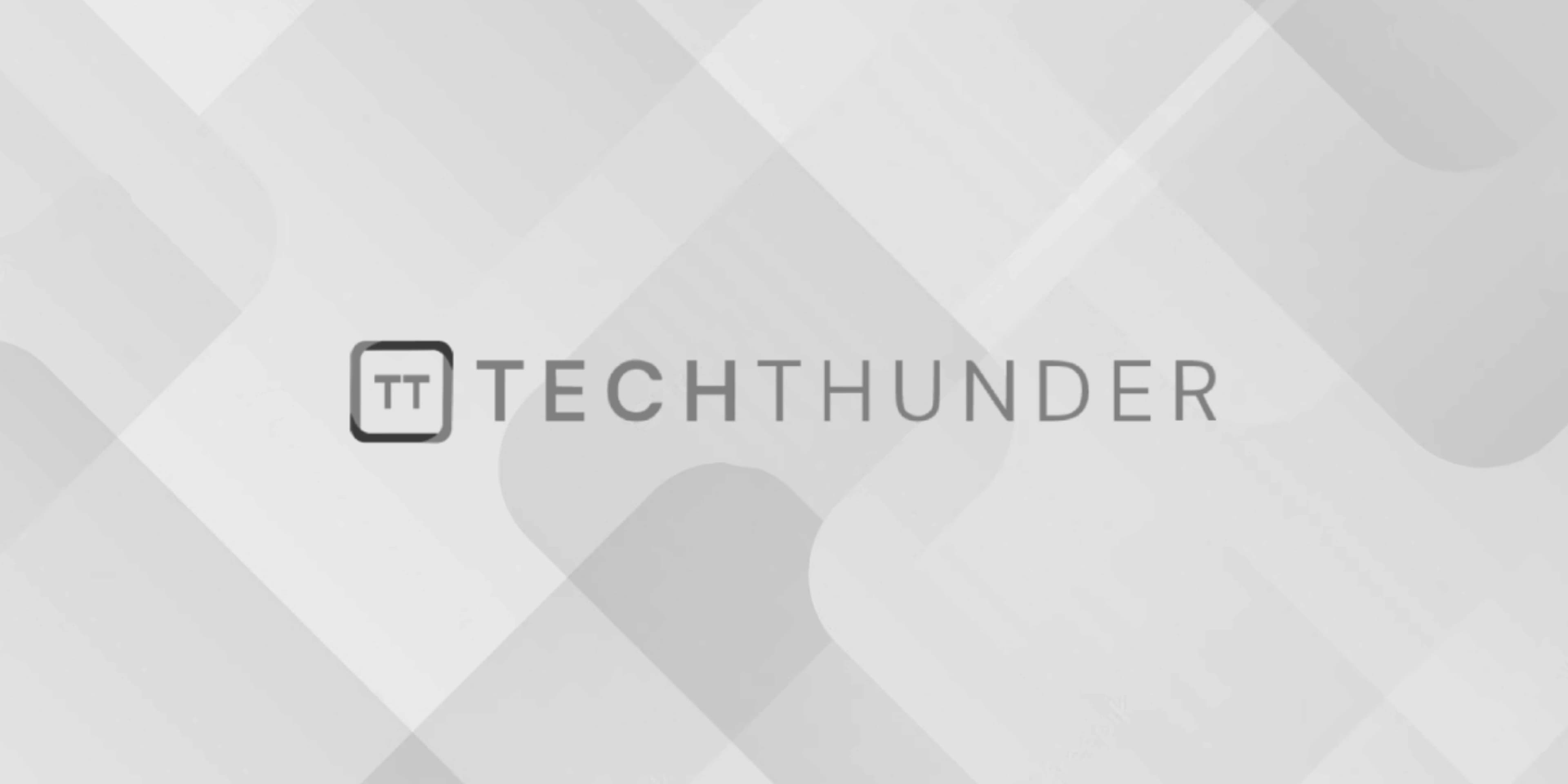
Spring Autowiring
The Spring, autowiring is a feature that allows you to automatically inject dependencies (other beans or components) into a Spring bean without explicitly specifying the dependencies in your bean configuration. Autowiring can help simplify your Spring configuration and reduce the need for manual wiring. Spring provides several autowiring modes, and you can choose the one that suits your needs. Here are the common autowiring modes:
1. No Autowiring (autowire="no"
): This is the default behavior. You need to specify the dependencies manually using <property>
or constructor-arg elements in your bean configuration. No automatic injection occurs.
<bean id="myBean" class="com.example.MyBean">
<property name="dependency" ref="anotherBean" />
</bean>
2. Autowire by Type (autowire="byType"
): Spring automatically injects the dependency if there’s exactly one bean of the required type available in the container. If there are multiple candidates, or no matching bean is found, it will throw an exception.
<bean id="myBean" class="com.example.MyBean" autowire="byType" />
3. Autowire by Name (autowire="byName"
): Spring tries to find a bean with a name that matches the property or constructor argument name in the target bean. If found, it injects the matching bean.
<bean id="myBean" class="com.example.MyBean" autowire="byName" />
4. Autowire by Constructor (autowire="constructor"
): Spring attempts to match constructor arguments by type. If a constructor parameter type matches the type of a bean in the container, it injects that bean into the constructor.
<bean id="myBean" class="com.example.MyBean" autowire="constructor" />
5. Autowire by Qualifier (@Qualifier
annotation): When using @Autowired
or @Inject
annotations for autowiring, you can also use @Qualifier
to specify which bean to inject when multiple beans of the same type are available.
@Autowired
@Qualifier("specificBean")
private MyBean myBean;
6. Autowire by Primary (@Primary
annotation): If you have multiple beans of the same type and want to specify a primary candidate for autowiring, you can use the @Primary
annotation on one of the beans.
@Bean
@Primary
public MyBean primaryBean() {
return new MyBean();
}
7. Autowire with Java Configuration: In Java-based Spring configurations, you can use the @Autowired
annotation along with Java configuration to specify autowiring. You can use @Autowired
on fields, methods, or constructors.
@Configuration
public class AppConfig {
@Autowired
private MyBean myBean;
// ...
}
Autowiring can help simplify your Spring configuration and reduce the boilerplate code required for setting up bean dependencies. However, it’s essential to use it carefully, as it may lead to unexpected behavior when multiple beans of the same type exist in the application context. You should also ensure that your beans have unique names or use qualifiers to specify the exact beans to be injected when needed.