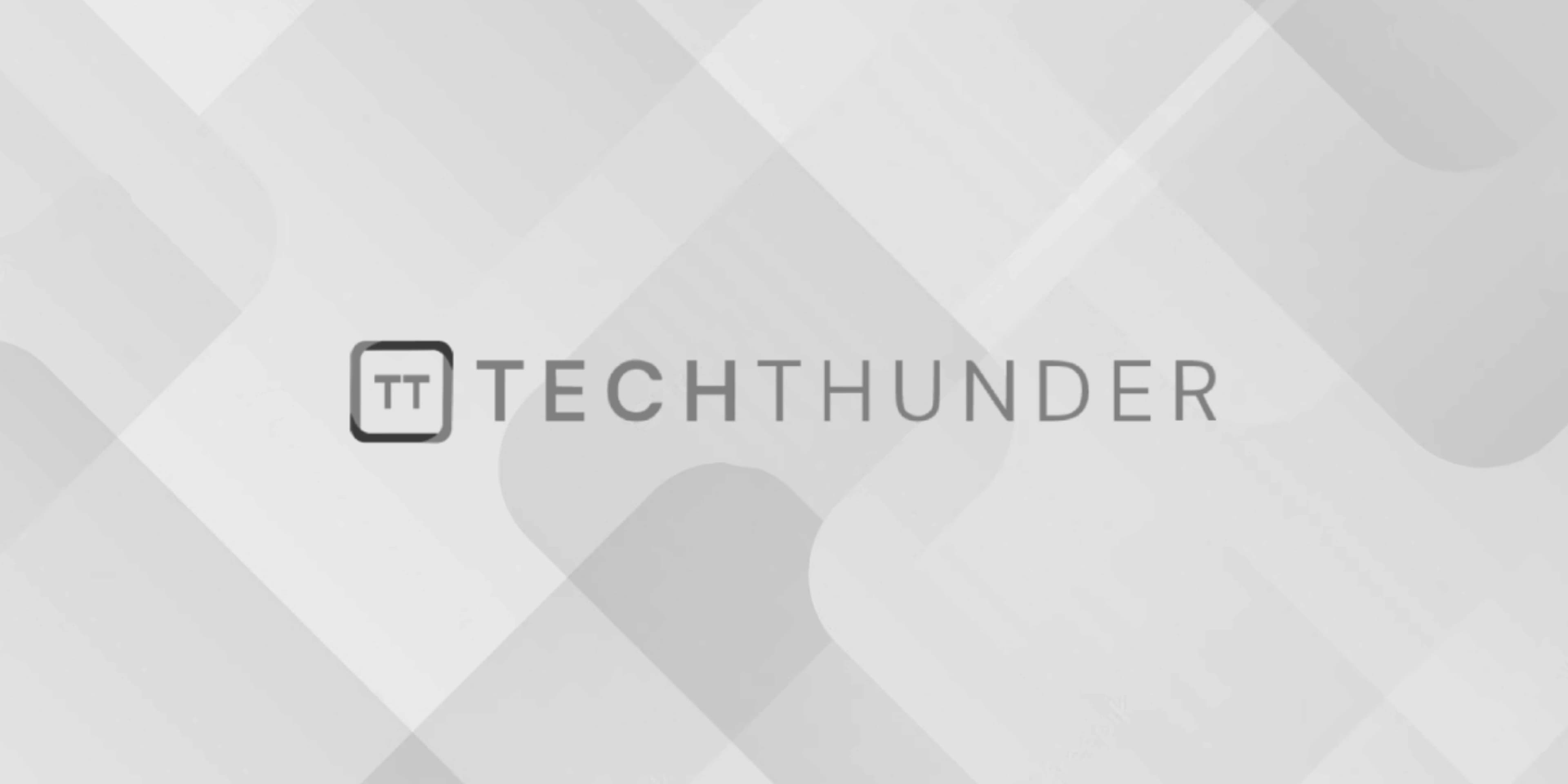
Spring CRUD Example
The simple example of creating a CRUD (Create, Read, Update, Delete) application using Spring Boot. This example demonstrates how to create a basic web application to manage a list of “items” using Spring Data JPA and Thymeleaf templating engine.
1. Create a Spring Boot Project:
Start by creating a new Spring Boot project using Spring Initializr or your preferred development environment.
2. Define the Item Entity:
Create an Item
entity class with fields for an ID, name, and description.
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Item {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String description;
// getters and setters
}
3. Create a Repository:
Create a repository interface for the Item
entity by extending JpaRepository
.
import org.springframework.data.jpa.repository.JpaRepository;
public interface ItemRepository extends JpaRepository<Item, Long> {
}
4. Implement CRUD Controller:
Create a controller that handles CRUD operations for the Item
entity.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.*;
@Controller
@RequestMapping("/items")
public class ItemController {
@Autowired
private ItemRepository itemRepository;
@GetMapping
public String listItems(Model model) {
model.addAttribute("items", itemRepository.findAll());
return "items/list";
}
@GetMapping("/new")
public String newItemForm(Model model) {
model.addAttribute("item", new Item());
return "items/new";
}
@PostMapping
public String createItem(Item item) {
itemRepository.save(item);
return "redirect:/items";
}
// Implement update and delete methods
}
5. Create HTML Templates:
Create Thymeleaf templates for listing items, creating new items, updating items, and displaying item details.
src/main/resources/templates/items/list.html
src/main/resources/templates/items/new.html
src/main/resources/templates/items/edit.html
src/main/resources/templates/items/details.html
These templates will use Thymeleaf to display and interact with the data.
6. Configure Application Properties:
Configure your database connection and other properties in src/main/resources/application.properties
or application.yml
.
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.jpa.hibernate.ddl-auto=update
7. Run the Application:
Run your Spring Boot application. You should be able to access the CRUD functionality through your browser at http://localhost:8080/items
.
This example provides a basic overview of setting up a CRUD application using Spring Boot, Spring Data JPA, and Thymeleaf. Depending on your requirements, you can enhance the application by adding validation, security, pagination, error handling, and more.