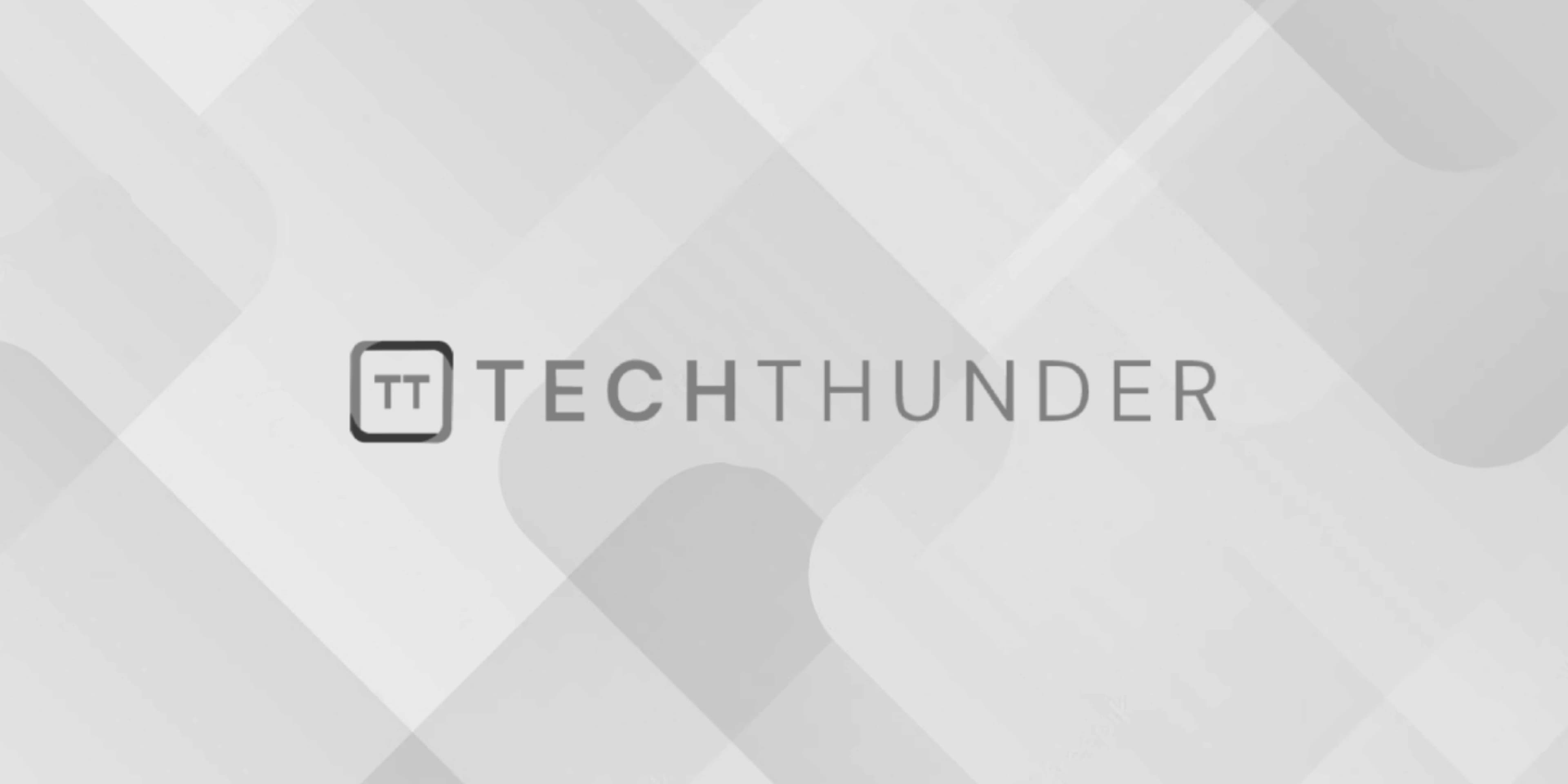
Spring variable in SpEL
The Spring Expression Language (SpEL), you can define and use variables within expressions. These variables provide a way to reuse values or objects within your expressions, making them more readable and maintainable. Here’s how you can define and use variables in SpEL:
1. Inline Variables:
You can define inline variables directly within SpEL expressions using the #
symbol followed by the variable name and the =
operator. For example:
<bean id="myBean" class="com.example.MyBean">
<property name="result" value="#{#myVariable = 5; 10 + #myVariable}" />
</bean>
In this example, #myVariable
is defined inline, assigned the value 5, and then used in the expression to calculate 10 + #myVariable
.
2. Using SpringELContext
(Programmatic Approach):
You can also define variables programmatically using the StandardEvaluationContext
class. Here’s an example in Java:
import org.springframework.expression.EvaluationContext;
import org.springframework.expression.spel.standard.SpelExpressionParser;
import org.springframework.expression.spel.support.StandardEvaluationContext;
public class MyBean {
private int result;
public void setResult(int result) {
this.result = result;
}
public static void main(String[] args) {
SpelExpressionParser parser = new SpelExpressionParser();
StandardEvaluationContext context = new StandardEvaluationContext();
// Define a variable in the context
context.setVariable("myVariable", 5);
// Evaluate an expression using the context
int calculatedResult = parser.parseExpression("#myVariable + 10").getValue(context, Integer.class);
System.out.println("Result: " + calculatedResult);
}
}
In this Java example, we use the StandardEvaluationContext
to define the variable myVariable
, which is then used in the SpEL expression.
3. Using ApplicationContext
(Spring Bean Configuration):
In Spring bean configuration files, you can also define variables using the <util:map>
element. Here’s an example:
<util:map id="variablesMap">
<entry key="myVariable" value="5" />
</util:map>
<bean id="myBean" class="com.example.MyBean">
<property name="result" value="#{variablesMap.myVariable + 10}" />
</bean>
In this example, we define a map named variablesMap
that contains the myVariable
variable with a value of 5. We then use this variable in the SpEL expression.
Using variables in SpEL can make your expressions more dynamic and reusable. You can define variables at various scopes, including inline, programmatic, and in Spring bean configurations, depending on your specific use case and requirements.