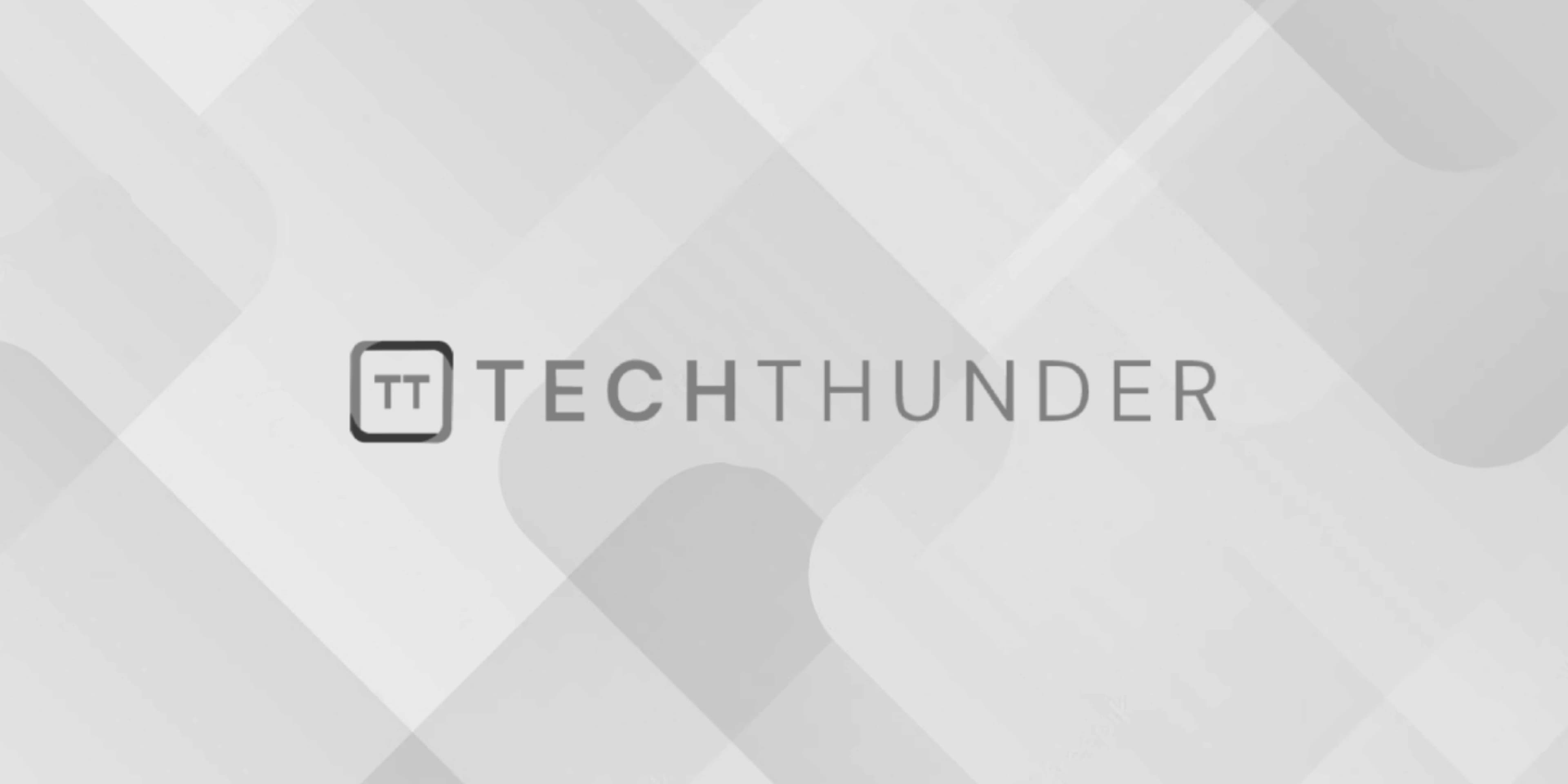
Search Field Example
To build a simple example of implementing a search functionality using Spring Boot and Thymeleaf. In this example, we’ll create a web application that allows users to search for items from a list.
1. Create a Spring Boot Project:
Start by creating a new Spring Boot project using Spring Initializr or your preferred development environment.
2. Create an Item Entity:
Create an Item
entity class similar to the previous examples. This class should represent the items that users can search for.
@Entity
public class Item {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String description;
// getters and setters
}
3. Create a Repository:
Create a repository interface for the Item
entity, as before.
public interface ItemRepository extends JpaRepository<Item, Long> {
List<Item> findByNameContainingIgnoreCase(String keyword);
}
4. Create a Controller:
Create a controller that handles the search functionality.
@Controller
@RequestMapping("/items")
public class ItemController {
@Autowired
private ItemRepository itemRepository;
@GetMapping("/search")
public String searchItems(@RequestParam("keyword") String keyword, Model model) {
List<Item> searchResults = itemRepository.findByNameContainingIgnoreCase(keyword);
model.addAttribute("searchResults", searchResults);
return "items/search-results";
}
}
5. Create HTML Templates:
Create Thymeleaf templates for displaying the search form and the search results.
src/main/resources/templates/items/search-form.html
src/main/resources/templates/items/search-results.html
6. Run the Application:
Run your Spring Boot application. You can access the search form at http://localhost:8080/items/search-form
and the search results will be displayed after entering a search term.
search-form.html
:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Item Search</title>
</head>
<body>
<h2>Search Items</h2>
<form th:action="@{/items/search}" method="get">
<input type="text" name="keyword" placeholder="Enter search keyword">
<button type="submit">Search</button>
</form>
</body>
</html>
search-results.html
:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Search Results</title>
</head>
<body>
<h2>Search Results</h2>
<ul>
<li th:each="item : ${searchResults}">
<strong th:text="${item.name}"></strong>: <span th:text="${item.description}"></span>
</li>
</ul>
<a href="/items/search-form">Back to Search</a>
</body>
</html>
Remember that this is a basic example and you can expand it with features like pagination, advanced search options, and more sophisticated UI.