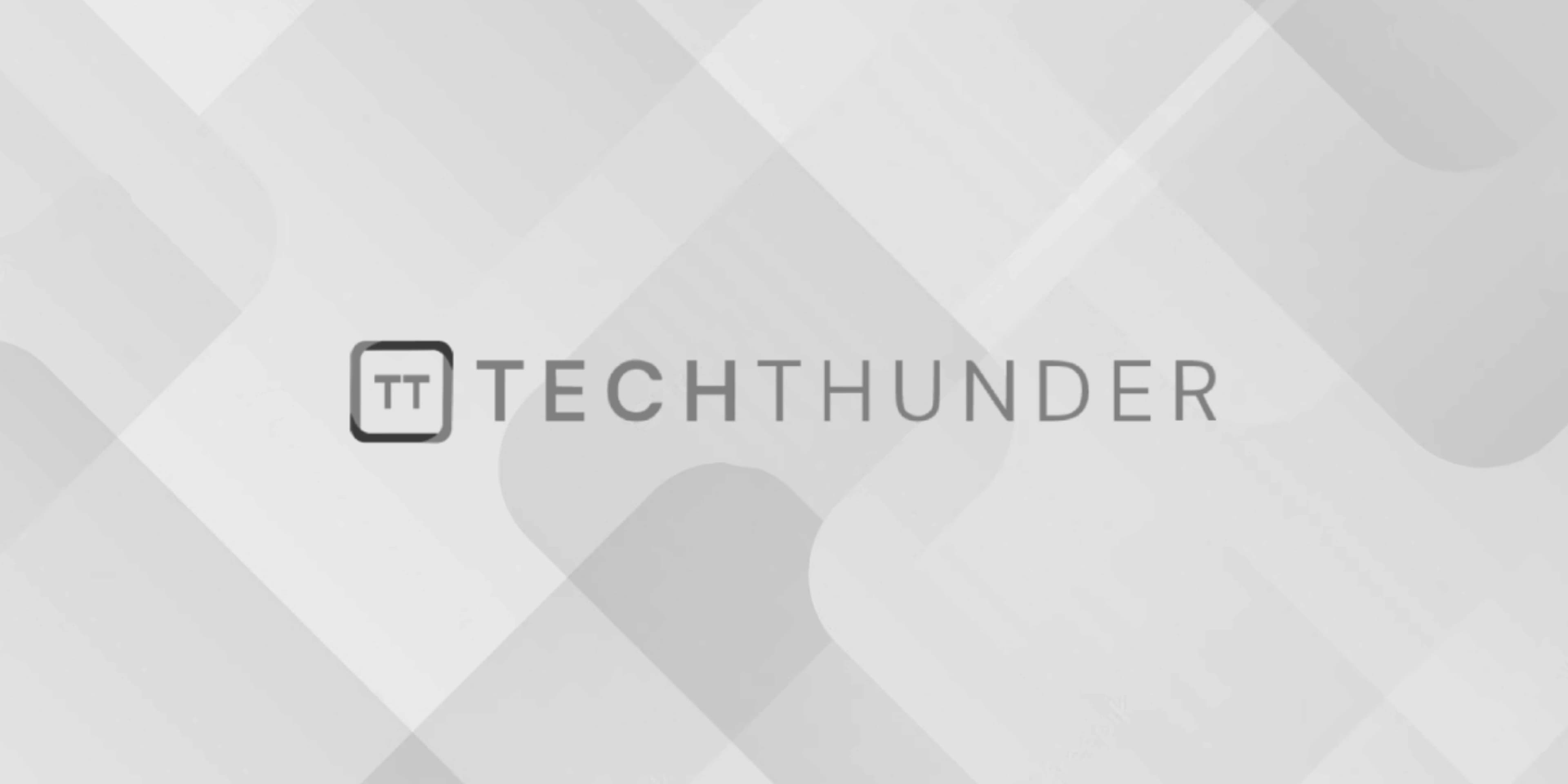
Spring Factory Method
The Spring, a factory method is a design pattern used for creating objects. Spring supports factory methods through its container configuration, allowing you to define and configure beans using factory methods. Factory methods are useful when the process of creating an object is more complex or requires specific logic beyond the default constructor. Here’s how to use factory methods in Spring:
1. Define a Factory Class:
First, create a factory class that contains one or more static or instance methods responsible for creating instances of your target class. The factory class can be any regular Java class.
public class MyFactory {
public static MyBean createMyBean() {
// Custom logic to create and configure MyBean
MyBean myBean = new MyBean();
myBean.setProperty1("Value1");
myBean.setProperty2("Value2");
return myBean;
}
}
In this example, the MyFactory
class has a static factory method createMyBean()
that creates and configures an instance of MyBean
.
2. Configure the Bean Definition:
In your Spring configuration file (e.g., applicationContext.xml
or Java configuration class), define a bean using the <bean>
element and specify the factory method using the factory-method
attribute.
<bean id="myBean" class="com.example.MyFactory" factory-method="createMyBean" />
In this XML configuration, we define a bean named “myBean” of the class com.example.MyFactory
and specify the factory method to use, which is createMyBean
. When Spring initializes the “myBean” bean, it will call the createMyBean
method of the factory class to obtain the instance.
3. Access the Bean:
You can access the bean as usual, either by retrieving it from the Spring application context or by injecting it into other beans or components.
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Main {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
MyBean myBean = context.getBean("myBean", MyBean.class);
// Use myBean
}
}
In this example, we retrieve the “myBean” bean from the Spring context, and Spring calls the factory method createMyBean()
to create the MyBean
instance.
Using factory methods in Spring provides flexibility and allows you to encapsulate complex object creation logic in separate factory classes. This approach is especially useful when creating objects with non-trivial setup or when you need to control the instantiation process more precisely.