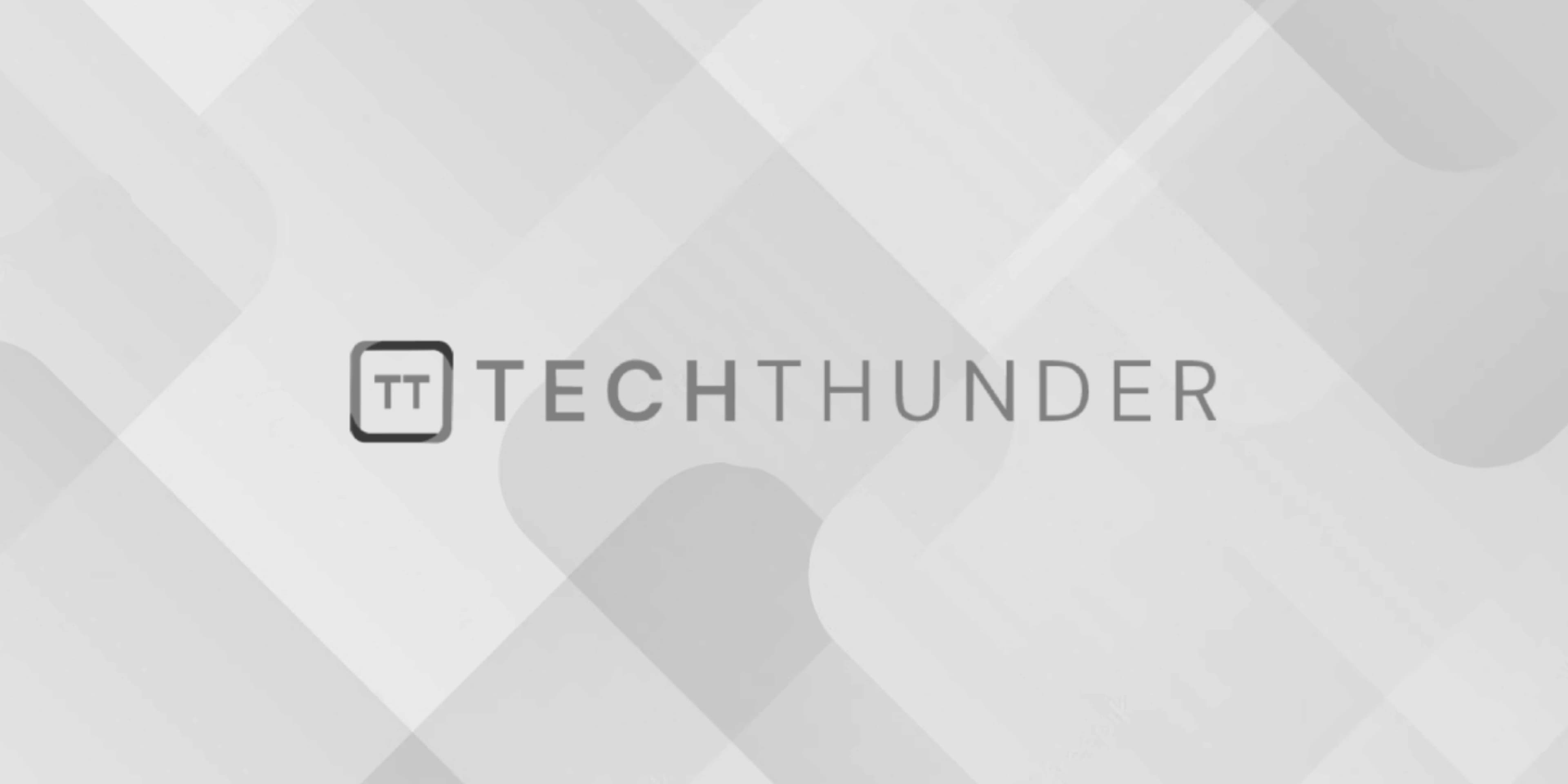
Spring Setter Injection with Collection
The Spring, you can use setter injection with collections to inject multiple dependent objects or beans into another bean. This is useful when you need to inject a list, set, or map of objects into a target bean. Here’s a step-by-step guide on how to use setter injection with collections in Spring:
1. Create the Dependent Objects:
First, create the dependent objects or beans that you want to inject into another bean. These dependent objects should have their own properties and behaviors. For example, let’s create a Person
class:
public class Person {
private String name;
// Getter and setter methods for the 'name' property
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
2. Create the Target Bean:
Next, create the target bean that will depend on a collection of Person
objects. The target bean should provide a setter method to receive the collection of Person
objects. For example:
import java.util.List;
public class GreetingService {
private List<Person> people;
// Setter method for injecting the collection of 'Person' objects
public void setPeople(List<Person> people) {
this.people = people;
}
public void greetAll() {
for (Person person : people) {
System.out.println("Hello, " + person.getName() + "!");
}
}
}
In this example, the GreetingService
bean has a List<Person>
property, and a setter method setPeople
is provided for setter injection.
3. Configure Spring Beans:
Configure the beans in your Spring configuration file (e.g., applicationContext.xml
) to define the Person
objects and the GreetingService
bean. Also, configure the setter injection by specifying the <property>
element in the GreetingService
bean definition. You can use the <list>
element to define the list of Person
objects:
<bean id="person1" class="com.example.Person">
<property name="name" value="John" />
</bean>
<bean id="person2" class="com.example.Person">
<property name="name" value="Alice" />
</bean>
<bean id="greetingService" class="com.example.GreetingService">
<!-- Setter injection with a list -->
<property name="people">
<list>
<ref bean="person1" />
<ref bean="person2" />
</list>
</property>
</bean>
In this XML configuration, we define two Person
beans named “person1” and “person2,” and we define the GreetingService
bean, injecting a list of Person
objects into it.
4. Access the Target Bean:
Now, you can access the GreetingService
bean, which has the collection of Person
objects injected into it:
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Main {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
GreetingService greetingService = context.getBean(GreetingService.class);
greetingService.greetAll();
}
}
In this example, we retrieve the GreetingService
bean from the Spring container and call its greetAll()
method, which uses the injected collection of Person
objects to generate greetings for each person.
Setter injection with collections allows you to inject multiple dependent objects into other beans, making your Spring application more flexible and adaptable to changing requirements. You can easily configure and manage collections of objects in your Spring configuration file.