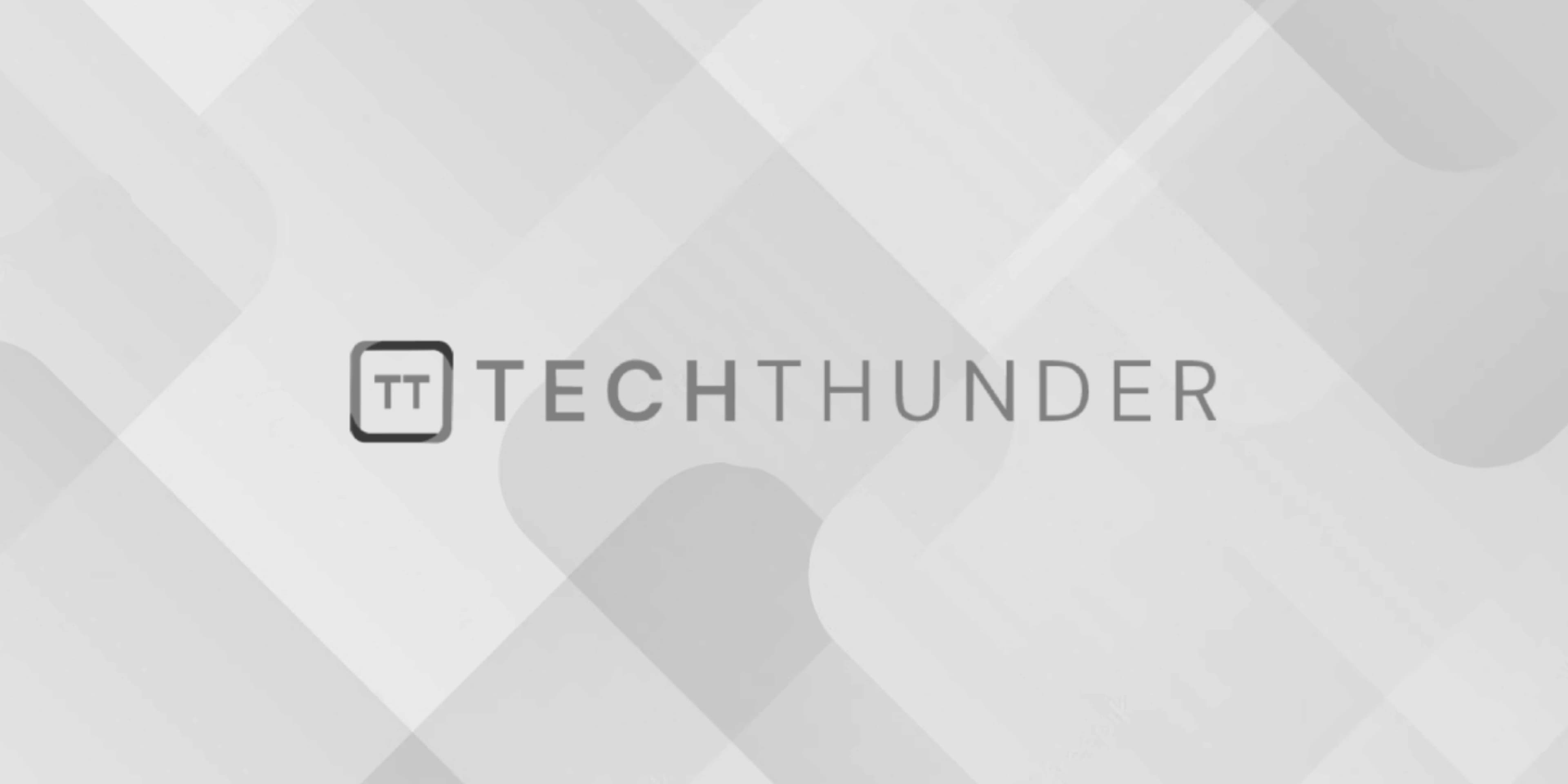
203 views
Spring Form Drop-Down List
The Spring Form Drop-Down List typically refers to creating a dropdown list or select box in a web application developed using the Spring Framework, a popular Java-based framework for building web applications. This dropdown list allows users to select one option from a list of predefined choices.
Here’s a basic outline of how you can create a Spring Form Drop-Down List:
- Create a Model Class: You should have a Java class to represent the data you want to display in the dropdown list. For example, if you want to create a dropdown of countries, you might have a
Country
class with properties likeid
andname
.
public class Country {
private int id;
private String name;
// getters and setters
}
- Create a Controller: In your Spring MVC controller, you would typically prepare a list of options (in this case, a list of countries) and pass it to the view.
@Controller
public class MyController {
@GetMapping("/myPage")
public String myPage(Model model) {
List<Country> countries = // Populate this list with your data
model.addAttribute("countries", countries);
return "myPage";
}
}
- Create a Thymeleaf or JSP View: In your view template (Thymeleaf or JSP), you can use the Spring Form Taglib to render the dropdown list. For Thymeleaf:
<form action="/submitForm" method="post">
<label for="country">Select a country:</label>
<select id="country" name="country">
<option th:each="country : ${countries}" th:value="${country.id}" th:text="${country.name}"></option>
</select>
<input type="submit" value="Submit">
</form>
For JSP:
<form action="/submitForm" method="post">
<label for="country">Select a country:</label>
<select id="country" name="country">
<c:forEach items="${countries}" var="country">
<option value="${country.id}">${country.name}</option>
</c:forEach>
</select>
<input type="submit" value="Submit">
</form>
- Handling Form Submission: Once the user selects an option and submits the form, you can handle the form submission in your controller to process the selected value.
@PostMapping("/submitForm")
public String submitForm(@RequestParam("country") int selectedCountryId) {
// Handle the selected country ID here
return "redirect:/successPage";
}
That’s a basic overview of how to create a Spring Form Drop-Down List. You would need to adjust the code to fit your specific use case, including populating the list of countries with actual data and handling the selected value as needed for your application.