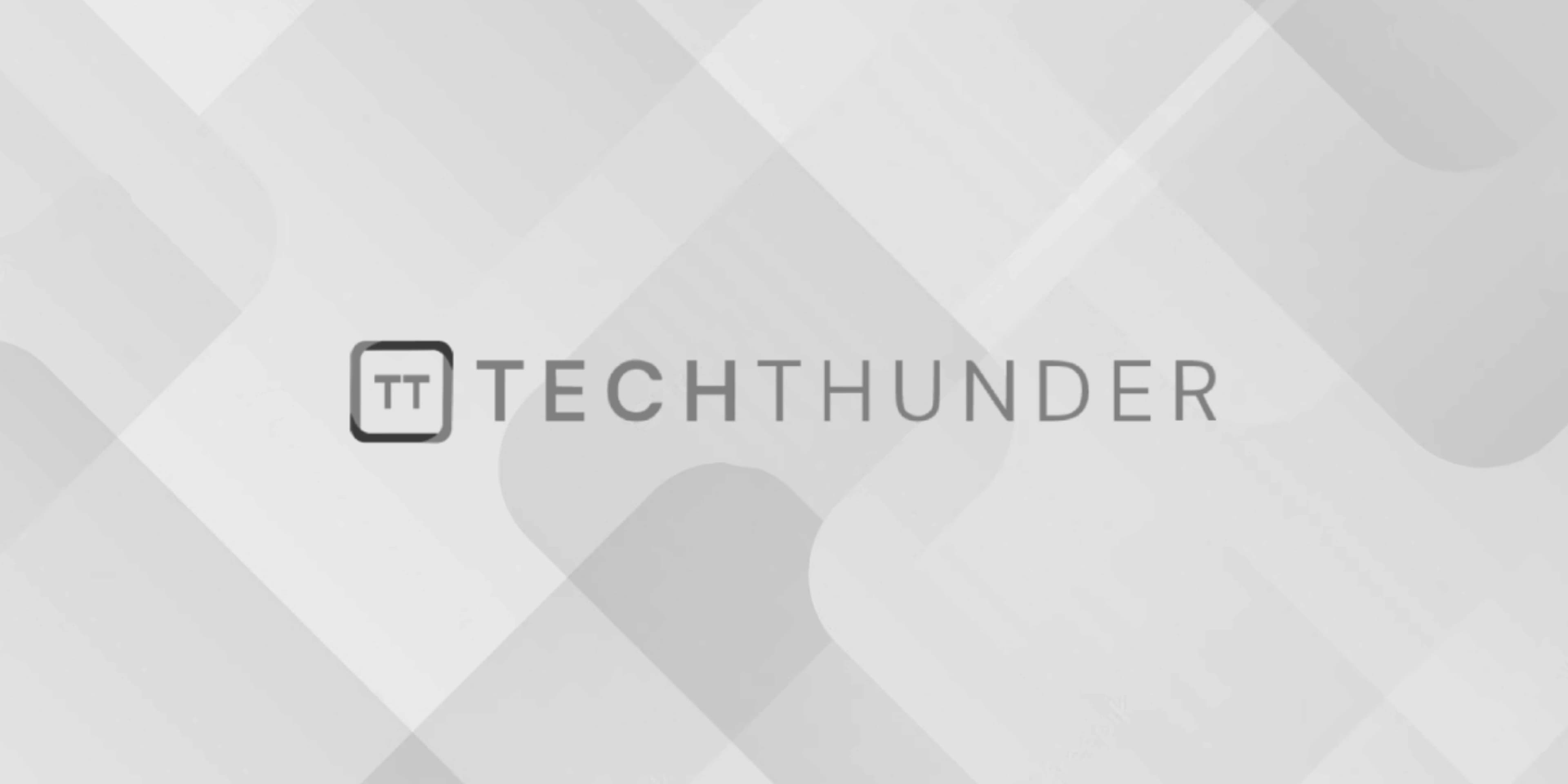
Spring MVC Pagination Example
Implementing pagination in a Spring MVC application allows you to split a large dataset into smaller, manageable chunks that can be displayed on different pages. In this example, I’ll show you how to implement pagination using Spring Boot and Thymeleaf for the view layer.
1. Set Up Your Project:
Create a Spring Boot project with the necessary dependencies. Ensure you have Spring Web, Spring Data JPA, and a database dependency like H2 or MySQL.
2. Create an Entity Class:
Create a JPA entity class that represents the data you want to paginate. For this example, let’s assume you have a Product
entity.
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private double price;
// Getters and setters
}
3. Create a Repository Interface:
Create a repository interface for your entity by extending JpaRepository
(or another repository interface provided by Spring Data JPA).
public interface ProductRepository extends JpaRepository<Product, Long> {
}
4. Create a Controller:
Create a controller to handle pagination. You will need to define methods for retrieving paginated data.
@Controller
@RequestMapping("/products")
public class ProductController {
@Autowired
private ProductRepository productRepository;
@GetMapping
public String listProducts(Model model, @RequestParam(defaultValue = "0") int page) {
int pageSize = 5; // Number of items per page
Page<Product> productPage = productRepository.findAll(PageRequest.of(page, pageSize));
model.addAttribute("productPage", productPage);
return "product/list";
}
}
5. Create a Thymeleaf Template:
Create a Thymeleaf template for displaying the paginated data. Here’s a simple example:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Product List</title>
</head>
<body>
<h1>Product List</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Price</th>
</tr>
</thead>
<tbody>
<tr th:each="product : ${productPage.content}">
<td th:text="${product.id}"></td>
<td th:text="${product.name}"></td>
<td th:text="${product.price}"></td>
</tr>
</tbody>
</table>
<div th:if="${productPage.totalPages > 1}">
<ul class="pagination">
<li th:if="${!productPage.first}" class="page-item">
<a class="page-link" th:href="@{'/products?page=' + (productPage.number - 1)}">Previous</a>
</li>
<li th:each="i : ${#numbers.sequence(0, productPage.totalPages - 1)}" class="page-item">
<a class="page-link" th:href="@{'/products?page=' + i}" th:text="${i + 1}"></a>
</li>
<li th:if="${!productPage.last}" class="page-item">
<a class="page-link" th:href="@{'/products?page=' + (productPage.number + 1)}">Next</a>
</li>
</ul>
</div>
</body>
</html>
This Thymeleaf template displays a paginated list of products and provides Previous and Next buttons to navigate between pages.
6. Configure Database:
Configure the database connection in your application.properties
or application.yml
file.
7. Run Your Application:
Run your Spring Boot application and access the paginated product list at /products
. You should see a list of products with pagination controls.
That’s it! You’ve created a simple Spring MVC pagination example using Spring Boot and Thymeleaf. You can customize the page size, styling, and other aspects to fit your application’s needs.