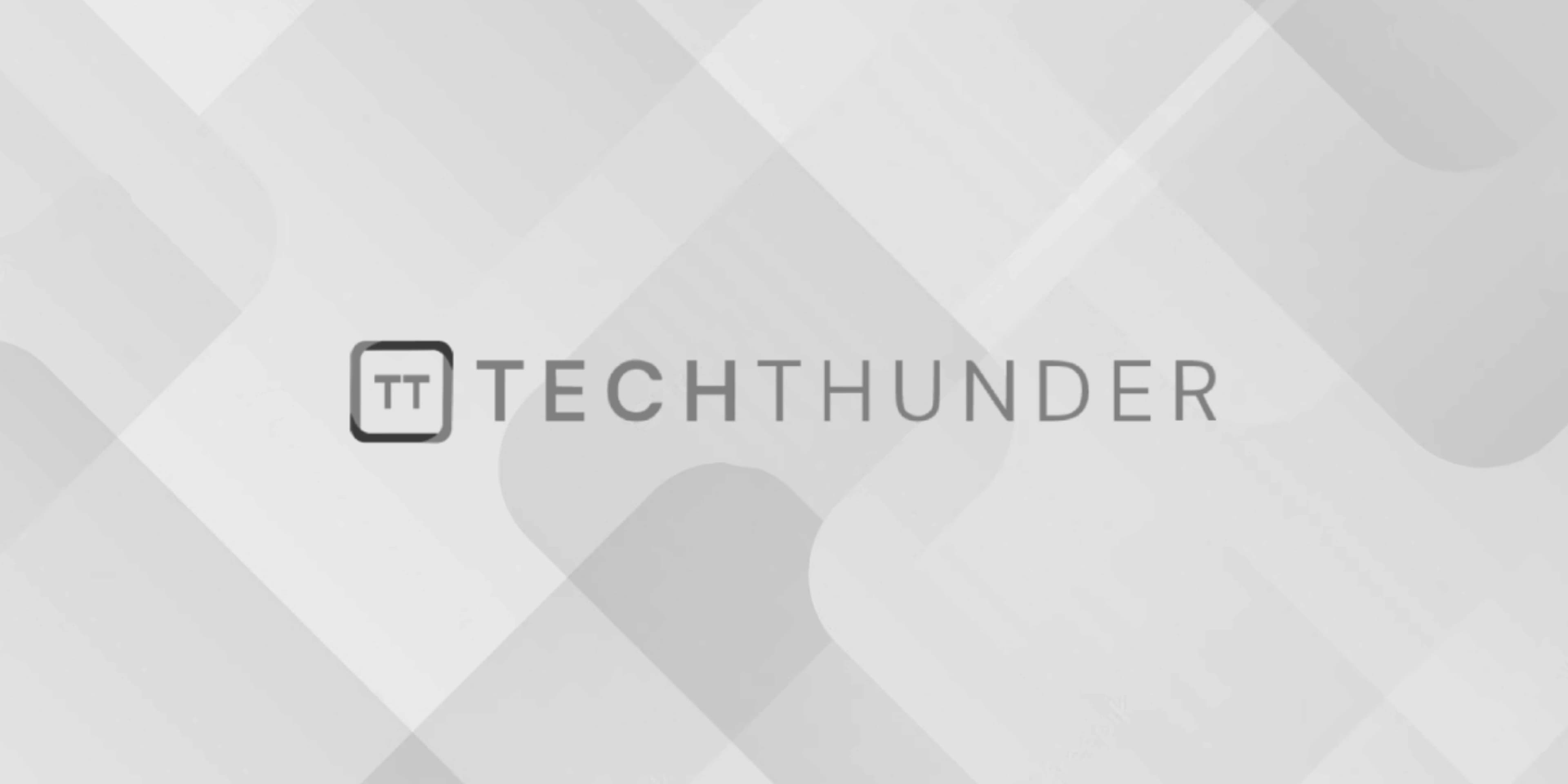
Spring with ORM
Spring provides robust support for integrating with various Object-Relational Mapping (ORM) frameworks, with Hibernate and JPA (Java Persistence API) being among the most commonly used ones. ORM frameworks enable you to work with databases in an object-oriented manner, allowing you to map Java objects to database tables and perform database operations using Java objects rather than writing raw SQL queries. Here’s how you can use Spring with ORM, specifically focusing on Hibernate and JPA:
1. Configure the DataSource:
First, you need to configure a DataSource
bean in your Spring configuration. The DataSource
provides the database connection information.
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/mydatabase" />
<property name="username" value="username" />
<property name="password" value="password" />
</bean>
2. Set Up the Hibernate SessionFactory (For Hibernate):
If you’re using Hibernate, you’ll need to configure a SessionFactory
bean. The SessionFactory
is responsible for creating Hibernate Session
objects.
<bean id="sessionFactory" class="org.springframework.orm.hibernate5.LocalSessionFactoryBean">
<property name="dataSource" ref="dataSource" />
<property name="packagesToScan" value="com.example.model" />
<property name="hibernateProperties">
<props>
<prop key="hibernate.dialect">org.hibernate.dialect.MySQLDialect</prop>
<prop key="hibernate.show_sql">true</prop>
</props>
</property>
</bean>
3. Set Up JPA EntityManagerFactory (For JPA):
If you’re using JPA, configure an EntityManagerFactory
bean. It’s responsible for creating EntityManager
instances.
<bean id="entityManagerFactory" class="org.springframework.orm.jpa.LocalEntityManagerFactoryBean">
<property name="persistenceUnitName" value="myPersistenceUnit" />
</bean>
4. Enable Transaction Management:
Configure transaction management for your application. You can use Spring’s declarative transaction management by using @EnableTransactionManagement
in your configuration class or XML configuration.
@Configuration
@EnableTransactionManagement
public class AppConfig {
// Other configuration settings
}
5. Define Your Model (Entities):
Create Java classes to represent your domain model (entities). These classes should be annotated with Hibernate or JPA annotations to specify the mapping between the objects and the database tables.
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String email;
// Getters and setters
}
6. Create DAOs (Data Access Objects):
Implement DAO classes that interact with the database using Hibernate or JPA. These classes should use the SessionFactory
or EntityManager
to perform database operations.
7. Use Spring’s @Repository
Annotation (Optional):
You can annotate your DAO classes with @Repository
to enable Spring’s exception translation for easier exception handling. This is optional but recommended.
@Repository
public class UserDao {
@Autowired
private SessionFactory sessionFactory;
// DAO methods
}
8. Create a Service Layer (Optional):
You can create a service layer that encapsulates business logic and transaction management. The service layer can use the DAOs to interact with the database.
9. Configure Transactional Behavior (For Transaction Management):
Use the @Transactional
annotation on service methods to specify transactional behavior. This ensures that database operations are performed within a transaction.
@Service
public class UserService {
@Autowired
private UserDao userDao;
@Transactional
public User getUserById(Long id) {
return userDao.findById(id);
}
// Other service methods
}
10. Create Spring Configuration and Main Application:
Define a Spring configuration class and set up the application context. Create an instance of the Spring ApplicationContext
to bootstrap your application.
11. Run Your Spring Application:
Run your Spring application, and it will now use Hibernate or JPA for ORM operations.
These are the fundamental steps to use Spring with ORM, whether you choose Hibernate or JPA as your ORM framework. You can choose the one that best fits your project requirements and preferences.