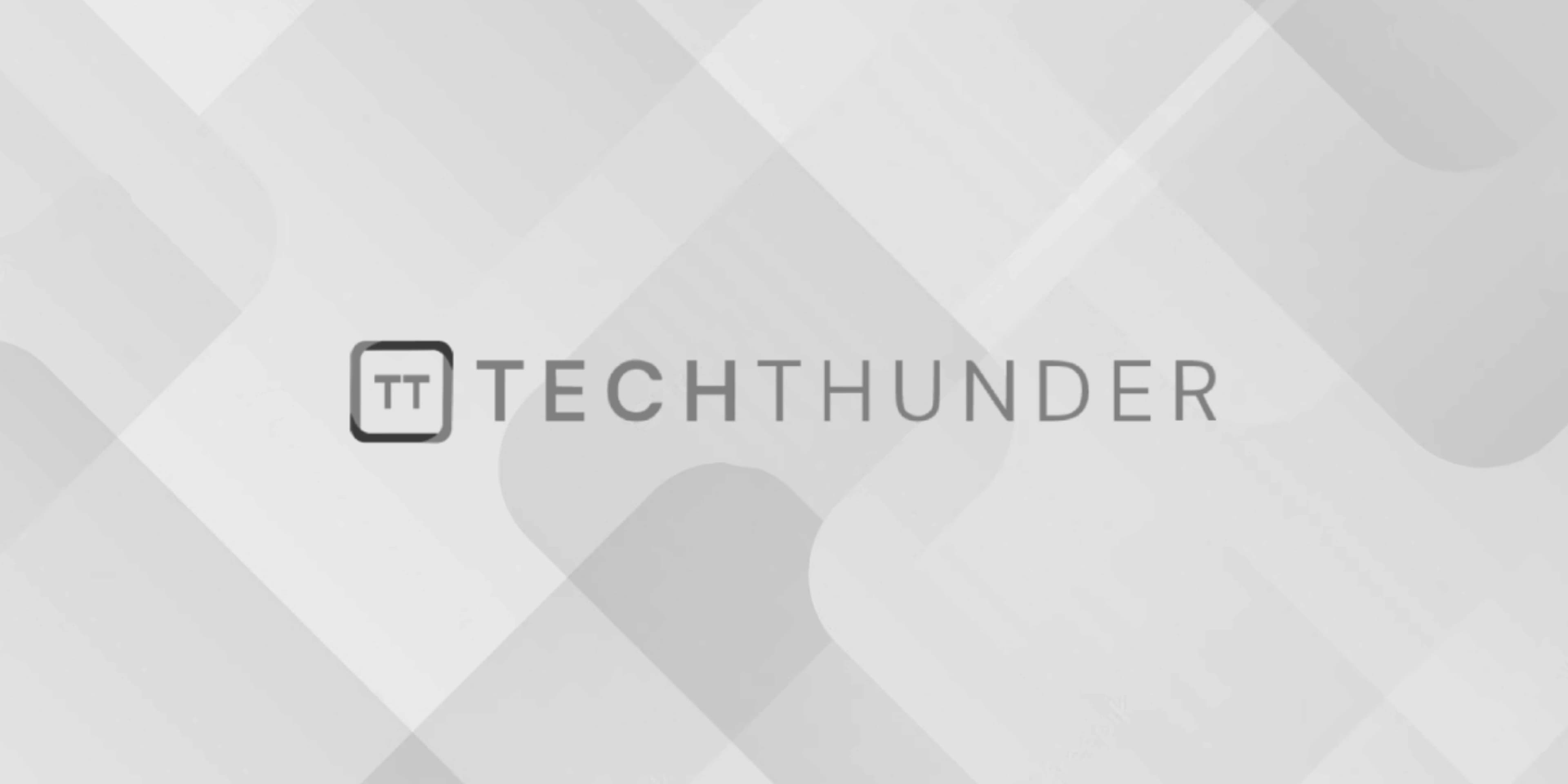
Spring Security at Method Level
Spring Security is a powerful framework that provides various features for securing your Spring-based applications. One of its capabilities is securing methods at the method level using annotations or configuration. This allows you to control access to specific methods based on roles, authorities, or other conditions.
To secure methods using Spring Security at the method level, follow these steps:
- Add Spring Security Dependencies:
Make sure you have the necessary Spring Security dependencies in your project’s build file (such as Maven or Gradle). - Configure Security Configuration:
Create a class that extendsorg.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter
and override itsconfigure(HttpSecurity http)
method. This method is used to define security rules for your application.
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.config.annotation.method.configuration.EnableGlobalMethodSecurity;
@Configuration
@EnableWebSecurity
@EnableGlobalMethodSecurity(securedEnabled = true) // Enable method level security
public class SecurityConfig extends WebSecurityConfigurerAdapter {
// ... Security configuration code ...
}
- Annotate Methods with Security Annotations:
You can use annotations such as@Secured
,@RolesAllowed
,@PreAuthorize
, and@PostAuthorize
to secure methods at the method level.
@Secured
:
Restricts access to a method based on a list of roles.@RolesAllowed
:
Similar to@Secured
, restricts access to a method based on a list of roles.@PreAuthorize
:
Allows you to specify an expression that must evaluate to true before the method is executed.@PostAuthorize
:
Similar to@PreAuthorize
, but the expression is evaluated after the method is executed.
import org.springframework.security.access.annotation.Secured;
import org.springframework.security.access.prepost.PreAuthorize;
import org.springframework.security.access.prepost.PostAuthorize;
public class MyService {
@Secured("ROLE_ADMIN")
public void adminMethod() {
// Method code accessible only to users with ROLE_ADMIN
}
@PreAuthorize("hasRole('USER') and #username == authentication.principal.username")
public void userMethod(String username) {
// Method code accessible to users with ROLE_USER and specific username
}
@PostAuthorize("returnObject.owner == authentication.principal.username")
public MyObject getObject(String id) {
// Method code to retrieve an object, accessible if the owner matches the current user
}
}
Remember that you need to configure your authentication and authorization mechanisms correctly for Spring Security to work as expected. This includes defining user roles, setting up authentication providers, and configuring access control rules.
Keep in mind that Spring Security’s method-level security can become quite flexible and powerful when combined with SpEL (Spring Expression Language) for defining expressions.