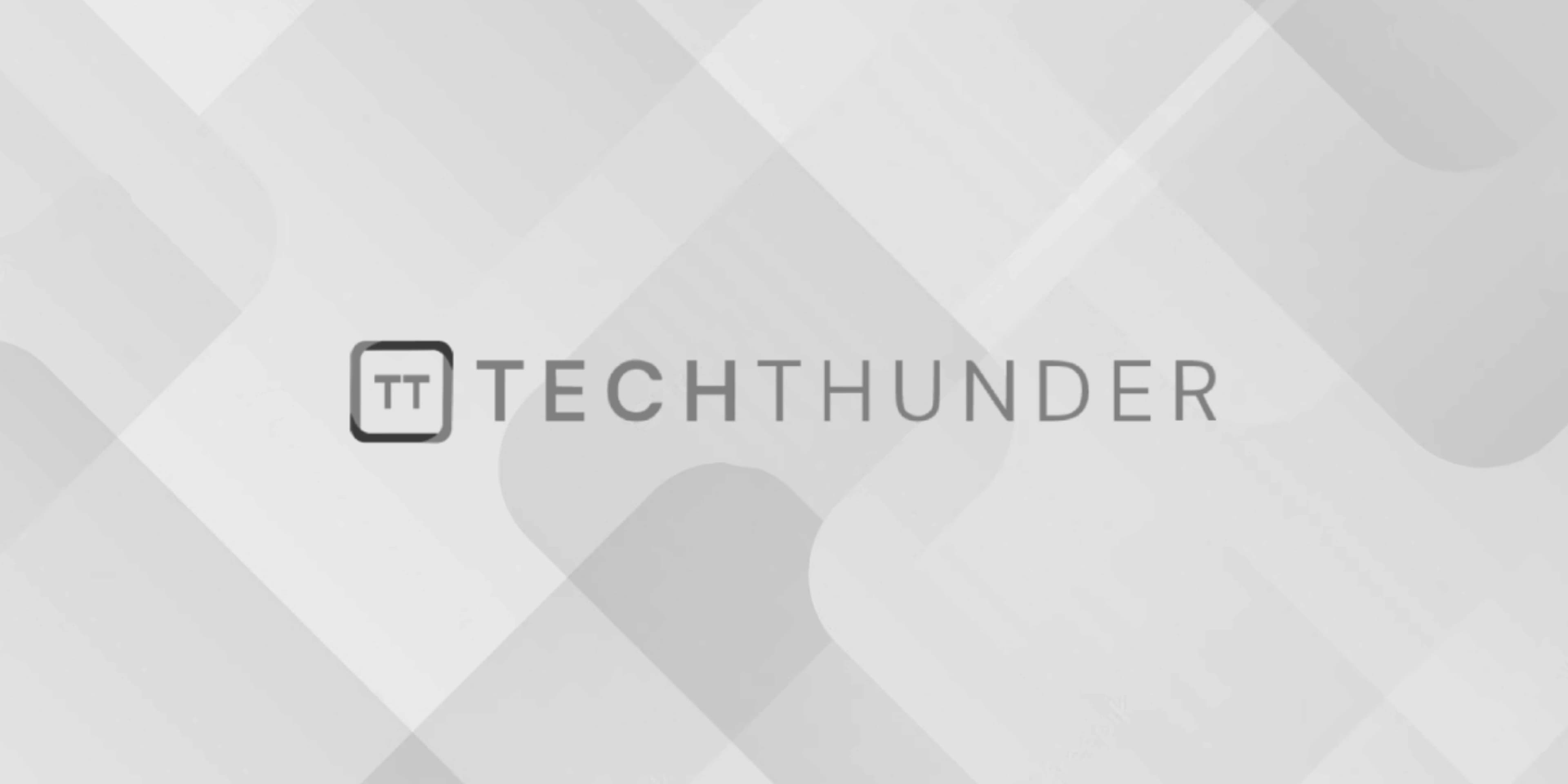
Spring with Hibernate
Integrating Spring with Hibernate is a common practice for building Java web applications that need to interact with relational databases. Hibernate is an Object-Relational Mapping (ORM) framework that allows you to work with databases in an object-oriented way, and Spring provides the infrastructure to manage and configure your application.
Here’s a step-by-step guide on how to integrate Spring with Hibernate:
1. Set Up Your Development Environment:
Make sure you have the necessary development tools and libraries installed, including Java, Spring, Hibernate, and your database (e.g., MySQL, PostgreSQL, or H2).
2. Configure DataSource:
Define a DataSource bean in your Spring configuration file (e.g., applicationContext.xml
). This bean provides the database connection information.
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.cj.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/mydatabase" />
<property name="username" value="your_username" />
<property name="password" value="your_password" />
</bean>
3. Configure Hibernate SessionFactory:
Define a SessionFactory bean that configures Hibernate. The SessionFactory is responsible for creating Hibernate Session objects.
<bean id="sessionFactory" class="org.springframework.orm.hibernate5.LocalSessionFactoryBean">
<property name="dataSource" ref="dataSource" />
<property name="packagesToScan" value="com.example.model" />
<property name="hibernateProperties">
<props>
<prop key="hibernate.dialect">org.hibernate.dialect.MySQL5Dialect</prop>
<prop key="hibernate.show_sql">true</prop>
<!-- Other Hibernate properties go here -->
</props>
</property>
</bean>
Make sure to replace "com.example.model"
with the package where your Hibernate entity classes are located.
4. Create Hibernate Entity Classes:
Create Java classes that represent your database tables and annotate them with Hibernate annotations, such as @Entity
, @Table
, @Id
, @GeneratedValue
, and others.
@Entity
@Table(name = "user")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String email;
// Getters and setters
}
5. Create DAO (Data Access Object) Classes:
Implement DAO classes that use Hibernate to perform database operations. These classes typically include methods for creating, reading, updating, and deleting records.
@Repository
public class UserDao {
@Autowired
private SessionFactory sessionFactory;
public User getUserById(Long id) {
return sessionFactory.getCurrentSession().get(User.class, id);
}
// Other DAO methods
}
6. Configure Spring’s Transaction Management:
Enable Spring’s transaction management by adding @EnableTransactionManagement
to your Spring configuration class or XML configuration file.
@Configuration
@EnableTransactionManagement
@ComponentScan(basePackages = "com.example")
public class AppConfig {
// Other configuration settings
}
7. Use @Transactional Annotation:
Use the @Transactional
annotation on service methods to specify transaction boundaries. This ensures that database operations are performed within a transaction.
@Service
public class UserService {
@Autowired
private UserDao userDao;
@Transactional
public User getUserById(Long id) {
return userDao.getUserById(id);
}
// Other service methods
}
8. Create Spring Configuration and Main Application:
Define a Spring configuration class and set up the application context. Create an instance of the Spring ApplicationContext
to bootstrap your application.
9. Run Your Spring Application:
Run your Spring application, and it will now use Hibernate for ORM operations.
With these steps, you have successfully integrated Spring with Hibernate. Your Spring application can now interact with a relational database using Hibernate for object-relational mapping.