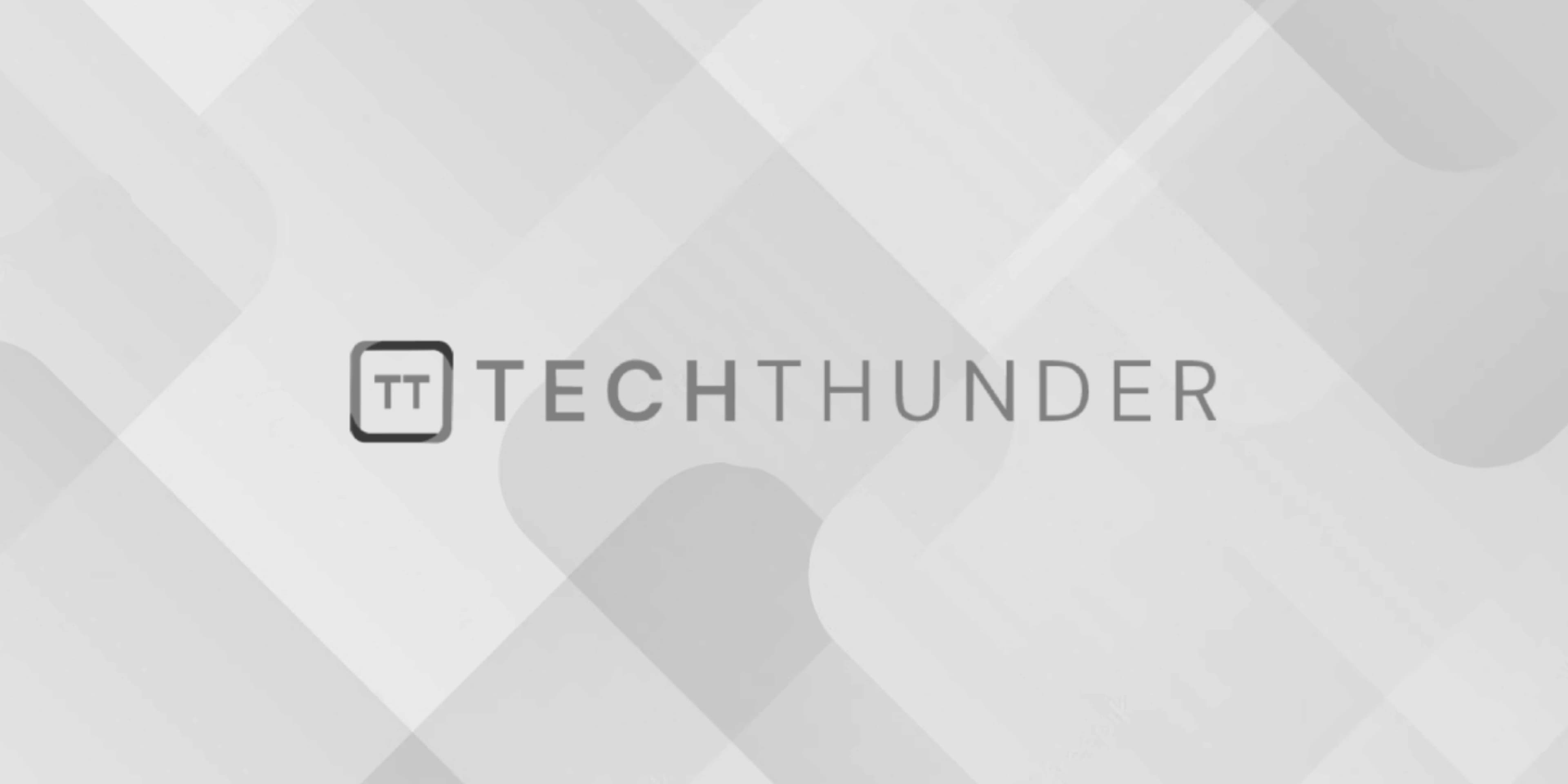
Spring MVC Introduction
Spring MVC (Model-View-Controller) is a web application framework provided by the Spring Framework for building dynamic web applications. It follows the Model-View-Controller architectural pattern, which separates the concerns of an application into three main components: Model, View, and Controller. Spring MVC is a popular choice for developing web applications due to its modularity, flexibility, and extensive features. Here’s an introduction to Spring MVC:
1. Model:
- The Model represents the application’s business logic and data.
- It encapsulates the data and the rules for processing and manipulating that data.
- In Spring MVC, the Model typically consists of Java objects (beans) that hold application data.
2. View:
- The View is responsible for rendering the user interface (UI) and presenting the data to the user.
- It receives data from the Model and displays it to the user.
- Views in Spring MVC are often created using technologies like JSP (JavaServer Pages), Thymeleaf, or other templating engines.
3. Controller:
- The Controller is the central component that handles user requests, processes them, and decides which View to render.
- It acts as an intermediary between the user interface (typically a web browser) and the Model.
- Controllers in Spring MVC are implemented as Java classes and are responsible for request mapping, data processing, and invoking appropriate business logic in the Model.
Key Features of Spring MVC:
- Request Mapping: Spring MVC provides powerful mechanisms for mapping incoming HTTP requests to Controller methods based on URLs, HTTP methods, and request parameters.
- Handler Methods: Controllers in Spring MVC are Java classes containing methods (handler methods) that are responsible for processing specific types of requests.
- Data Binding: Spring MVC supports automatic data binding between HTTP request parameters and Java objects (form backing objects), simplifying the handling of form submissions.
- View Resolution: Spring MVC allows you to define multiple views and provides flexible mechanisms for resolving which view should be rendered based on the outcome of the request.
- Validation: Spring MVC supports validation of form input data using validation frameworks like Hibernate Validator or custom validation logic.
- Interceptors: Interceptors allow you to perform pre-processing and post-processing tasks for HTTP requests and responses.
- Internationalization and Localization: Spring MVC provides features for building applications that can be easily internationalized and localized for different languages and regions.
- Exception Handling: Spring MVC offers robust exception handling mechanisms, allowing you to define global exception handlers and handle exceptions gracefully.
- RESTful Web Services: Spring MVC can be used to build RESTful web services by returning data as JSON or XML, in addition to rendering HTML views.
- Testing Support: Spring MVC provides excellent support for unit and integration testing using tools like JUnit and Spring’s testing framework.
Typical Workflow in Spring MVC:
- A user makes an HTTP request to the web application.
- The DispatcherServlet, a central component in Spring MVC, intercepts the request.
- The DispatcherServlet identifies the appropriate Controller based on the request mapping.
- The Controller processes the request, interacts with the Model (if needed), and prepares the data.
- The Controller selects the appropriate View.
- The View renders the HTML response or other formats (e.g., JSON) based on the data.
- The HTML response is sent back to the user’s browser.
Overall, Spring MVC is a comprehensive framework for building web applications that follow best practices, promote separation of concerns, and provide a robust foundation for creating scalable and maintainable web applications.