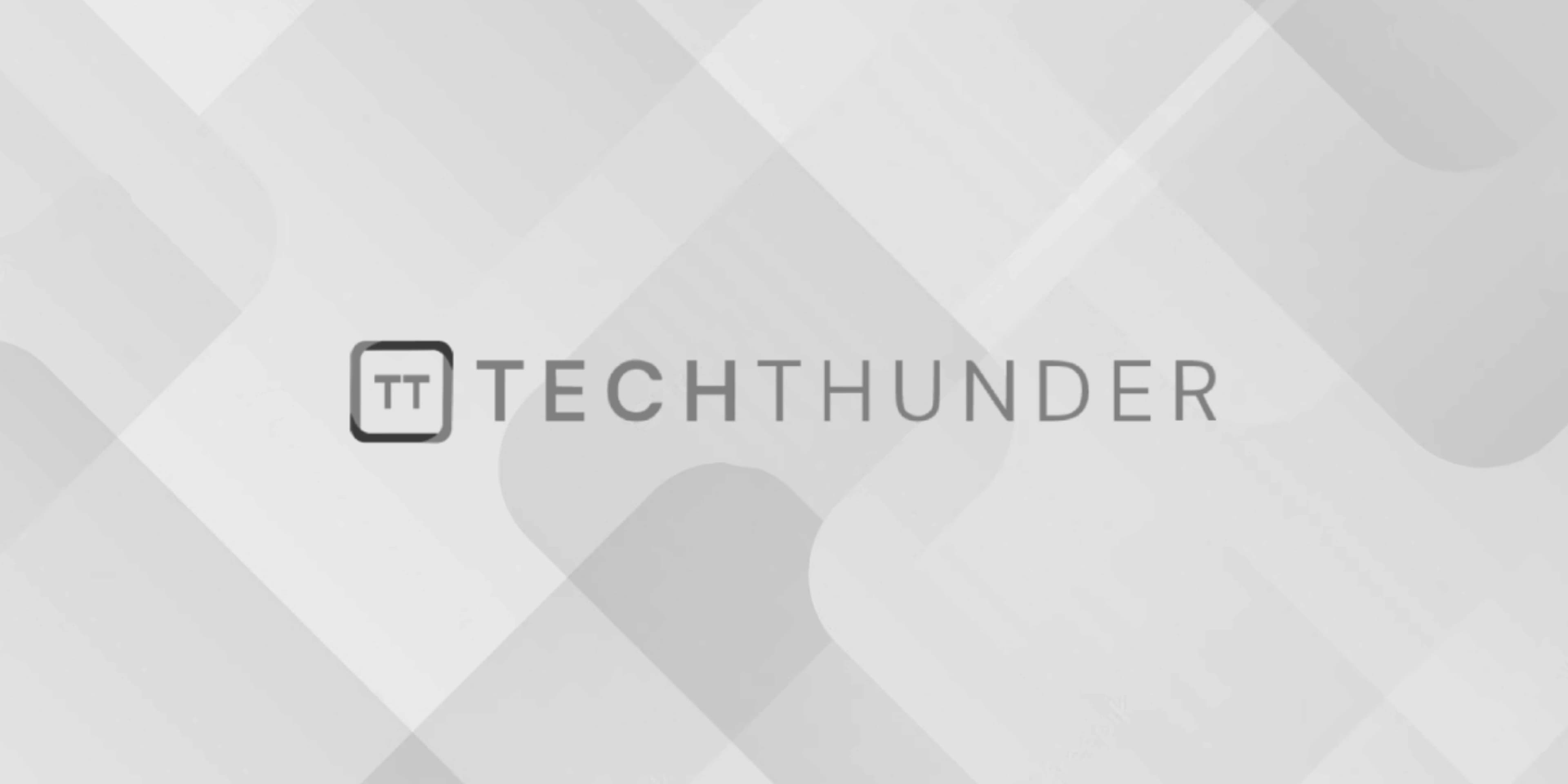
Spring RowMapper
The Spring JDBC, a RowMapper
is an interface used to map rows from a database query result (represented as a ResultSet
) into Java objects. It provides a way to customize the mapping logic for each row of data retrieved from the database. Here’s how you can use a RowMapper
in a Spring application:
1. Define a RowMapper
Implementation:
To use a RowMapper
, you need to create a class that implements the RowMapper<T>
interface, where T
is the type of object you want to map from the ResultSet
rows.
Here’s an example of a RowMapper
implementation for mapping User
objects from a ResultSet
:
import org.springframework.jdbc.core.RowMapper;
import java.sql.ResultSet;
import java.sql.SQLException;
public class UserRowMapper implements RowMapper<User> {
@Override
public User mapRow(ResultSet rs, int rowNum) throws SQLException {
User user = new User();
user.setId(rs.getLong("id"));
user.setUsername(rs.getString("username"));
user.setEmail(rs.getString("email"));
// Populate other fields as needed
return user;
}
}
In this example, the UserRowMapper
class defines the mapping logic for converting a row from the ResultSet
into a User
object.
2. Use the RowMapper
with JdbcTemplate:
To use the RowMapper
, you can pass it as an argument to the query
method of the JdbcTemplate
when executing a query. Here’s an example:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public class UserDao {
private final JdbcTemplate jdbcTemplate;
@Autowired
public UserDao(JdbcTemplate jdbcTemplate) {
this.jdbcTemplate = jdbcTemplate;
}
public List<User> getAllUsers() {
String sql = "SELECT * FROM users";
return jdbcTemplate.query(sql, new UserRowMapper());
}
// Other database operations go here
}
In this example, the UserDao
class uses the UserRowMapper
when querying the database to retrieve a list of all users.
3. Use the RowMapper
in Your Application:
You can then use the UserDao
in your application to retrieve and process the results:
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Main {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
UserDao userDao = context.getBean(UserDao.class);
List<User> users = userDao.getAllUsers();
for (User user : users) {
System.out.println("User ID: " + user.getId());
System.out.println("Username: " + user.getUsername());
System.out.println("Email: " + user.getEmail());
}
}
}
In this example, the getAllUsers
method of the UserDao
returns a list of users using the UserRowMapper
, and you can iterate over the list to process the user data.
Using a RowMapper
is a common practice in Spring JDBC for mapping database query results to Java objects. It provides a clean and reusable way to encapsulate the mapping logic, making your code more maintainable and easier to understand.